DOM Testing Library
Asynchronous Testing with Testing Library
Managing asynchronous operations is an essential part of many modern web applications. In this chapter, we will explore how to write asynchronous tests using DOM Testing Library to ensure that our applications correctly handle operations that are not instantaneous, such as API calls or intentional delays.
Common Asynchronous Operations
The asynchronous operations we usually need to test include:
- API calls.
- Timers (setTimeout, setInterval).
- DOM updates after asynchronous operations.
Creating a Component that Performs an Asynchronous Operation
We are going to create a component that simulates an API call and displays the data once received.
File index.js
:
javascript
Testing an Asynchronous Operation
We are going to write a test for the component that performs an asynchronous operation.
File asyncComponent.test.js
:
javascript
Handling Wait Times with waitFor
In some cases, we may need to explicitly wait for certain changes to appear in the DOM. For this, DOM Testing Library provides waitFor
.
Example using waitFor
:
javascript
[Placeholder for explanatory image: Diagram illustrating the flow of an asynchronous test with DOM Testing Library, from the initial event to waiting for DOM update]
Common Challenges in Asynchronous Tests
-
Awkward Timing Flows:
- Solution: Use
waitFor
to appropriately handle timers and delayed logic.
- Solution: Use
-
Fragile Tests Due to Imprecise Timings:
- Solution: Mock timing operations (like
setTimeout
andsetInterval
) during tests for precise control.
- Solution: Mock timing operations (like
Mocking setTimeout
:
javascript
Conclusion
In this chapter, we have explored how to write asynchronous tests using DOM Testing Library. We covered techniques and tools to effectively manage asynchronous operations in our tests, ensuring that our applications respond correctly after these operations.
In the coming chapters, we will explore test organization and structure strategies, as well as test automation with CI/CD.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
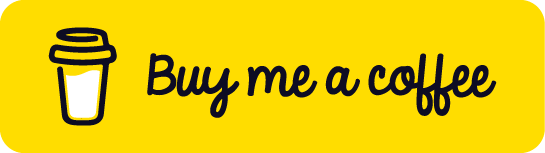
Chat with Chuck
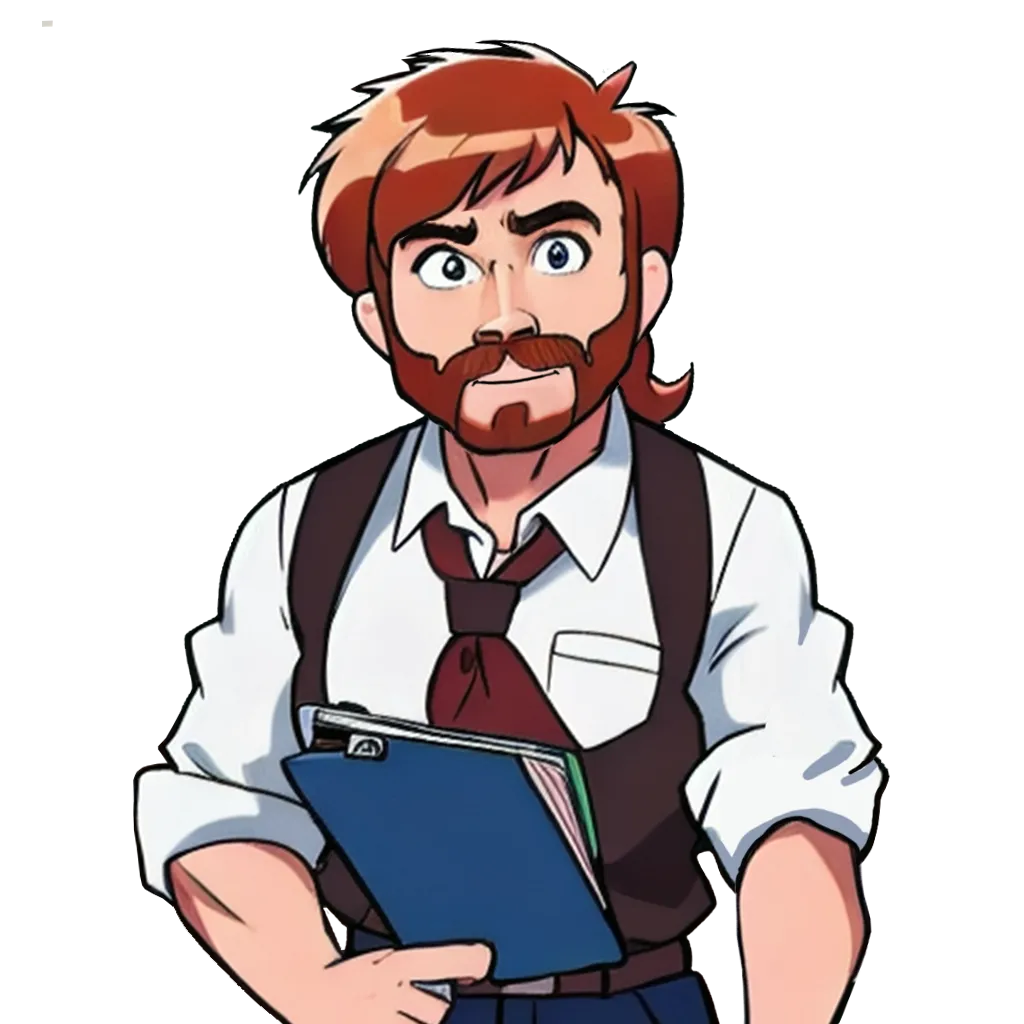
- Introduction to Testing in JavaScript with Testing Library
- DOM Fundamentals
- Installation and Setup of Testing Library
- Writing Your First Unit Tests with Testing Library
- DOM Component Testing with Testing Library
- DOM Event Testing with Testing Library
- Mocking and Stubbing in Testing Library
- User Interaction Testing with Testing Library
- Accessibility Testing with Testing Library
- Asynchronous Testing with Testing Library
- Organization and Structure of Tests in Testing Library
- Test Automation with CI/CD using Testing Library
- Best Practices for Testing with Testing Library
- Debugging Failed Tests in Testing Library
- Conclusions and Next Steps in Testing with Testing Library