DOM Testing Library
DOM Event Testing with Testing Library
In this chapter, we will explore how to test DOM events using DOM Testing Library. Events are a fundamental part of interaction in web applications, and it is crucial to ensure that event handlers work correctly.
Understanding DOM Events
DOM events are actions that occur in the browser, such as clicking a button, moving the mouse, typing in a text field, among others. We can use DOM Testing Library to simulate these events and verify that the event handlers produce the expected behavior.
Click Event Testing
Let's see how to write a test for a click event.
index.js
file:
javascript
button.test.js
file:
javascript
Simulating Other Events
DOM Testing Library supports a wide variety of events. Here are some examples of how to simulate different types of events.
Text Input Event
Modify and test the change in a text input field.
input.test.js
file:
javascript
Form Submit Event
Modify the value of an input field and submit a form.
form.test.js
file:
javascript
[Placeholder for explanatory image: Diagram showing how DOM Testing Library interacts with various DOM events, including clicks, text inputs, and form submissions]
Event Simulation Methods Summary
DOM Testing Library provides several methods to simulate events:
fireEvent.click(element)
: Simulates a click on the element.fireEvent.change(element, { target: { value: '...' } })
: Simulates a change in the value of an input field.fireEvent.input(element, { target: { value: '...' } })
: Simulates text input in an input field.fireEvent.submit(element)
: Simulates form submission.
Conclusion
In this chapter, we learned how to use DOM Testing Library to simulate and test DOM events. Understanding and manipulating events is essential to ensure that your application responds correctly to user interactions. These skills will allow you to write more robust and reliable tests.
In the upcoming chapters, we will explore advanced techniques such as mocking and testing complex user interactions.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
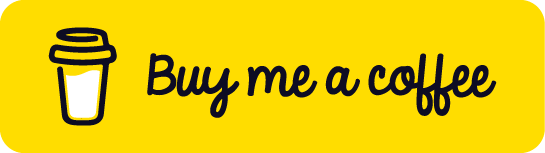
Chat with Chuck
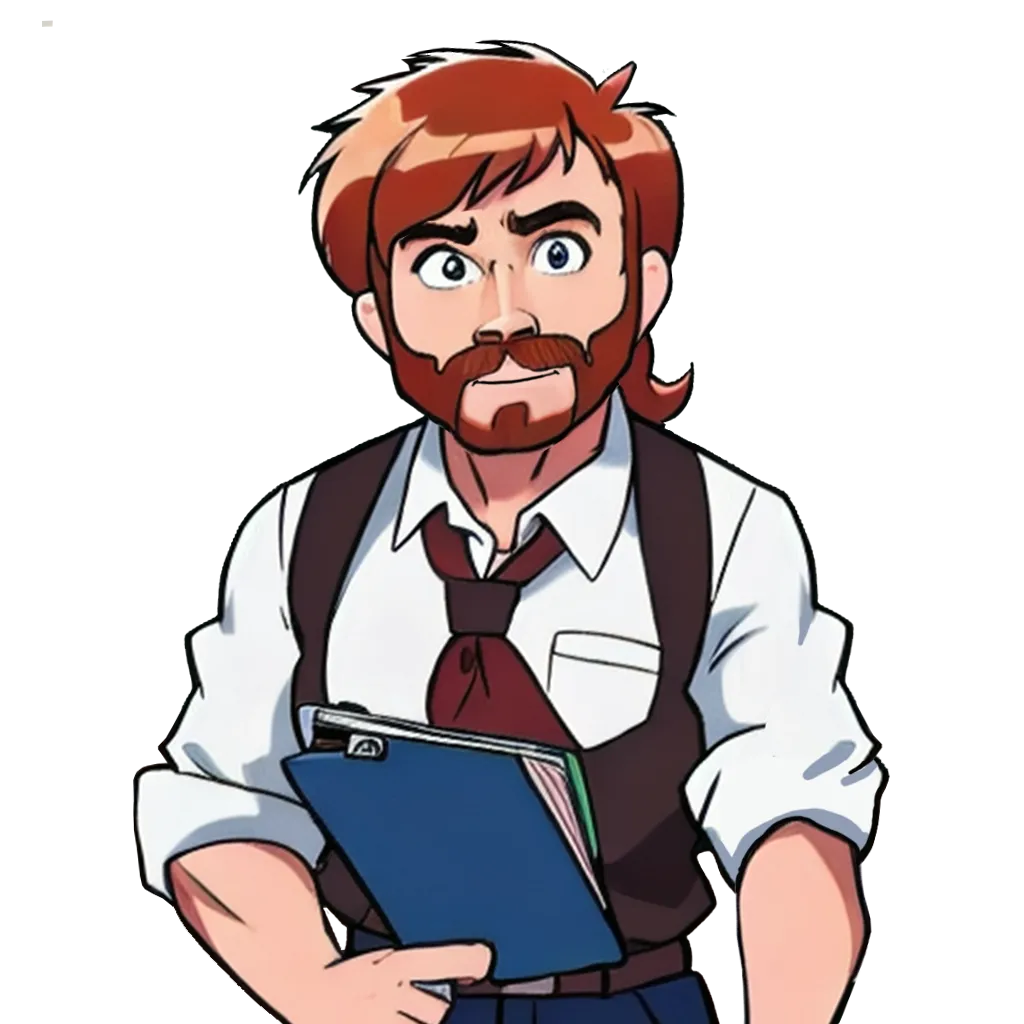
- Introduction to Testing in JavaScript with Testing Library
- DOM Fundamentals
- Installation and Setup of Testing Library
- Writing Your First Unit Tests with Testing Library
- DOM Component Testing with Testing Library
- DOM Event Testing with Testing Library
- Mocking and Stubbing in Testing Library
- User Interaction Testing with Testing Library
- Accessibility Testing with Testing Library
- Asynchronous Testing with Testing Library
- Organization and Structure of Tests in Testing Library
- Test Automation with CI/CD using Testing Library
- Best Practices for Testing with Testing Library
- Debugging Failed Tests in Testing Library
- Conclusions and Next Steps in Testing with Testing Library