DOM Testing Library
User Interaction Testing with Testing Library
User interactions are a crucial aspect of any web application, as they determine how end users interact with the UI. In this chapter, we will learn how to simulate and test complex user interactions using DOM Testing Library.
Common User Interactions
User interactions can include clicks, text inputs, selecting items from dropdown lists, navigation, among others. We will use DOM Testing Library to simulate these interactions and ensure your application responds correctly.
Create an Interactive Component
We will create a dropdown list component that allows the user to select an item and display their choice in the DOM.
index.js
file:
javascript
Testing User Interactions
Let's see how to test user interactions with this component.
dropdown.test.js
file:
javascript
Simulating Multiple Events
Sometimes, user interactions can involve multiple events. Consider a form with validation where users must enter text and, upon making an error, see a warning message.
index.js
file (form validation component):
javascript
Testing Form Validation
Test that simulates user input and form submission, verifying the validation.
validationForm.test.js
file:
javascript
[Placeholder para imagen explicativa: Diagrama que muestra las interacciones del usuario, como la selección de opciones y el envío de formularios, y cómo las pruebas simulan estas acciones]
Summary of User Interaction Simulation Methods
DOM Testing Library provides methods to simulate various user interactions:
fireEvent.change(element, { target: { value: '...' } })
: Simulates a change in the value of an input field or dropdown list.fireEvent.input(element, { target: { value: '...' } })
: Simulates text input in an input field.fireEvent.submit(element)
: Simulates form submission.fireEvent.click(element)
: Simulates a click on an element.
Conclusion
In this chapter, we have learned how to simulate and test user interactions with DOM Testing Library. This skill set is crucial to ensuring that your application responds correctly to user actions, providing a smooth and robust user experience.
In the upcoming chapters, we will explore accessibility testing and asynchronous testing with Testing Library.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
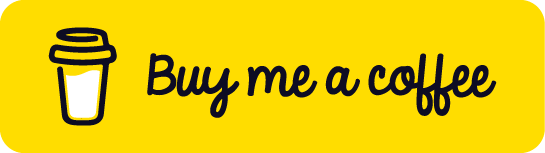
Chat with Chuck
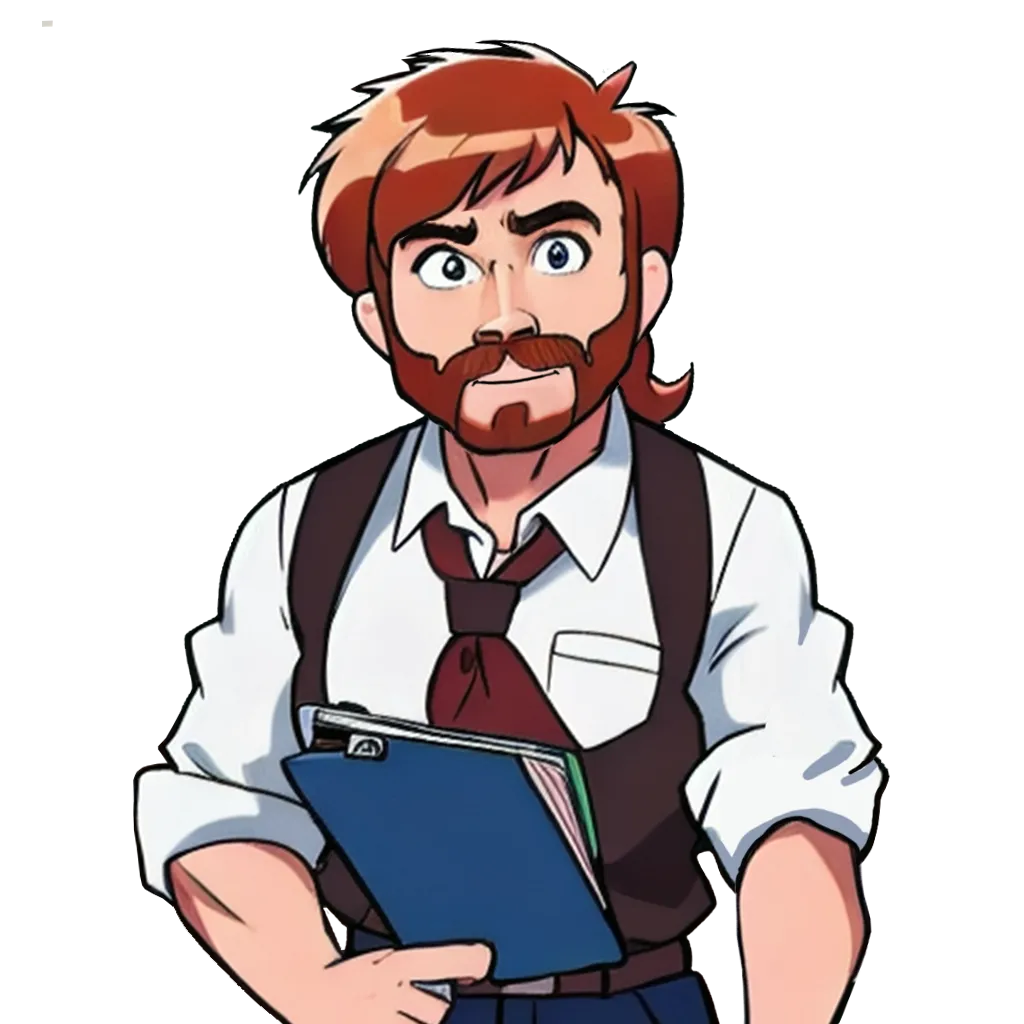
- Introduction to Testing in JavaScript with Testing Library
- DOM Fundamentals
- Installation and Setup of Testing Library
- Writing Your First Unit Tests with Testing Library
- DOM Component Testing with Testing Library
- DOM Event Testing with Testing Library
- Mocking and Stubbing in Testing Library
- User Interaction Testing with Testing Library
- Accessibility Testing with Testing Library
- Asynchronous Testing with Testing Library
- Organization and Structure of Tests in Testing Library
- Test Automation with CI/CD using Testing Library
- Best Practices for Testing with Testing Library
- Debugging Failed Tests in Testing Library
- Conclusions and Next Steps in Testing with Testing Library