DOM Testing Library
DOM Component Testing with Testing Library
Now that we have a basic understanding of how to write unit tests, it’s time to move on to testing more complex DOM components. We will see how to use DOM Testing Library to verify the structure, content, and behavior of components that interact with each other in the DOM.
Create a Complex Component
For this exercise, we will create a simple form component with an input field and a button that, when clicked, displays a message.
File index.html
:
html
File index.js
:
javascript
Writing Tests for the Component
We are going to write several tests that verify different aspects of the component: its initial structure, user input, and the behavior of the form upon submission.
File form.test.js
:
javascript
Understanding the Tests
- Initial Render Test:
getByLabelText
is used to find theinput
by its associated label.getByText
is used to find the button by its text.
- Form Submission Test:
fireEvent.change
simulates user input in the text field.fireEvent.click
simulates a click event on the form’s submit button.- We verify that the message updates correctly after the form submission.
[Placeholder for explanatory image: Diagram showing the flow of user interactions with the form, from input to submission and message update]
Additional Tests
We can write additional tests to cover more scenarios, such as form validation or event handling tests.
Input Field Validation
Suppose we want the form to show an error message if the input field is empty when the form is submitted.
File index.js
(modified):
javascript
File form.test.js
(add test):
javascript
Conclusion
In this chapter, you have learned how to write tests for more complex DOM components using DOM Testing Library. We covered various aspects, from verifying the initial structure to simulating user interactions and validating component behavior.
These techniques will allow you to ensure that your application components work as expected, and prepare you to write more advanced tests involving multiple components and their interactions.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
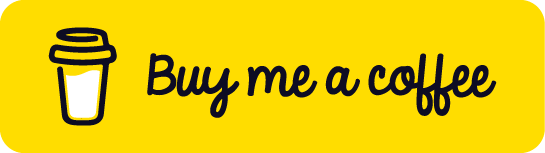
Chat with Chuck
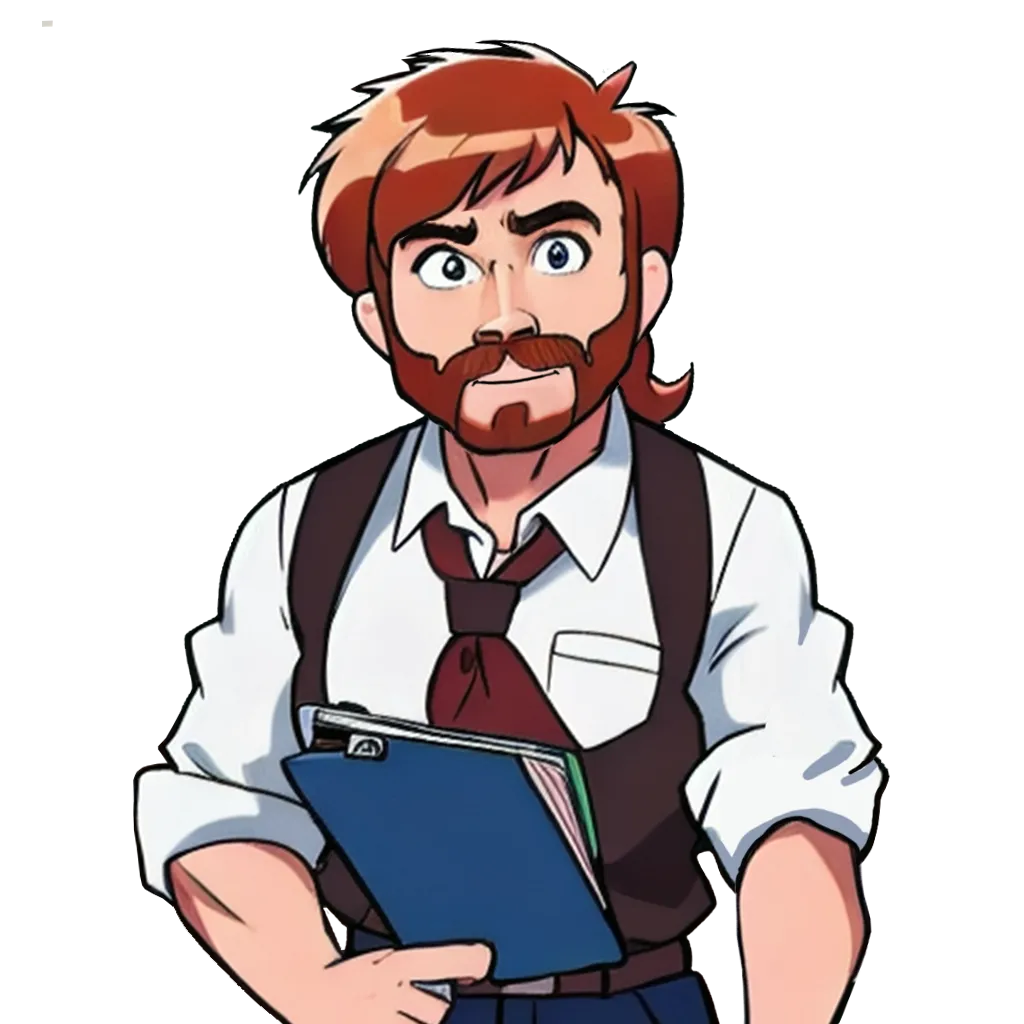
- Introduction to Testing in JavaScript with Testing Library
- DOM Fundamentals
- Installation and Setup of Testing Library
- Writing Your First Unit Tests with Testing Library
- DOM Component Testing with Testing Library
- DOM Event Testing with Testing Library
- Mocking and Stubbing in Testing Library
- User Interaction Testing with Testing Library
- Accessibility Testing with Testing Library
- Asynchronous Testing with Testing Library
- Organization and Structure of Tests in Testing Library
- Test Automation with CI/CD using Testing Library
- Best Practices for Testing with Testing Library
- Debugging Failed Tests in Testing Library
- Conclusions and Next Steps in Testing with Testing Library