Node.js
Alternatives to Express and Fetch
Although Express and Fetch are very popular and widely used tools in the Node.js ecosystem for creating REST APIs and making HTTP requests, there are other alternatives that can be useful depending on your project's needs. In this chapter, we'll explore some of these frameworks and libraries, highlighting their advantages and use cases.
Alternatives to Express
Express is the most popular framework for creating applications and APIs in Node.js, but there are other frameworks that offer different features and approaches.
Koa.js
Koa.js is a framework created by the same developers of Express, but with a minimalist and modular approach. Koa doesn't include middleware by default, which gives you more control over the dependencies you use. Some of the advantages of Koa include:
- Greater modularity: Koa doesn't come with built-in middleware, allowing you to add only what you need.
- Better handling of asynchrony: Koa uses Promises and
async/await
more cleanly than Express, making it easier to manage asynchronous flows.
Basic Server Example with Koa
javascript
Hapi.js
Hapi.js is another popular framework that focuses on configuration over coding. It is known for its robustness in terms of security and validation, making it an excellent choice for enterprise applications.
- Validation: Hapi.js includes an advanced request validation system, reducing the need to depend on external libraries like
Joi
. - Security: Hapi.js has advanced security features that make it easy to build applications resistant to common attacks.
Basic Server Example with Hapi
javascript
Fastify
Fastify is a framework focused on performance. It is promoted as faster than Express and is ideal for applications that require high speed and low latency.
- High performance: Fastify is optimized to handle a large number of requests per second.
- Built-in validation: Similar to Hapi.js, Fastify includes request validation, simplifying data management.
Basic Server Example with Fastify
javascript
Alternatives to Fetch
Fetch
is a modern and native API in browsers that allows making HTTP requests, but in Node.js it is not available by default (unless using recent Node.js versions that include it). Fortunately, there are other options for making HTTP requests from Node.js.
Axios
Axios is a very popular library that offers a simple and clean API for making HTTP requests in Node.js and browsers. Some of its advantages include:
- Support for Promises: Axios uses Promises, making asynchronous handling easier.
- Interceptors: Allows modifying requests or responses before sending or receiving them.
HTTP Request Example with Axios
javascript
Got
Got is another library for making HTTP requests focused on being lightweight and fast. It is an excellent alternative if you need something lighter than Axios or if you prefer an API based on async/await
.
HTTP Request Example with Got
javascript
Request (Deprecated)
The request
library was very popular for many years, but it has become deprecated and no longer receives updates. Although it is still used in legacy projects, it is recommended to migrate to more modern alternatives like Axios or Got.
REST vs GraphQL
While REST remains the most widely used architecture for building APIs, GraphQL has gained popularity in recent years as a more flexible alternative. Let's see some key differences between REST and GraphQL.
REST
In REST, HTTP requests are directed to specific routes (endpoints) and the server responds with complete resources. Each resource has its own URL and operations are limited to standard HTTP methods like GET, POST, PUT, and DELETE.
GraphQL
GraphQL, on the other hand, allows clients to specify exactly what data they need, reducing the amount of data sent between the client and server. Instead of multiple endpoints, GraphQL uses a single URL where clients send queries that define what data they want to receive.
GraphQL Query Example
graphql
When to Use REST or GraphQL?
- Use REST: When you need simplicity and standardization. REST is more suitable for simple applications or when reducing response size is not necessary.
- Use GraphQL: When you need flexibility in data requests or when your application needs to optimize data traffic, especially in mobile or limited connection applications.
Summary
In this chapter, we have explored various alternatives to Express and Fetch in the Node.js ecosystem, including Koa, Hapi, Fastify, Axios, and Got. We have also discussed the difference between REST and GraphQL, providing practical examples of how to use them. The choice of a tool or framework depends on your project's specific needs and the features you seek in terms of performance, modularity, and ease of use.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
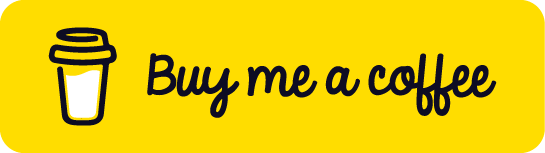
Chat with Chuck
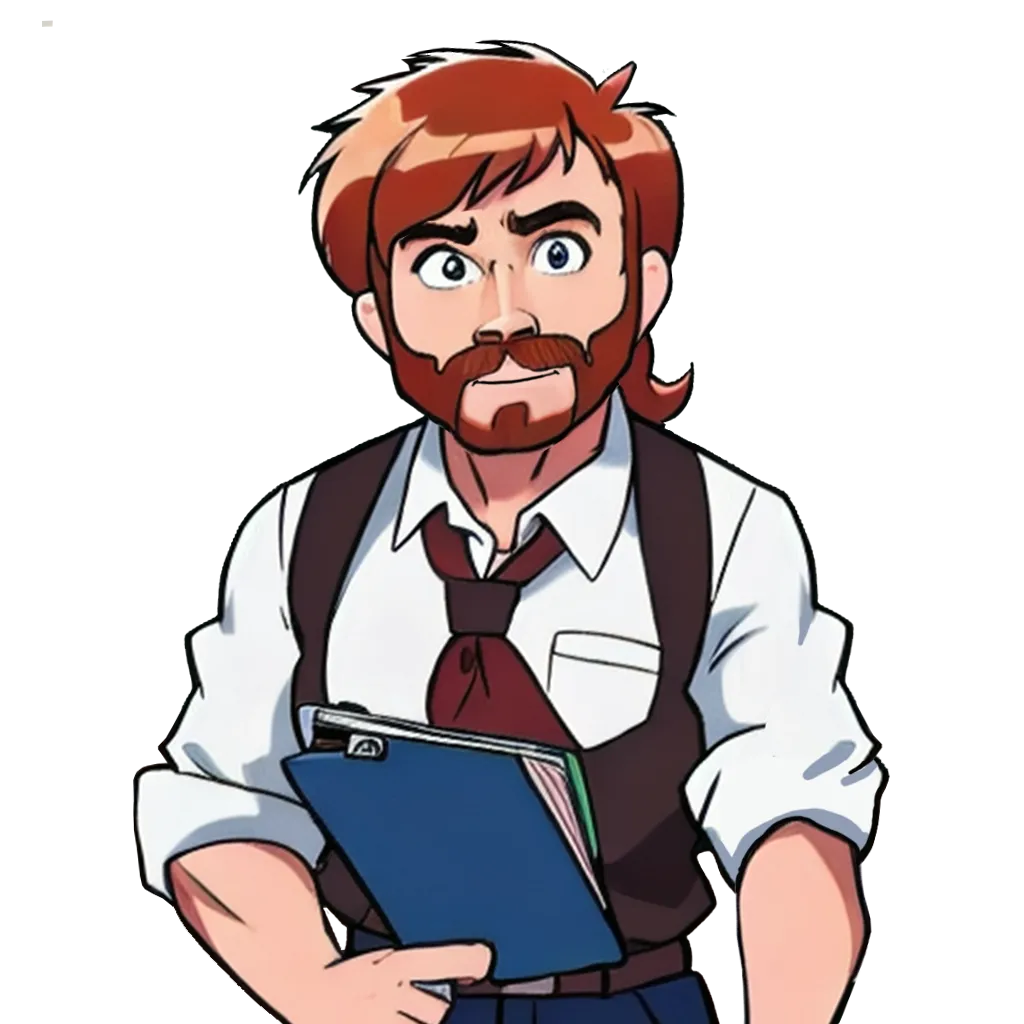
- Introduction to Node.js
- Modules and Packages in Node.js
- Asynchrony in Node.js
- Creating a REST API with Express
- Data Management in Node.js
- Authentication and Authorization
- File Handling and Uploads
- Testing in Node.js
- Security in Node.js Applications
- WebSocket Implementation
- Deployment and Scalability in Node.js
- Monitorización y Mantenimiento
- Alternatives to Express and Fetch
- Conclusions and Best Practices in Node.js