Node.js
Authentication and Authorization
Authentication and authorization are critical components in developing secure backend applications. Authentication refers to verifying a user's identity, while authorization controls user permissions to access specific resources. In this chapter, we will learn how to implement authentication and authorization using JSON Web Tokens (JWT) in Node.js and explore some alternatives.
Introduction to JSON Web Tokens (JWT)
A JSON Web Token (JWT) is an open standard used to transmit information securely between two parties. A JWT consists of three parts: the header, the payload, and the signature, which are encoded in Base64 and separated by dots. JWT tokens are ideal for authentication because they are secure, easy to implement, and do not require the server to maintain user state.
Installing jsonwebtoken
To work with JWT in Node.js, we will use the jsonwebtoken
package. First, let's install the package:
bash
Generating a JWT
Once installed, we can generate a JWT that contains user information. Let's see an example of how to create a JWT token in Node.js:
javascript
Verifying a JWT
Once the client receives the token, it sends it to the server on each request to prove its identity. The server must verify that the token is valid. Here is how to verify a JWT in Node.js:
javascript
Implementing JWT Authentication in Express
Next, we will see how to implement a JWT-based authentication system in a REST API using Express.
Creating an Authentication Route
First, we need to create a login route that generates and returns a JWT to the client when the user logs in successfully. We will assume that the user data is in a database and has been verified before generating the token:
javascript
Route Protection Middleware
To protect specific routes in our API, we can create middleware that verifies the JWT token before allowing access. If the token is not valid, the request will be rejected.
javascript
Role-Based Authorization
In addition to authentication, we can implement authorization, which is based on user roles to restrict access to certain features. For example, only administrators might have access to certain routes.
javascript
Alternatives to JWT: Passport.js and OAuth
While JWT is a popular and efficient solution for API authentication, there are other alternatives that may be useful depending on your application's requirements.
Passport.js
Passport.js
is a flexible authentication middleware that allows different authentication strategies, such as session-based authentication, OAuth, and JWT. It's a robust option if you need a more complex authentication system or want to integrate multiple authentication methods.
OAuth
OAuth
is an authorization protocol mainly used to allow third-party applications to access a user's information in a service, such as Google or Facebook, without sharing credentials. If your application needs to authenticate with external services, OAuth is a strong option.
Summary
In this chapter, we have learned to implement authentication and authorization using JSON Web Tokens in Node.js. We also explored how to protect routes with middleware and how to implement roles to control access. While JWT is a very popular solution, alternatives like Passport.js and OAuth can be useful for applications that require more complex authentication.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
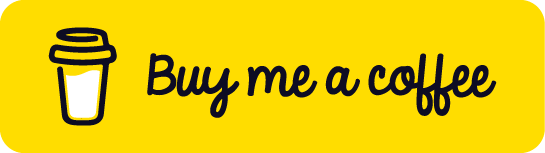
Chat with Chuck
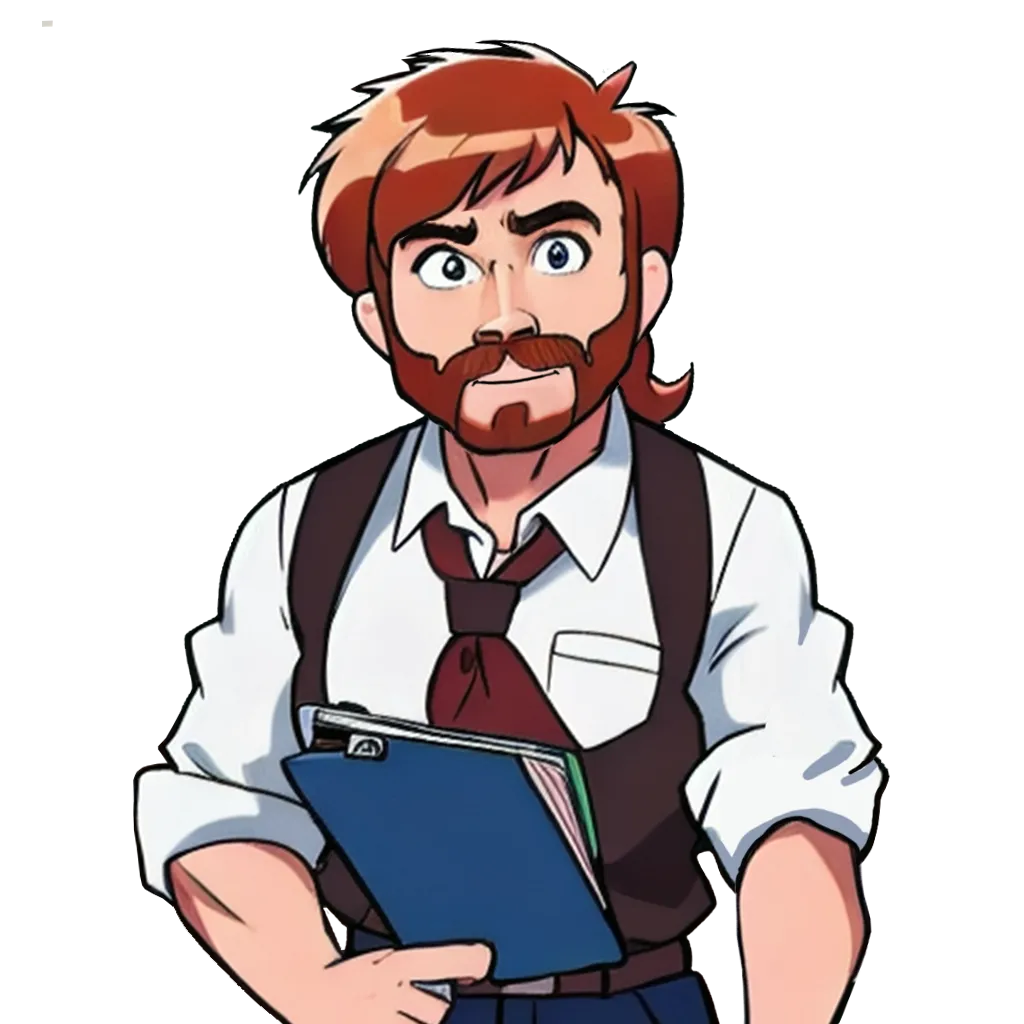
- Introduction to Node.js
- Modules and Packages in Node.js
- Asynchrony in Node.js
- Creating a REST API with Express
- Data Management in Node.js
- Authentication and Authorization
- File Handling and Uploads
- Testing in Node.js
- Security in Node.js Applications
- WebSocket Implementation
- Deployment and Scalability in Node.js
- Monitorización y Mantenimiento
- Alternatives to Express and Fetch
- Conclusions and Best Practices in Node.js