Node.js
Testing in Node.js
Testing is a crucial part of application development because it ensures that the code works as expected and that changes do not introduce errors. In Node.js, there are several tools that allow us to perform unit, integration, and acceptance tests. In this chapter, we will learn to write tests using Mocha and Chai, and how to test our APIs with Supertest.
Introduction to Testing in Node.js
In Node.js, application testing can be divided into several categories:
- Unit Tests: Verify if an individual unit of code (e.g., a function) works correctly.
- Integration Tests: Test how different modules or components interact within an application.
- Acceptance Tests: Validate that the application works as required, from the user's perspective.
Installing Mocha and Chai
Mocha is a popular testing framework that provides a flexible way to define and run tests. Chai is an assertion library that works well with Mocha. Let's install them:
bash
Creating a Unit Test with Mocha and Chai
Let's create a simple unit test for a function that adds two numbers. Create a file sum.js
with the following content:
javascript
Now, create a test file called test/sum.test.js
and define a test for this function using Mocha and Chai:
javascript
Running the Tests
To run the tests, we can add a script in our package.json
and then run Mocha from the terminal:
json
Then, run the following command:
bash
Testing APIs with Supertest
Supertest is a tool that allows us to perform integration tests of our APIs in Node.js. Let's see how we can test a basic API using Express.
Installing Supertest
First, let's install Supertest as a development dependency:
bash
Creating an API to Test
Imagine we have a basic API that returns a list of users. Here's the code for app.js
:
javascript
Creating an API Test with Supertest
Now, let's write a test to verify that the API returns the user list correctly. Create a test file called test/app.test.js
:
javascript
Code Coverage Tools
To ensure that all parts of our code are being tested, we can use a code coverage tool like nyc
. Install nyc
with the following command:
bash
Then, add the following script in your package.json
to run the tests with coverage:
json
Run the tests with:
bash
Best Testing Practices
When testing Node.js applications, it is important to follow some best practices:
- Small and Modular Tests: Tests should focus on a single unit of code to facilitate debugging.
- Test Isolation: Tests should not depend on each other; they should be independent and reproducible in any environment.
- Automation: Integrate tests into your CI/CD flow so they run automatically whenever code changes are made.
Summary
In this chapter, we have learned to write unit and integration tests in Node.js using Mocha, Chai, and Supertest. We have also explored how to measure code coverage with nyc. Testing is a vital part of developing robust applications as it ensures that our code works correctly before moving to production.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
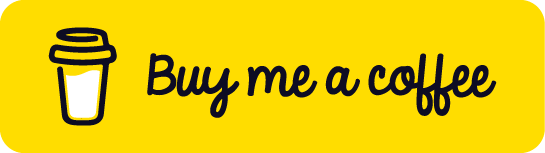
Chat with Chuck
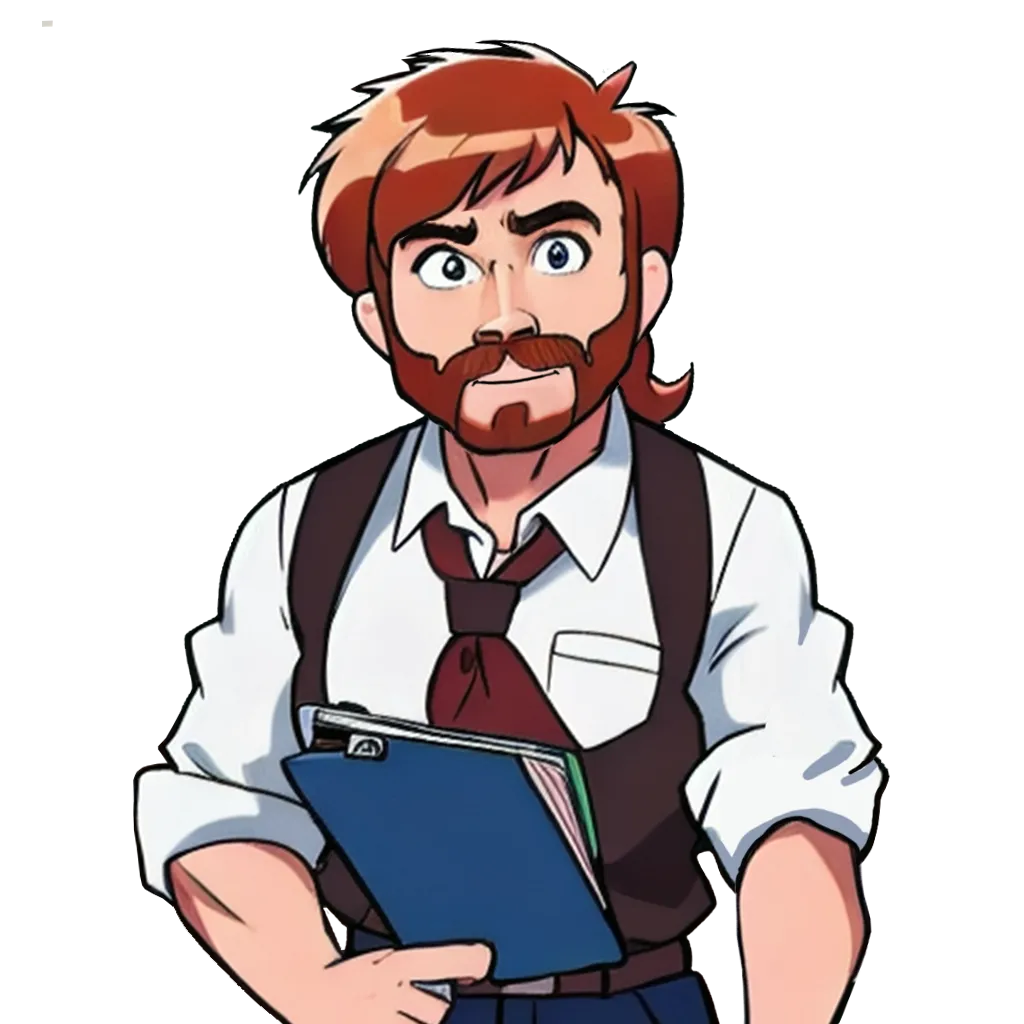
- Introduction to Node.js
- Modules and Packages in Node.js
- Asynchrony in Node.js
- Creating a REST API with Express
- Data Management in Node.js
- Authentication and Authorization
- File Handling and Uploads
- Testing in Node.js
- Security in Node.js Applications
- WebSocket Implementation
- Deployment and Scalability in Node.js
- Monitorización y Mantenimiento
- Alternatives to Express and Fetch
- Conclusions and Best Practices in Node.js