Node.js
Data Management in Node.js
Data management is one of the fundamental pillars in backend development. In Node.js, we can interact with databases efficiently using both SQL and NoSQL databases. In this chapter, we will learn how to connect to relational and non-relational databases, and perform CRUD (Create, Read, Update, Delete) operations on them.
Connecting to Databases in Node.js
Node.js makes it easy to connect to multiple types of databases using specific libraries. Below, we will explore how to work with MySQL (SQL) and MongoDB (NoSQL).
Connecting to MySQL with Node.js
MySQL is one of the most widely used relational databases in backend development. To connect to a MySQL database in Node.js, we use the mysql2
package.
First, let's install the package:
bash
Once installed, we can set up a basic connection to MySQL as follows:
javascript
Connecting to MongoDB with Node.js
MongoDB is a very popular NoSQL database in the world of web development. To connect to MongoDB in Node.js, we use the mongoose
package, which facilitates interaction with this database.
Install mongoose
:
bash
Here is an example of how to connect to a MongoDB database using Mongoose:
javascript
CRUD Operations in Databases
Once we have the connection established, we can perform CRUD operations. Below, we will see examples of how to perform these operations in both MySQL and MongoDB.
CRUD Operations in MySQL
CREATE
To insert a new record into a MySQL table, we use the following code:
javascript
READ
To fetch data from a table, we do the following:
javascript
UPDATE
To update an existing record in MySQL, we use the following code:
javascript
DELETE
Finally, to delete a record, we use the following code:
javascript
CRUD Operations in MongoDB
CREATE
To insert a new document into a MongoDB collection, we use the following code:
javascript
READ
To fetch documents from a MongoDB collection, we do the following:
javascript
UPDATE
To update an existing document, we use the following code:
javascript
DELETE
To delete a document from the collection, we use:
javascript
Summary
In this chapter, we have learned to connect our Node.js applications to relational (MySQL) and non-relational (MongoDB) databases, and perform CRUD operations on both. These skills are fundamental for any modern backend application.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
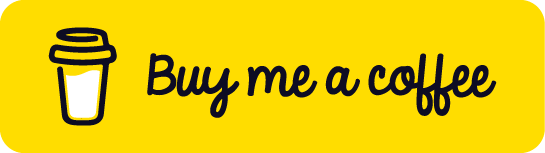
Chat with Chuck
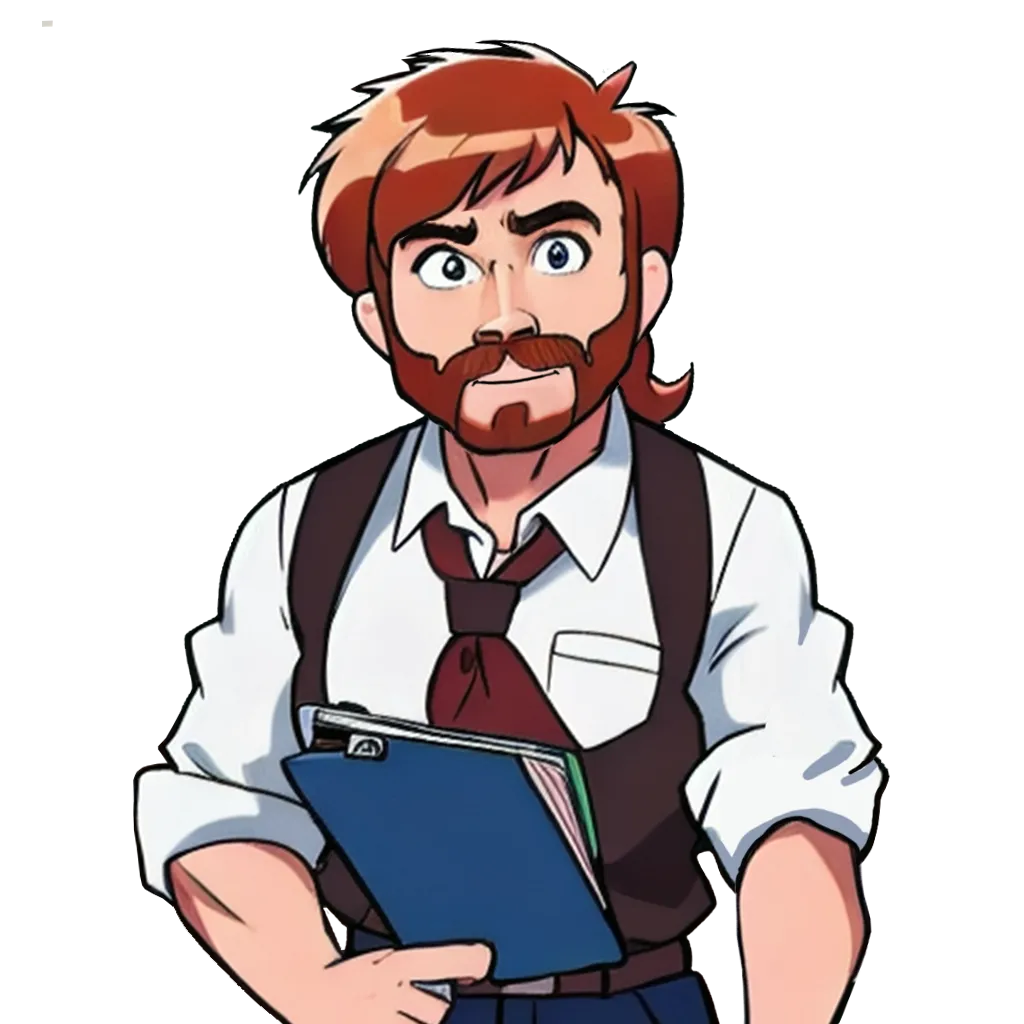
- Introduction to Node.js
- Modules and Packages in Node.js
- Asynchrony in Node.js
- Creating a REST API with Express
- Data Management in Node.js
- Authentication and Authorization
- File Handling and Uploads
- Testing in Node.js
- Security in Node.js Applications
- WebSocket Implementation
- Deployment and Scalability in Node.js
- Monitorización y Mantenimiento
- Alternatives to Express and Fetch
- Conclusions and Best Practices in Node.js