Node.js
Introduction to Node.js
Node.js is a JavaScript runtime environment that allows this language to be used outside the browser. Although JavaScript is traditionally known for running on the client side, Node.js extends its use to the server, making it an ideal option for developing scalable and efficient backend applications. In this chapter, we will explore the basic concepts of Node.js, its advantages, and how to start using it.
What is Node.js?
Node.js was created by Ryan Dahl in 2009 with the purpose of building fast and scalable applications, leveraging JavaScript's non-blocking I/O model. This makes it ideal for handling large volumes of simultaneous requests without saturating server resources.
Node.js uses Google's Chrome V8 engine, allowing it to execute JavaScript with high performance. It is also designed to handle input/output operations efficiently, preventing the server from waiting for time-consuming tasks like file reading or database requests.
Advantages of Node.js
Node.js offers several advantages for backend application development:
- Scalability: Its non-blocking nature allows handling many simultaneous connections, making it ideal for large-scale applications.
- Speed: By using the V8 engine, applications developed in Node.js tend to be fast and efficient.
- Single Language: You can write both the frontend and backend in JavaScript, simplifying the technology stack.
Getting Started with Node.js
Before starting to write code in Node.js, we need to install it on our machine. Follow these steps to install Node.js on a modern operating system like Windows, macOS, or Linux.
Node.js Installation
- Go to the official Node.js website and download the recommended version for your operating system.
- Follow the installer instructions. Ensure that the
node
binary is accessible from your command line. - Verify that the installation was successful by running the following command in the terminal:
bash
Creating a Basic Server with Node.js
Now that we have Node.js installed, let's create our first basic server.
Create a file named server.js
and write the following code:
javascript
To run the server, use the following command in the terminal:
bash
Summary
Node.js is a powerful and flexible platform for backend development. Its ability to handle multiple requests efficiently, along with its speed and simplicity, has made it an indispensable tool for developers worldwide. In the upcoming chapters, we will delve deeper into how to make the most of Node.js to create REST APIs, manage authentication, and much more.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
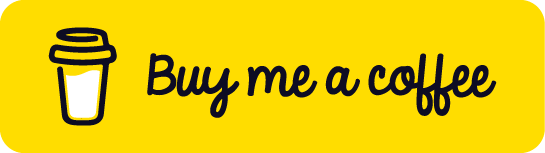
Chat with Chuck
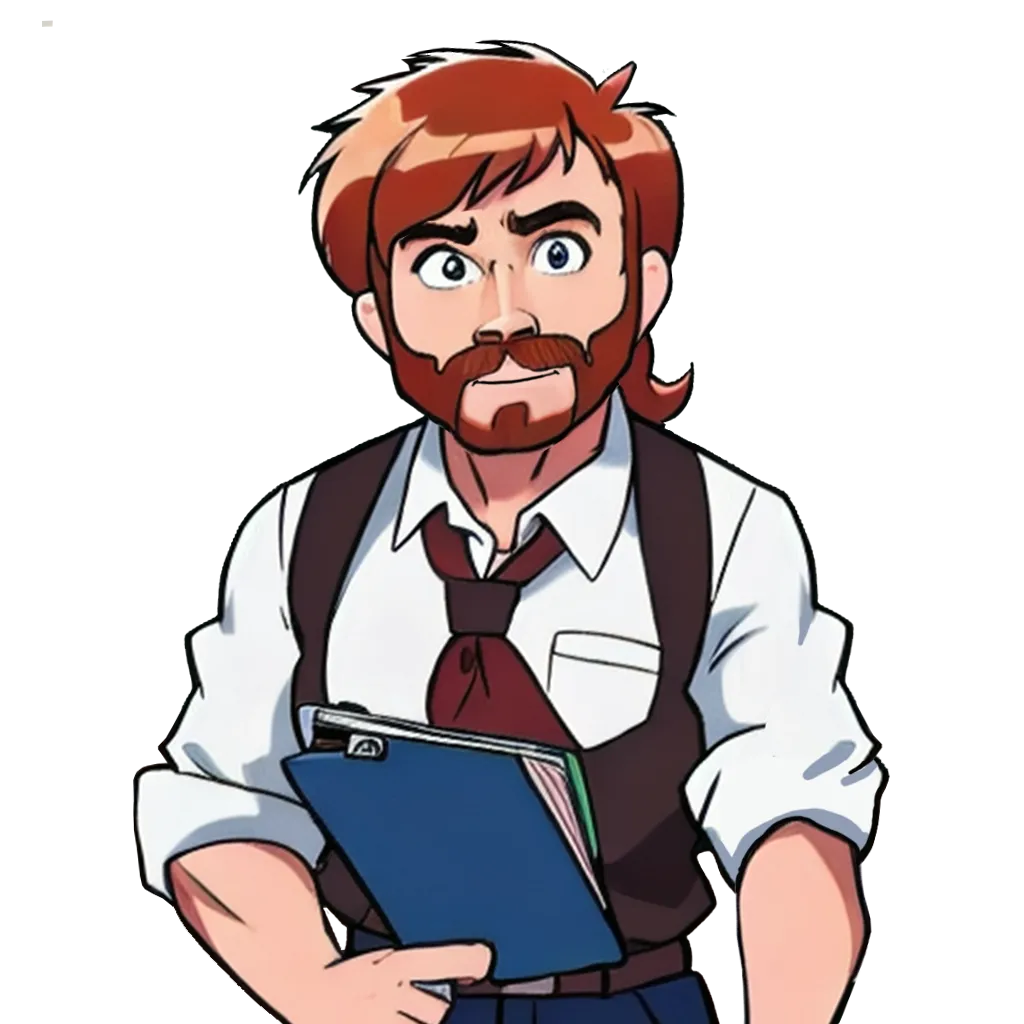
- Introduction to Node.js
- Modules and Packages in Node.js
- Asynchrony in Node.js
- Creating a REST API with Express
- Data Management in Node.js
- Authentication and Authorization
- File Handling and Uploads
- Testing in Node.js
- Security in Node.js Applications
- WebSocket Implementation
- Deployment and Scalability in Node.js
- Monitorización y Mantenimiento
- Alternatives to Express and Fetch
- Conclusions and Best Practices in Node.js