Node.js
WebSocket Implementation
WebSockets are a protocol that enables bidirectional communication between a server and a client, making them ideal for applications requiring real-time updates, such as chats, online games, or live tracking applications. Unlike HTTP, where requests must be initiated by the client, WebSockets allow the server to send data to the client without waiting for a prior request.
In this chapter, we will learn how to implement WebSockets in Node.js using the ws
and Socket.io
packages, and explore practical use cases.
What are WebSockets?
WebSockets establish a persistent connection between the client and the server, allowing efficient communication in both directions. This is ideal for real-time applications, where events or data must flow continuously without the client having to make new requests.
Installing ws
ws
is one of the most widely used libraries for working with WebSockets in Node.js. It is lightweight and easy to use. Let's install it:
bash
Creating a Basic WebSocket Server
To create a simple WebSocket server, we use ws
. Here is a basic example:
javascript
To connect to this server from a client, you can use the following code in the browser:
javascript
Implementing a Real-Time Chat with WebSockets
One of the most common use cases for WebSockets is implementing real-time chats. Here we show you how to set up a basic chat where multiple clients can connect and send messages through the server.
Server Configuration
First, we define the server that will manage client connections and relay messages to all connected users:
javascript
Client Configuration
Now, let's create the client that will allow users to connect to the chat and send messages:
javascript
Alternative: Using Socket.io
Socket.io
is a more advanced library that not only handles WebSockets but also offers support for other transport methods in case WebSockets are not available. Additionally, it offers advanced features such as automatic reconnection and grouping clients in rooms.
Installing Socket.io
First, let's install Socket.io
:
bash
Creating a Server with Socket.io
Here is a basic example of how to set up a server with Socket.io
:
javascript
Client with Socket.io
Here is the client code that connects to the Socket.io
server and sends messages:
javascript
Use Cases for WebSockets
WebSockets are used in a variety of applications that require real-time communication. Some examples include:
-
Real-time chats: As we have seen, WebSockets are ideal for chats where messages need to be transmitted instantly between multiple users.
-
Multiplayer games: WebSockets allow players to interact in real time, updating the game state without delays.
-
Live monitoring: In applications where data needs to be constantly updated (such as monitoring dashboards or financial applications), WebSockets enable quick and efficient updates.
Summary
In this chapter, we have learned to implement WebSockets in Node.js using the ws
and Socket.io
libraries. WebSockets allow bidirectional communication between the client and the server, which is ideal for real-time applications like chats, games, and monitoring systems. We also explored how to use Socket.io
as a more advanced alternative that automatically handles reconnections and other network issues.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
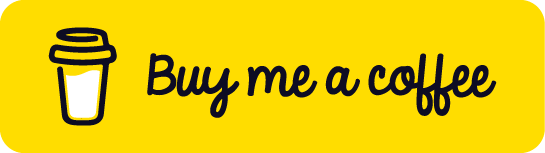
Chat with Chuck
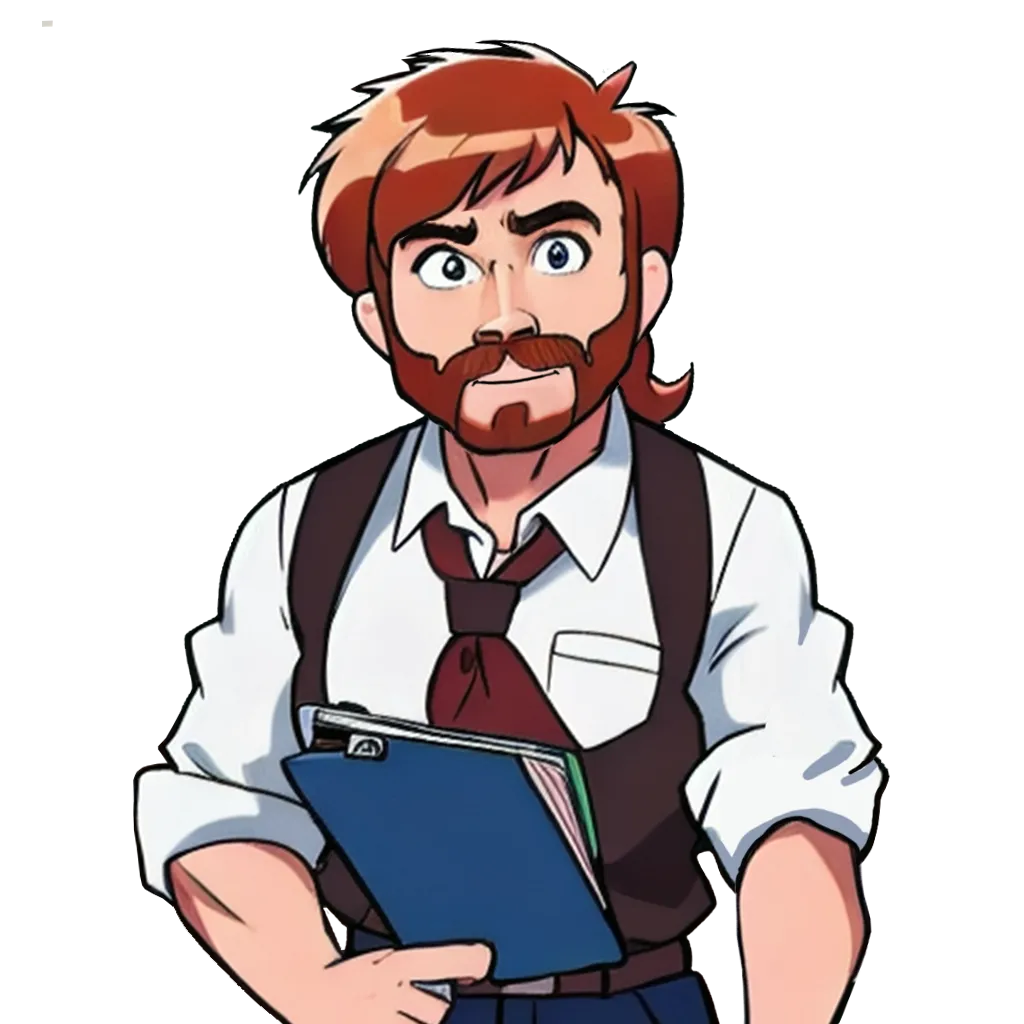
- Introduction to Node.js
- Modules and Packages in Node.js
- Asynchrony in Node.js
- Creating a REST API with Express
- Data Management in Node.js
- Authentication and Authorization
- File Handling and Uploads
- Testing in Node.js
- Security in Node.js Applications
- WebSocket Implementation
- Deployment and Scalability in Node.js
- Monitorización y Mantenimiento
- Alternatives to Express and Fetch
- Conclusions and Best Practices in Node.js