Node.js
Security in Node.js Applications
Security is a fundamental aspect of backend application development, especially when handling sensitive data or interacting with external users. Node.js, like any other platform, can be vulnerable to a series of attacks if the appropriate precautions are not taken. In this chapter, we will explore how to protect our Node.js applications against common attacks, such as Cross-Site Scripting (XSS), Cross-Site Request Forgery (CSRF), and SQL Injection, among others.
Preventing Cross-Site Scripting (XSS)
XSS is a type of attack where an attacker injects malicious code (like scripts) into an application, which is then executed in the browser of other users. To prevent this type of attack, it is important to validate and sanitize any user input.
Input Sanitization
We can use libraries like DOMPurify
or xss-clean
to clean user inputs and remove any malicious scripts. Here is an example using xss-clean
on an Express server:
bash
Then, in your Express application, you can apply the xss-clean
middleware as follows:
javascript
Preventing Cross-Site Request Forgery (CSRF)
CSRF is an attack where an attacker tricks an authenticated user into performing unwanted actions on a web application. To prevent this type of attack, we can use CSRF tokens to ensure the request comes from a valid origin.
Implementing CSRF Protection
Express has a middleware called csurf
that provides protection against CSRF. First, let's install the package:
bash
Then, configure the csurf
middleware in your application:
javascript
Preventing SQL Injection
SQL injection occurs when an attacker manipulates an SQL query by inserting malicious commands into input fields. To avoid this type of attack, it is important to never include user inputs directly in SQL queries.
Use of Parameterized Queries
Parameterized queries allow separating user data from SQL queries, preventing malicious data from altering the query behavior. Here is an example using mysql2
:
javascript
Securing HTTP Headers
Another important aspect of security is ensuring that HTTP headers are configured correctly. We can use the helmet
library to secure the headers of our Node.js applications.
Installation and Configuration of Helmet
Helmet helps protect applications by adjusting various HTTP headers to prevent attacks. First, let's install helmet
:
bash
Then, configure it in your Express application:
javascript
Using HTTPS
The use of HTTPS instead of HTTP ensures that the communication between the server and the client is encrypted, which protects against "man-in-the-middle" attacks. To enable HTTPS in Node.js, you will need an SSL certificate.
Here is a basic example of how to set up an HTTPS server:
javascript
Summary
In this chapter, we have covered several strategies to improve the security of Node.js applications, including preventing XSS, CSRF, and SQL injection attacks, using parameterized queries, and configuring secure HTTP headers with Helmet. We have also explored the importance of using HTTPS to protect communications.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
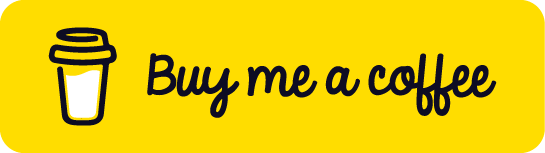
Chat with Chuck
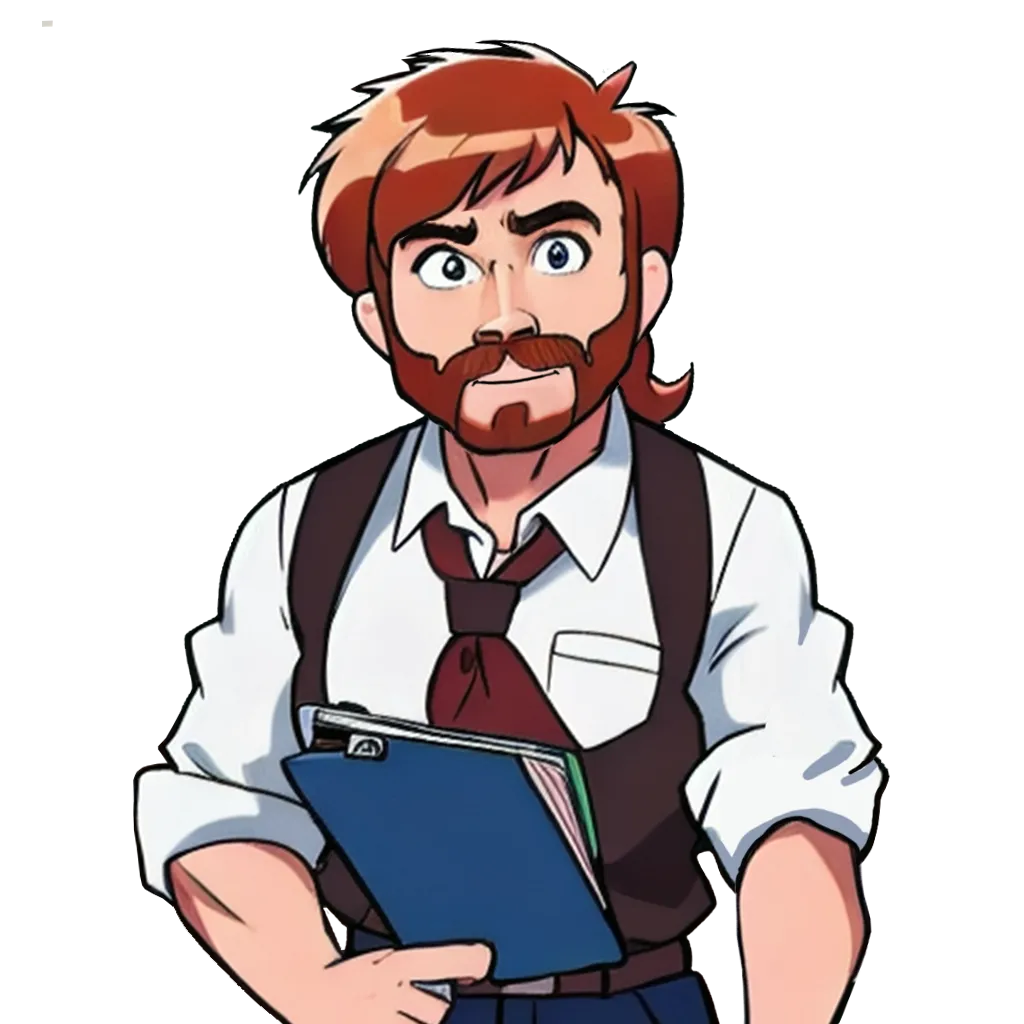
- Introduction to Node.js
- Modules and Packages in Node.js
- Asynchrony in Node.js
- Creating a REST API with Express
- Data Management in Node.js
- Authentication and Authorization
- File Handling and Uploads
- Testing in Node.js
- Security in Node.js Applications
- WebSocket Implementation
- Deployment and Scalability in Node.js
- Monitorización y Mantenimiento
- Alternatives to Express and Fetch
- Conclusions and Best Practices in Node.js