Node.js
Conclusions and Best Practices in Node.js
Congratulations on reaching the end of the Node.js course! Throughout these chapters, we covered the essential concepts and tools for working with Node.js in backend development, from creating basic servers to implementing REST APIs, managing databases, authentication, deployment and scalability, monitoring and maintenance, and much more.
In this final chapter, we will review the course and discuss some best practices to ensure that your Node.js applications are efficient, secure, and easy to maintain.
General Review
Throughout the course, you have learned to:
-
Create Servers and REST APIs: We used Express to build servers and APIs, configuring routes, handling HTTP methods, and responding to client requests.
-
Data Management: We learned to connect to SQL and NoSQL databases, perform CRUD operations, and ensure data persistence in our applications.
-
Authentication and Authorization: We implemented secure authentication using JWT and explored the use of roles and permissions to control access to application resources.
-
Deployment and Scalability: We learned to deploy applications on Heroku and AWS, as well as scaling our applications horizontally using tools like PM2.
-
Monitoring and Maintenance: We covered how to monitor applications in production with tools like PM2, New Relic, and Sentry, and how to handle errors effectively.
Best Practices in Node.js
To develop quality Node.js applications, it's important to follow a set of best practices that not only improve your code's efficiency but also its security and long-term maintainability.
1. Keep Your Code Modular and Reusable
Dividing the code into modules helps keep it organized and facilitates its reuse. Use require
or import
to include modules as needed and ensure each module fulfills a unique responsibility.
javascript
2. Proper Error Handling
It is essential to capture and handle errors appropriately, both in synchronous and asynchronous applications. Always capture errors with try/catch
blocks in code that uses async/await
or handle errors with callbacks.
javascript
3. Use a .env
File for Environment Variables
Do not store sensitive information like passwords, API keys, or environment-specific configurations directly in your code. Use a .env
file to store these environment variables and keep this file out of version control.
env
4. Secure Your Application
Security is essential. Always use HTTPS to protect communications, validate and sanitize all user inputs to prevent attacks such as XSS and SQL injection, and ensure that passwords and other sensitive data are correctly encrypted.
javascript
5. Optimize Performance
To improve your applications' performance, ensure you handle asynchronicity correctly and use the right tools to avoid blockages. Avoid operations that could block the execution thread, and use caching techniques when necessary.
6. Implement Testing
Testing is essential to ensure your code's quality. Implement unit tests for critical functions and integration tests to ensure the different components of your application work well together.
bash
7. Document Your Code
Proper documentation of your code facilitates teamwork and long-term maintenance. Be sure to include helpful comments and clear descriptions in important functions and modules.
8. Keep Your Dependencies Updated
Always keep your dependencies updated to take advantage of the latest security and performance improvements. Use tools like npm audit
to identify vulnerabilities in the dependencies.
bash
9. Use LTS Versions of Node.js
It is recommended to use long-term support (LTS) versions of Node.js to ensure stability and long-term security updates.
10. Automate Deployment and Maintenance
Use CI/CD pipelines to automate the deployment and monitoring process. This ensures that changes to your application are deployed efficiently and without errors.
Additional Resources
To continue learning and honing your Node.js skills, we recommend the following resources:
- Official Node.js Documentation: https://nodejs.org/en/docs/
- Express.js Guide: https://expressjs.com/
- MongoDB API: https://docs.mongodb.com/
- MySQL API: https://dev.mysql.com/doc/
Conclusion
Node.js is a powerful tool for backend development, and with the right best practices, you can create scalable, secure, and efficient applications. We hope this course has provided you with the foundations and tools needed to continue building complex solutions in Node.js.
Remember to keep learning, experiment with new technologies, and hone your skills with real projects. Thank you for completing the course and good luck on your journey as a Node.js developer!
You can also deepen in the following topics:
- Streaming and Buffering in Node
- Middlewares in Node
- Node with Express JS
- Security in Node
- Working with Images in Node
- Microservices in Node
- GraphQL with Node
- WebSockets with Node
- Testing in Node.js with Mocha and Chai
- Testing in Node.js with Jest
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
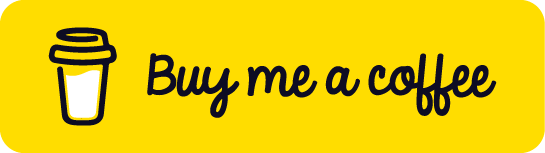
Chat with Chuck
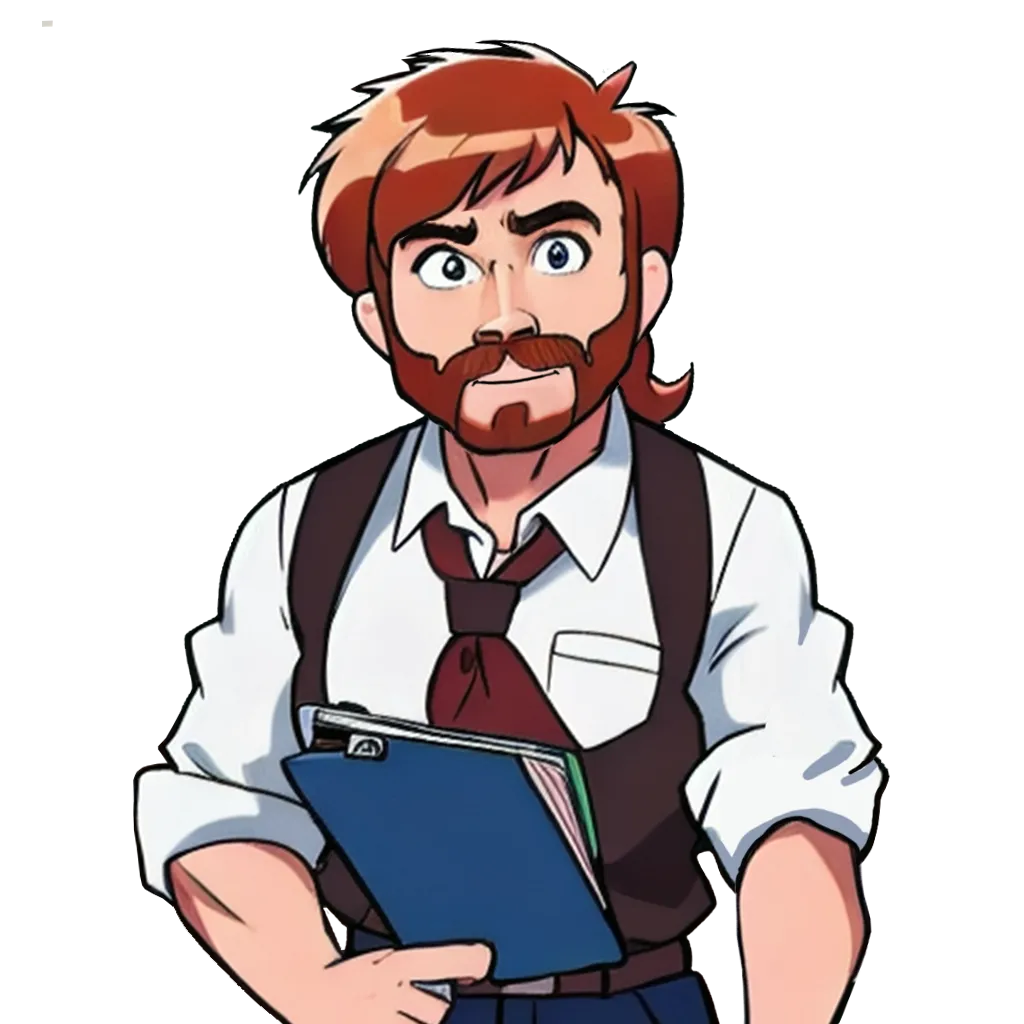
- Introduction to Node.js
- Modules and Packages in Node.js
- Asynchrony in Node.js
- Creating a REST API with Express
- Data Management in Node.js
- Authentication and Authorization
- File Handling and Uploads
- Testing in Node.js
- Security in Node.js Applications
- WebSocket Implementation
- Deployment and Scalability in Node.js
- Monitorización y Mantenimiento
- Alternatives to Express and Fetch
- Conclusions and Best Practices in Node.js