Node.js
File Handling and Uploads
File handling is a common task in backend applications, from reading and writing files on the server to managing user file uploads. Node.js offers powerful tools to work with files efficiently. In this chapter, we will learn how to handle files and manage file uploads in Node.js using fs
(file system) and multer
.
Reading and Writing Files in Node.js
Node.js includes the fs
module to work with the file system. This module allows you to perform operations such as reading, writing, deleting, and renaming files on the server. Let's see how to perform some of the most common operations.
Reading Files
To read the content of a file, we use the fs.readFile
method. Here's an example:
javascript
Writing Files
To write to a file, we can use fs.writeFile
. If the file does not exist, it will be created; if it already exists, its content will be overwritten.
javascript
Deleting Files
To delete a file, we use fs.unlink
:
javascript
File Upload in Node.js with Multer
multer
is a middleware that makes managing file uploads in Node.js easier. With multer
, we can handle files sent through HTML forms and store them on the server. First, let's install the package:
bash
Configuring Multer
To handle file uploads, we need to configure multer on our Express server. Let's see how to do it:
javascript
Uploading Multiple Files
If we need to allow the uploading of several files at once, we can use upload.array()
instead of upload.single()
:
javascript
Custom Storage
Multer also allows configuring more advanced storage options like renaming files and defining where they will be stored. Let's see how to configure custom storage:
javascript
Security Considerations for File Handling
File handling can introduce security vulnerabilities if proper measures are not taken. Here are some tips to improve security when handling files in Node.js:
-
File type validation: Ensure you validate the file type users can upload to prevent the execution of malicious files.
-
File size: Set a limit on the size of files users can upload to avoid overloading the server.
-
File names: Generate unique names for uploaded files to avoid overwriting existing files.
javascript
Summary
In this chapter, we learned how to handle files in Node.js using the fs
module to read, write, and delete files, and how to manage file uploads using the multer
middleware. These skills are fundamental for many modern applications, from image storage to document management.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
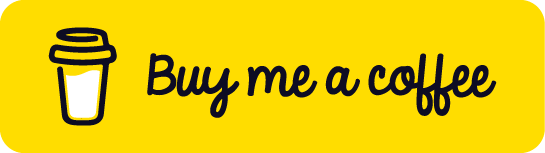
Chat with Chuck
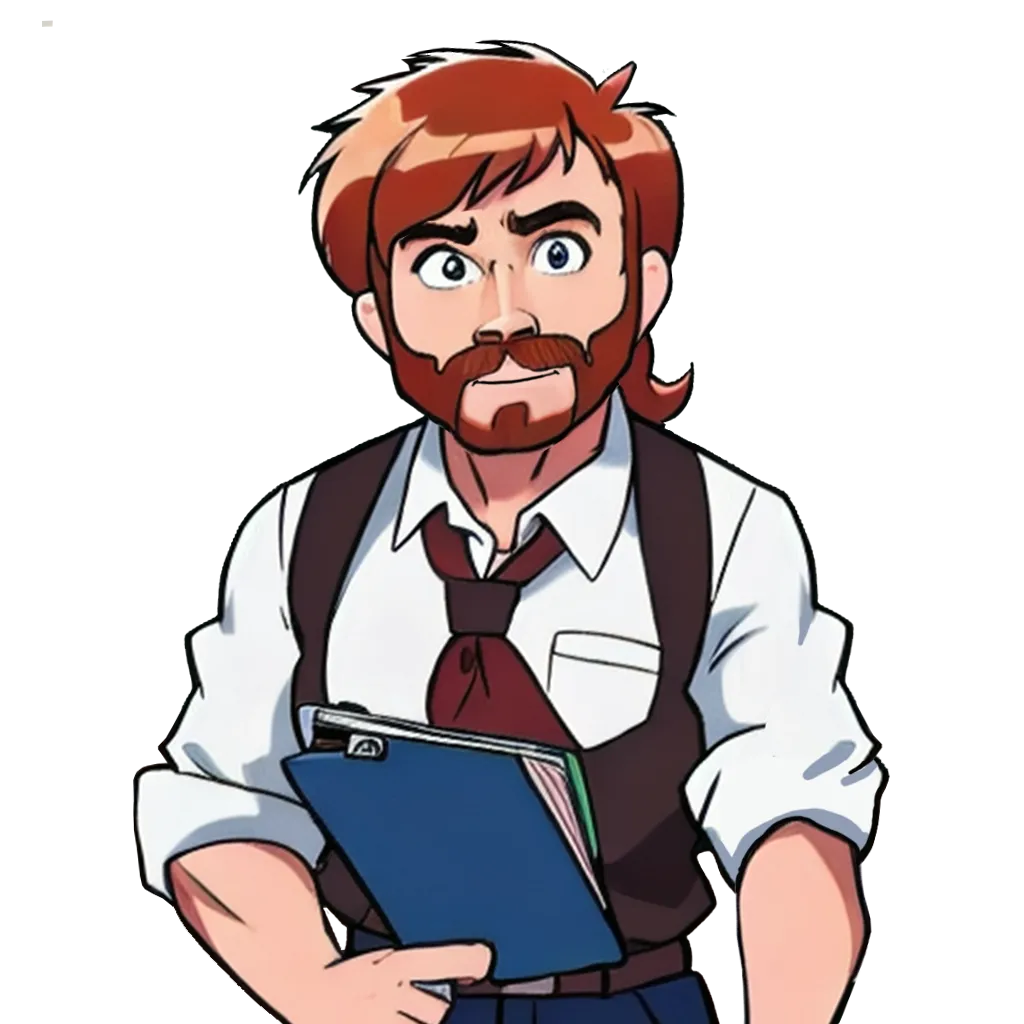
- Introduction to Node.js
- Modules and Packages in Node.js
- Asynchrony in Node.js
- Creating a REST API with Express
- Data Management in Node.js
- Authentication and Authorization
- File Handling and Uploads
- Testing in Node.js
- Security in Node.js Applications
- WebSocket Implementation
- Deployment and Scalability in Node.js
- Monitorización y Mantenimiento
- Alternatives to Express and Fetch
- Conclusions and Best Practices in Node.js