Node.js
Deployment and Scalability in Node.js
Deploying Node.js applications efficiently and scalable is crucial to ensure their performance and availability in a production environment. In this chapter, we will learn how to configure different development environments, deploy a Node.js application on popular platforms like Heroku and AWS, use tools like PM2 to manage processes, and scale our application horizontally.
Environment Configuration
Before deploying an application, it is important to manage development, testing, and production environments. Node.js allows configuring environment variables to control application behavior in different contexts.
Using Environment Variables
In Node.js, we can use the process.env
module to access environment variables. A common approach is to use an .env
file to define these variables:
- Install
dotenv
:
bash
- Create an
.env
file:
- Load the variables in your Node.js application:
javascript
Deployment on Heroku
Heroku is a cloud platform that makes it easy to deploy Node.js applications. To deploy your application on Heroku, follow these steps:
- Install the Heroku CLI tool:
bash
- Log in to Heroku:
bash
- Create a new application on Heroku:
bash
- Deploy your application using Git:
bash
- Open the application in your browser:
bash
Automatic Deployment with Heroku Pipelines
Heroku also supports pipelines, allowing you to configure automatic deployments every time you commit to a specific branch, such as main
or production
. This facilitates the continuous development cycle.
Deployment on AWS (Amazon Web Services)
AWS offers a variety of services to deploy Node.js applications, one of the most used being Elastic Beanstalk. Elastic Beanstalk automatically manages the infrastructure needed to run your application, allowing you to focus only on the code.
Deployment with Elastic Beanstalk
- Install the Elastic Beanstalk CLI:
bash
- Initialize your project in Elastic Beanstalk:
bash
- Deploy the application:
bash
- Monitor your application:
bash
Alternatives: Amazon EC2 and AWS Lambda
Besides Elastic Beanstalk, you can also use Amazon EC2 to have more control over the servers or AWS Lambda to deploy functions without the need to manage servers.
Process Management with PM2
PM2 is a tool that facilitates the management and monitoring of Node.js processes. It allows automatic application restarts in case of failures, manages multiple instances, and provides load balancing support.
Installation of PM2
First, let's install PM2:
bash
Run the Application with PM2
To run your application with PM2 and ensure that it automatically restarts in case of failures, use the following command:
bash
Monitoring Processes with PM2
PM2 offers a real-time monitoring interface to see the status of all processes:
bash
Automatic Restarts in Case of Failure
PM2 will automatically restart any application that fails, and you can configure PM2 to automatically start your application when the server boots:
bash
Horizontal Scalability
Horizontal scaling means adding more instances of the application to distribute the load across multiple servers. PM2 facilitates load balancing between several instances on the same server.
To run multiple instances of your application on a single server, use:
bash
To scale horizontally across multiple servers, you can use platforms like AWS, Heroku, or container services like Docker to distribute the load between multiple machines.
Summary
In this chapter, we have learned how to deploy Node.js applications on popular platforms like Heroku and AWS, and how to manage processes and scale applications using PM2. Deploying and scaling applications efficiently is crucial to ensure that our applications can handle high traffic volume and continue to operate without interruptions.
- Introduction to Node.js
- Modules and Packages in Node.js
- Asynchrony in Node.js
- Creating a REST API with Express
- Data Management in Node.js
- Authentication and Authorization
- File Handling and Uploads
- Testing in Node.js
- Security in Node.js Applications
- WebSocket Implementation
- Deployment and Scalability in Node.js
- Monitorización y Mantenimiento
- Alternatives to Express and Fetch
- Conclusions and Best Practices in Node.js
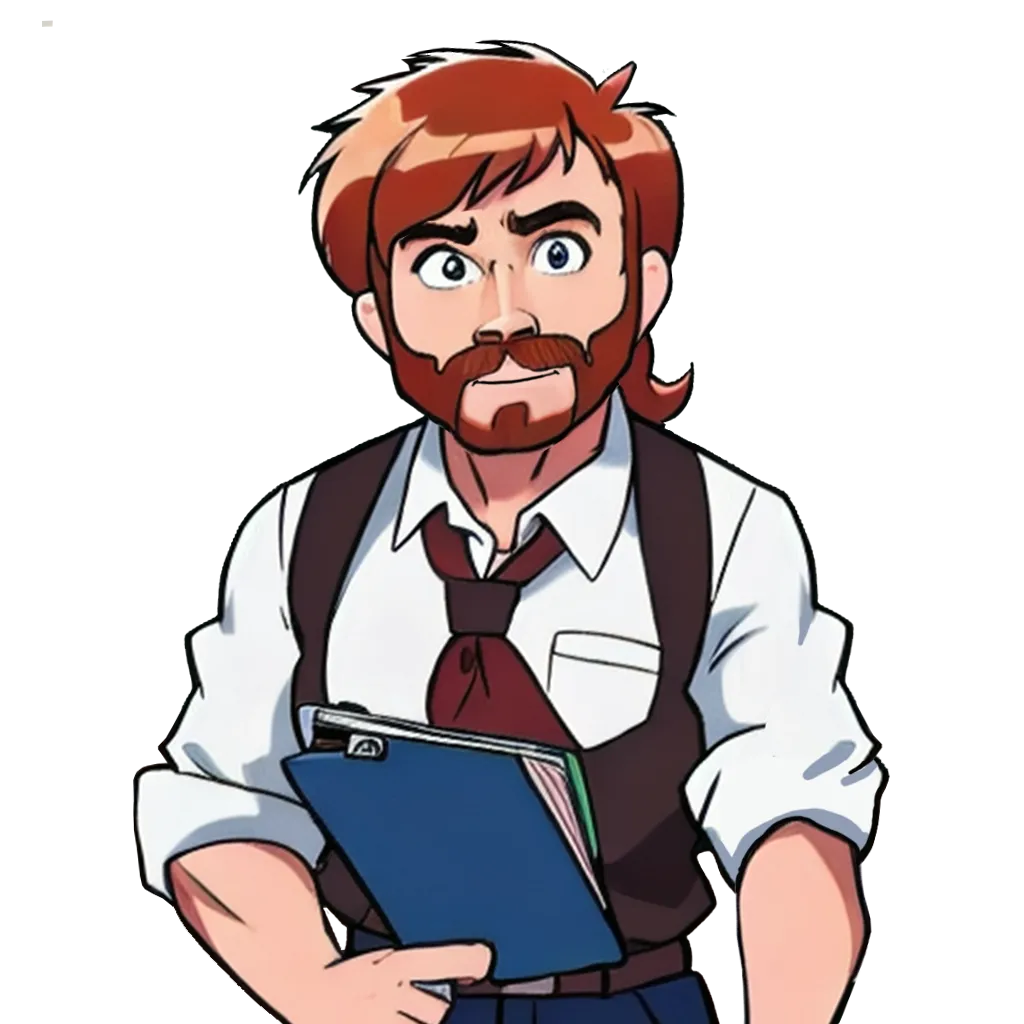