Node.js
Creating a REST API with Express
Express is one of the most popular and widely used frameworks in Node.js for creating REST APIs. Its simplicity and flexibility allow building robust applications quickly. In this chapter, we will learn how to create a REST API using Express, handling routes and HTTP requests like GET, POST, PUT, and DELETE.
What is Express?
Express is a minimalist web framework for Node.js that provides a set of essential features for web application and API development. Through Express, we can manage routes, handle middleware, and process HTTP requests with great ease.
Installing Express
To use Express in our project, we first need to install it. If you haven't done so, make sure to initialize your Node.js project by running npm init -y
. Then, install Express with the following command:
bash
Setting up a basic server with Express
Once installed, let's set up a basic server using Express. Create a file named app.js
and write the following code:
javascript
To run your server, use the following command in the terminal:
bash
Routes and HTTP Methods
In a REST API, each route corresponds to a URL that the client can access, and HTTP methods like GET, POST, PUT, and DELETE define the operations that can be performed at that URL.
Handling GET requests
GET requests are used to retrieve information from the server. Let's see how to define a GET route that returns a list of users:
javascript
Handling POST requests
POST requests are used to send data to the server, usually to create new resources. To handle a POST, we need middleware that allows us to read the request body, such as express.json()
.
javascript
Handling PUT requests
PUT requests are used to update an existing resource. Here we see how to handle a PUT request to update a user:
javascript
Handling DELETE requests
Lastly, DELETE requests are used to remove a resource. Here is an example to delete a user by their ID:
javascript
Summary
In this chapter, we have learned to create a basic REST API using Express. We covered how to handle HTTP GET, POST, PUT, and DELETE requests to interact with the server. Express allows us to create APIs in a simple and efficient way, making it an excellent tool for backend development.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
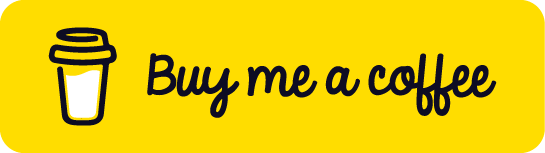
Chat with Chuck
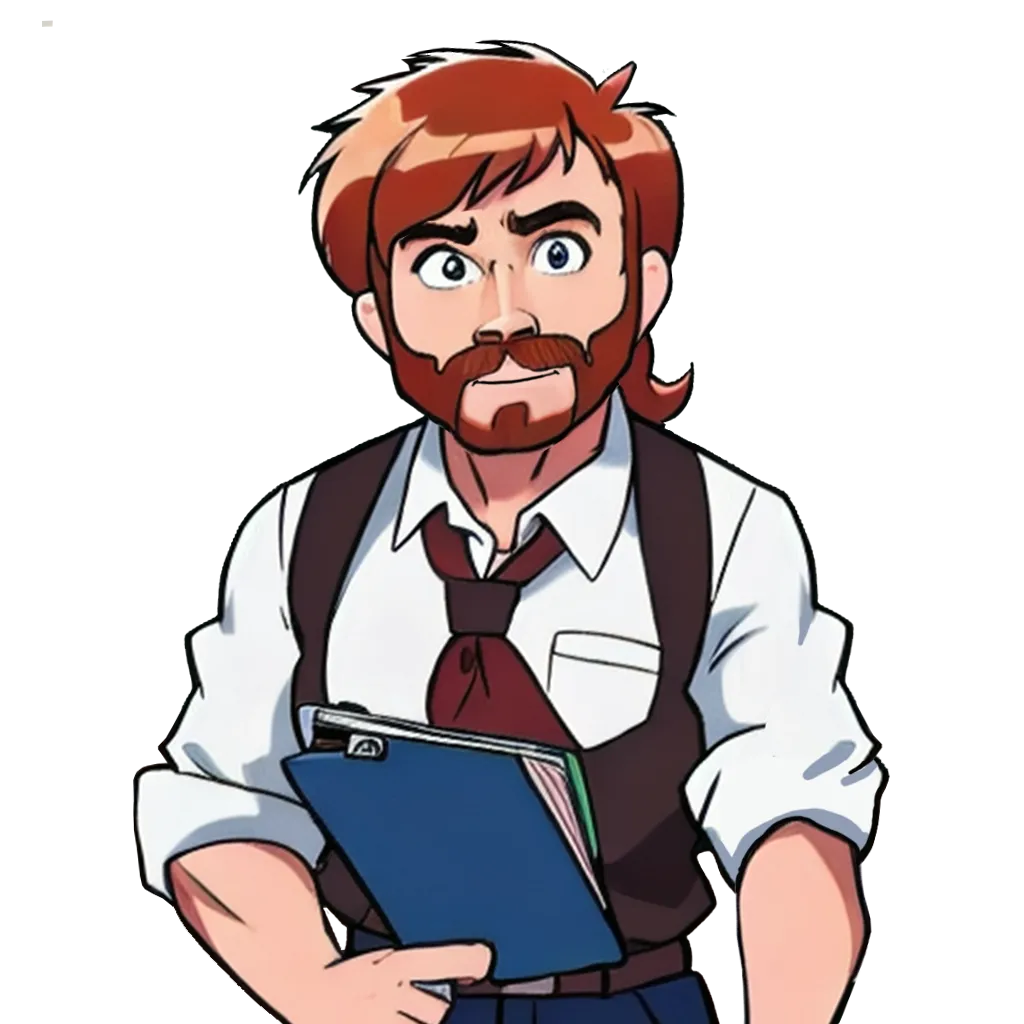
- Introduction to Node.js
- Modules and Packages in Node.js
- Asynchrony in Node.js
- Creating a REST API with Express
- Data Management in Node.js
- Authentication and Authorization
- File Handling and Uploads
- Testing in Node.js
- Security in Node.js Applications
- WebSocket Implementation
- Deployment and Scalability in Node.js
- Monitorización y Mantenimiento
- Alternatives to Express and Fetch
- Conclusions and Best Practices in Node.js