Node.js
Modules and Packages in Node.js
One of the most powerful aspects of Node.js is its module system, which allows you to split your code into smaller, reusable files. Additionally, with the use of npm (Node Package Manager), you can access thousands of packages that extend Node.js functionalities, simplifying the development of complex applications.
What are modules?
In Node.js, a module is simply a file that contains code. Every file in Node.js is a module, and you can use the module system to export functionalities from a file and utilize them in others.
For example, we can create a module that exports a greeting function and then use that module in another file.
Creating a Basic Module
Create a file named greet.js
with the following content:
javascript
Now, in a separate file, for example app.js
, we can import and use this function:
javascript
When you run app.js
, you should see the following result in the terminal:
bash
Working with Packages
Node.js includes npm, a package manager that allows you to install and share modules with other developers. npm is the world's largest package ecosystem, with thousands of packages available for you to use in your projects.
Installing a Package Using npm
Let's install the lodash
package, a popular utility library that makes working with arrays, objects, and other data structures easier.
First, initialize a new Node.js project by running the following command in the terminal:
bash
Then, install the lodash
package with the following command:
bash
Using an Installed Package
Once the package is installed, we can require it in our project like any other module:
javascript
When you run this code, you should see something like this:
bash
package.json and Dependency Management
The package.json
file is where npm stores all the information about your project, including the dependencies you've installed. Every time you install a package, npm adds it to this file automatically.
Here's an example of a package.json
file after installing lodash
:
json
Summary
In this chapter, we have learned how modules allow us to organize our code and how npm facilitates access to thousands of useful packages. This gives us the necessary tools to build complex and scalable applications in Node.js more efficiently.
- Introduction to Node.js
- Modules and Packages in Node.js
- Asynchrony in Node.js
- Creating a REST API with Express
- Data Management in Node.js
- Authentication and Authorization
- File Handling and Uploads
- Testing in Node.js
- Security in Node.js Applications
- WebSocket Implementation
- Deployment and Scalability in Node.js
- Monitorización y Mantenimiento
- Alternatives to Express and Fetch
- Conclusions and Best Practices in Node.js
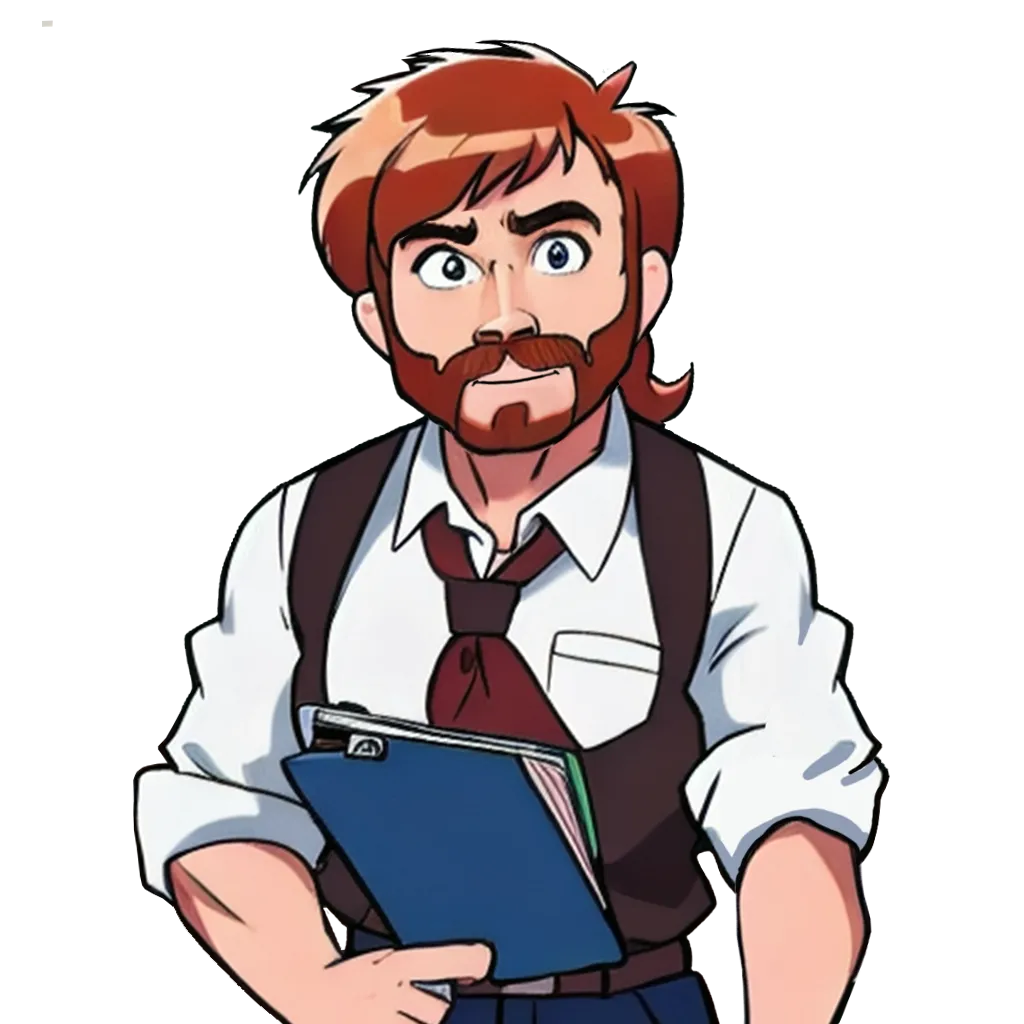