Node.js
Asynchrony in Node.js
One of the most important aspects of Node.js is its focus on asynchrony. Node.js is designed to handle multiple simultaneous operations without blocking the flow of the program, making it an ideal tool for building applications that need to scale and handle many connections at the same time. In this chapter, we will explore various mechanisms for asynchronous handling in Node.js, including callbacks, promises, and async/await
.
The non-blocking I/O model
Node.js follows a non-blocking I/O model, meaning it does not wait for input/output operations (such as reading a file or making a request to a database) to finish before continuing with the rest of the code. This allows Node.js to handle many operations simultaneously without blocking the main thread.
Callbacks in Node.js
Asynchronous handling in Node.js was originally based on callbacks, which are functions that execute when an operation has finished. Although callbacks are effective, they can become difficult to manage when there are multiple chained operations, leading to what is commonly known as "callback hell."
Here is a basic example of a callback:
javascript
Promises in Node.js
To improve asynchrony handling, promises were introduced in ES6 and are supported in Node.js. Promises allow us to chain asynchronous operations more cleanly, avoiding "callback hell."
Let's see the same file reading example using promises:
javascript
async/await
One of the most modern and clean ways to handle asynchrony in Node.js is using async/await
, introduced in ES8. async/await
makes it possible to write asynchronous code that looks synchronous, enhancing readability.
Here is the same example using async/await
:
javascript
Handling asynchronous errors
It is important to consider that error handling is crucial in asynchronous applications, as many errors can occur during input/output operations or when communicating with external databases.
When using callbacks, ensure to check for errors in each operation. With promises, you can use the .catch()
method, and with async/await
you can use try/catch
.
Conclusion
In this chapter, we have covered the main asynchronous handling mechanisms in Node.js: callbacks, promises, and async/await
. Each has its advantages, and it is important to understand when to use them. async/await
has been widely adopted due to its simplicity and readability, but you should also be familiar with callbacks and promises, as they are still used in many cases.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
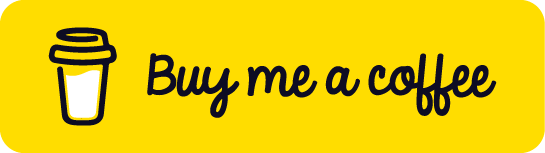
Chat with Chuck
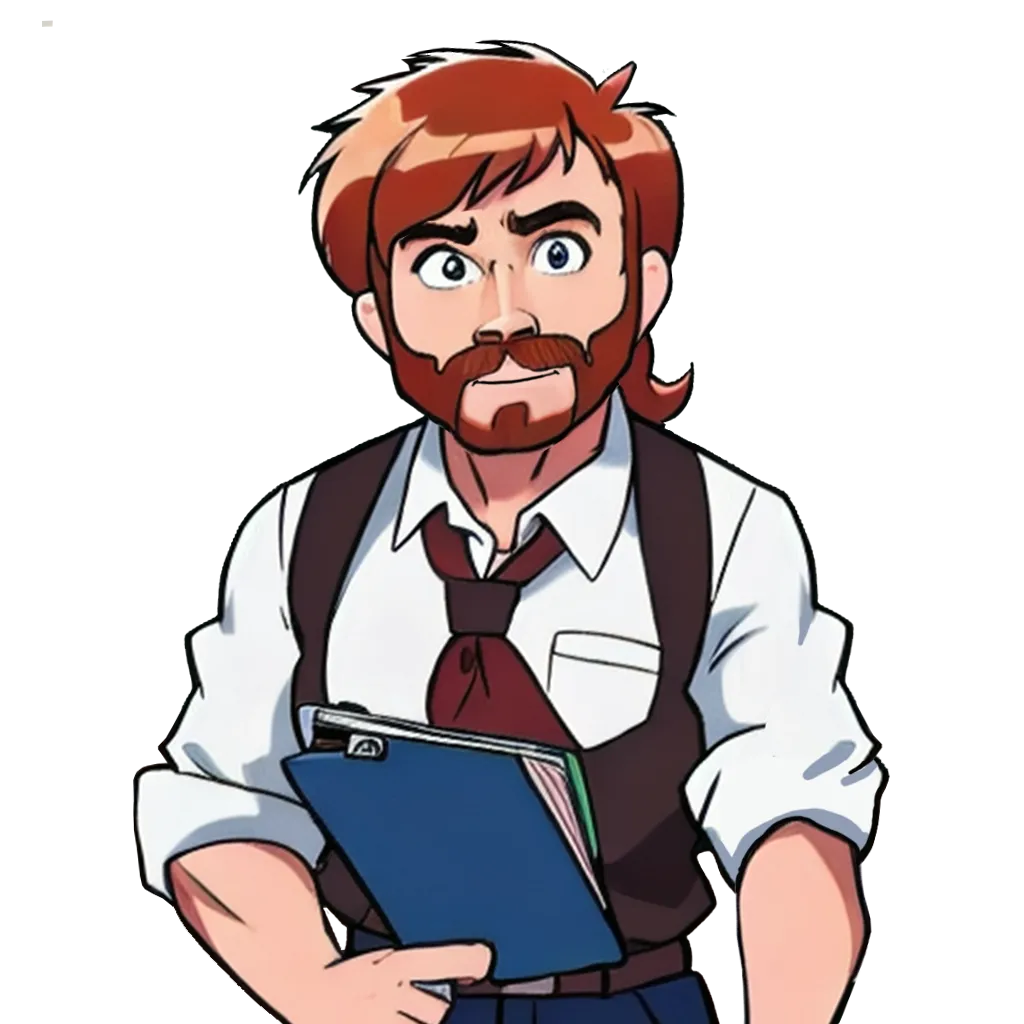
- Introduction to Node.js
- Modules and Packages in Node.js
- Asynchrony in Node.js
- Creating a REST API with Express
- Data Management in Node.js
- Authentication and Authorization
- File Handling and Uploads
- Testing in Node.js
- Security in Node.js Applications
- WebSocket Implementation
- Deployment and Scalability in Node.js
- Monitorización y Mantenimiento
- Alternatives to Express and Fetch
- Conclusions and Best Practices in Node.js