Testing in Node.js with Jest
Asserts and Matchers in Jest
In this section, we will delve into the assertions and matchers that Jest provides for evaluating conditions in our tests. Assertions and matchers are fundamental for comparing the expected result with the actual result of a function or process.
Assertions in Jest
In Jest, assertions are made using the expect()
function, which takes care of comparing values. The expect()
function takes a value as an argument and returns an object with several matchers that you can use to make specific comparisons.
javascript
Main Matchers in Jest
Below are some of the most common matchers:
Equality
-
toBe
: Compares using the===
operator.javascript -
toEqual
: Checks value equality, useful for objects and arrays.javascript
Truthiness
-
toBeNull
: Verifies if the value isnull
.javascript -
toBeUndefined
: Verifies if the value isundefined
.javascript -
toBeDefined
: Verifies if the value is notundefined
.javascript -
toBeTruthy
: Verifies if the value istrue
in a boolean context.javascript -
toBeFalsy
: Verifies if the value isfalse
in a boolean context.javascript
Numbers
-
toBeGreaterThan
: Verifies if a number is greater than another.javascript -
toBeGreaterThanOrEqual
: Verifies if a number is greater than or equal to another.javascript -
toBeLessThan
: Verifies if a number is less than another.javascript -
toBeLessThanOrEqual
: Verifies if a number is less than or equal to another.javascript -
toBeCloseTo
: Verifies if two numbers are close to each other, useful for decimal comparison.javascript
Strings
-
toMatch
: Verifies if a string matches a regular expression.javascript
Arrays and Sets
-
toContain
: Verifies if an element is in an array orSet
.javascript -
toContainEqual
: Verifies if an equivalent object is in an array.javascript
Exceptions
-
toThrow
: Verifies if a function throws an error when executed.javascript
Placeholder for an image: Comparative table showing different matchers with short examples and expected results.
Complete Example with Matchers
Below is an example where several of the mentioned matchers are used:
javascript
Conclusion
Mastering matchers and assertions in Jest will allow you to write more effective and precise tests. In the following chapters, we will explore advanced testing techniques, including TDD, mocks, and testing asynchronous functions.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
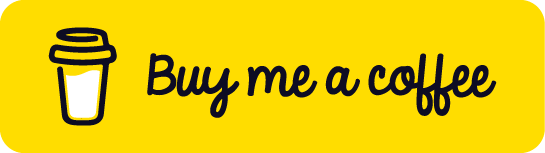
Chat with Chuck
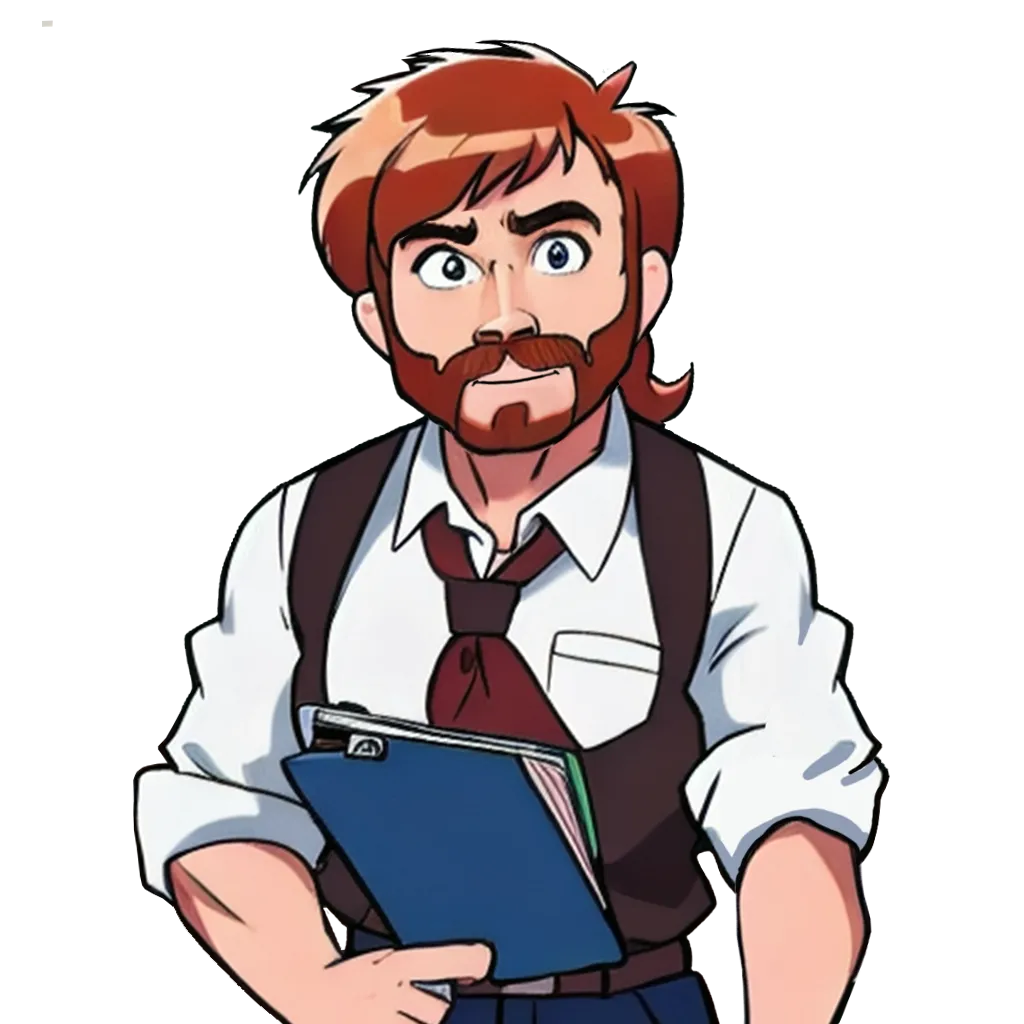
- Introduction to Testing in Node.js
- Installation and Configuration of Jest
- Basic Testing Concepts
- Structure of a Test with Jest
- Asserts and Matchers in Jest
- Test Driven Development (TDD) with Jest
- Mocks and Stubs in Jest
- Testing Asynchronous Functions
- Testing RESTful APIs
- Integration of Tests in the CI/CD Process
- Load and Performance Testing
- Best Practices in Testing
- Herramientas Complementarias para Testing
- Practical Examples and Use Cases
- Conclusions and Next Steps