Testing in Node.js with Jest
Basic Testing Concepts
In this section, we will address the fundamental testing concepts that every developer should know before diving into testing with Jest and Node.js. Familiarizing yourself with these concepts will facilitate the understanding and application of testing techniques in your projects.
Types of Testing
Testing can be classified into several types, each with its own specific objective and scope:
-
Unit Tests:
- Definition: Evaluate specific units of code in isolation, typically individual functions or methods.
- Objective: Ensure that each unit functions correctly on its own.
- Example:
javascript
-
Integration Tests:
- Definition: Verify that modules or services interact correctly with each other.
- Objective: Ensure that the integration of multiple units works as expected.
- Example:
javascript
-
Functional Tests:
- Definition: Evaluate if the system meets the specified functional requirements.
- Objective: Validate that the system performs all the functions needed by the user.
- Example:
javascript
-
Load and Performance Tests:
- Definition: Measure the system's behavior under specific workloads.
- Objective: Ensure that the system operates efficiently and within the required performance thresholds.
- Example: Run 1000 simultaneous requests and measure the response time.
Test Lifecycle
The lifecycle of a test generally follows these steps:
-
Setup:
- Set up the environment and prepare the data necessary for the test.
- Example:
javascript
-
Execution:
- Execute the unit or function being tested.
- Example:
javascript
-
Verification:
- Check the obtained results against the expected results using assertions.
- Example:
javascript
-
Teardown:
- Optionally, clean up the environment after the test to avoid interference with other tests.
- Example:
javascript
Assertions
Assertions are the core of any test. Assertions compare the result of executing your code with the expected result. If the comparison fails, the test will fail. Examples of assertions in Jest include toBe
, toEqual
, toBeTruthy
, etc.
javascript
[Placeholder for image: Diagram illustrating the test lifecycle with steps like Setup, Execution, Verification, and Teardown.]
Conclusion
Understanding these basic testing concepts is crucial for developing reliable and maintainable applications. In the following sections, we will delve into how to structure and write tests with Jest, as well as advanced testing techniques.
- Introduction to Testing in Node.js
- Installation and Configuration of Jest
- Basic Testing Concepts
- Structure of a Test with Jest
- Asserts and Matchers in Jest
- Test Driven Development (TDD) with Jest
- Mocks and Stubs in Jest
- Testing Asynchronous Functions
- Testing RESTful APIs
- Integration of Tests in the CI/CD Process
- Load and Performance Testing
- Best Practices in Testing
- Herramientas Complementarias para Testing
- Practical Examples and Use Cases
- Conclusions and Next Steps
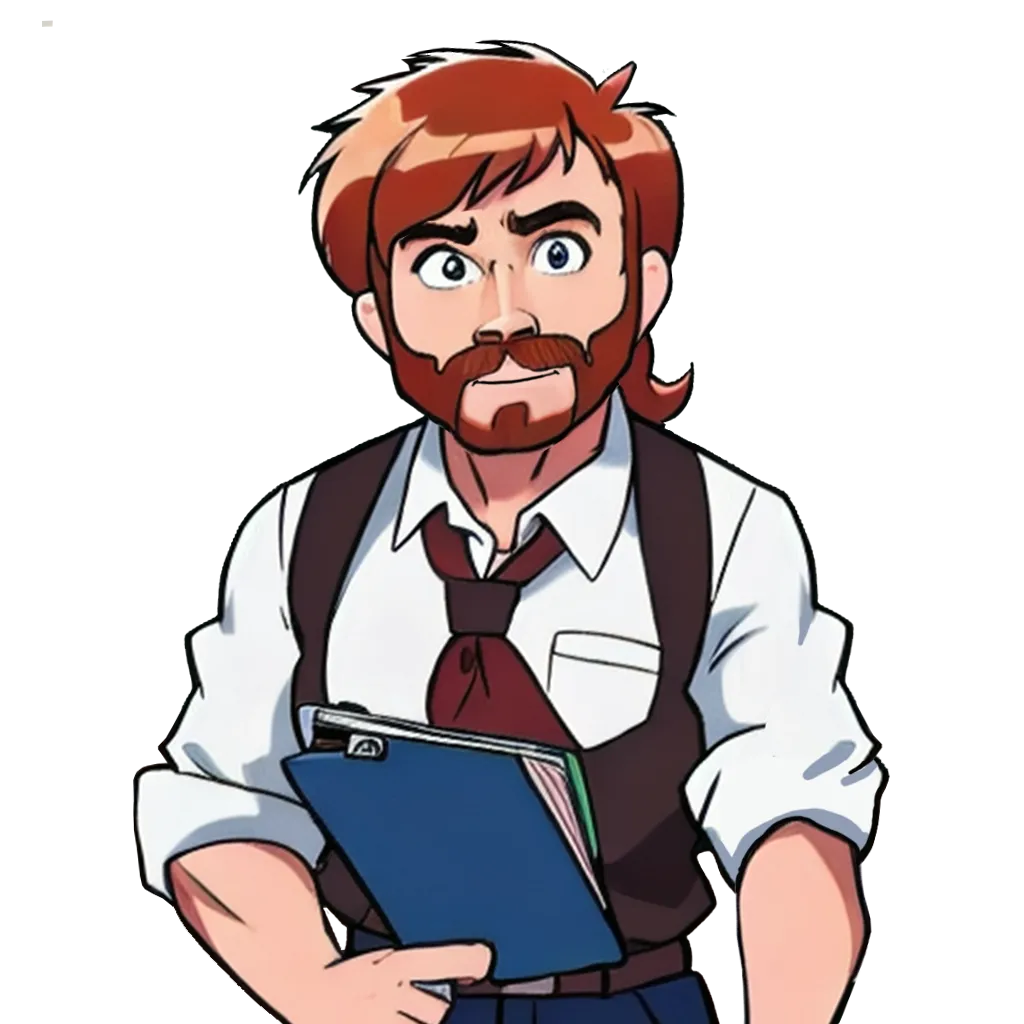