Testing in Node.js with Jest
Mocks and Stubs in Jest
In this chapter, we will explore how to use mocks and stubs in Jest. These testing techniques are essential for simulating behaviors and dependencies, allowing you to isolate the part of the code you want to test.
What are Mocks and Stubs?
-
Mocks: These are fake objects that mimic the behavior of real objects. They allow you to control how functions and methods are called and how they respond.
-
Stubs: These are variants of mocks that temporarily replace an implementation with a predefined behavior.
Mocks in Jest
Defining Mocks Manually
You can manually define a mock for a function or module. Here is a simple example:
javascript
Automatic Mocks
Jest provides various ways to create mocks. An effective way is to use jest.fn
to create mock functions.
javascript
Mocking Modules
You can mock entire modules using jest.mock()
. For example, if you have a utils
module and you want to mock a greet
function:
javascript
Stubs in Jest
Stubs in Jest are generally created using jest.fn
. You can predefine how a function should behave.
javascript
Simulating Dependencies
Often, it is necessary to simulate dependencies for isolated tests. For example, if a function depends on an external API call, you can mock that dependency.
javascript
Full Example: Mocks and Stubs in Action
Let's assume we want to test a function that depends on another function to fetch data from an API:
javascript
Our test using mocks and stubs would look something like this:
javascript
[Placeholder for image: Diagram showing the relationship between a function and its dependencies being replaced by mocks or stubs.]
Conclusion
Using mocks and stubs in Jest facilitates unit and component testing in isolation, allowing you to simulate dependencies and control the behavior of functions. In the next section, we will explore how to handle testing asynchronous functions in Jest, a crucial aspect in Node.js applications.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
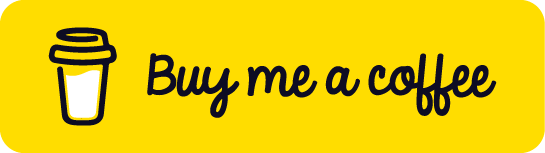
Chat with Chuck
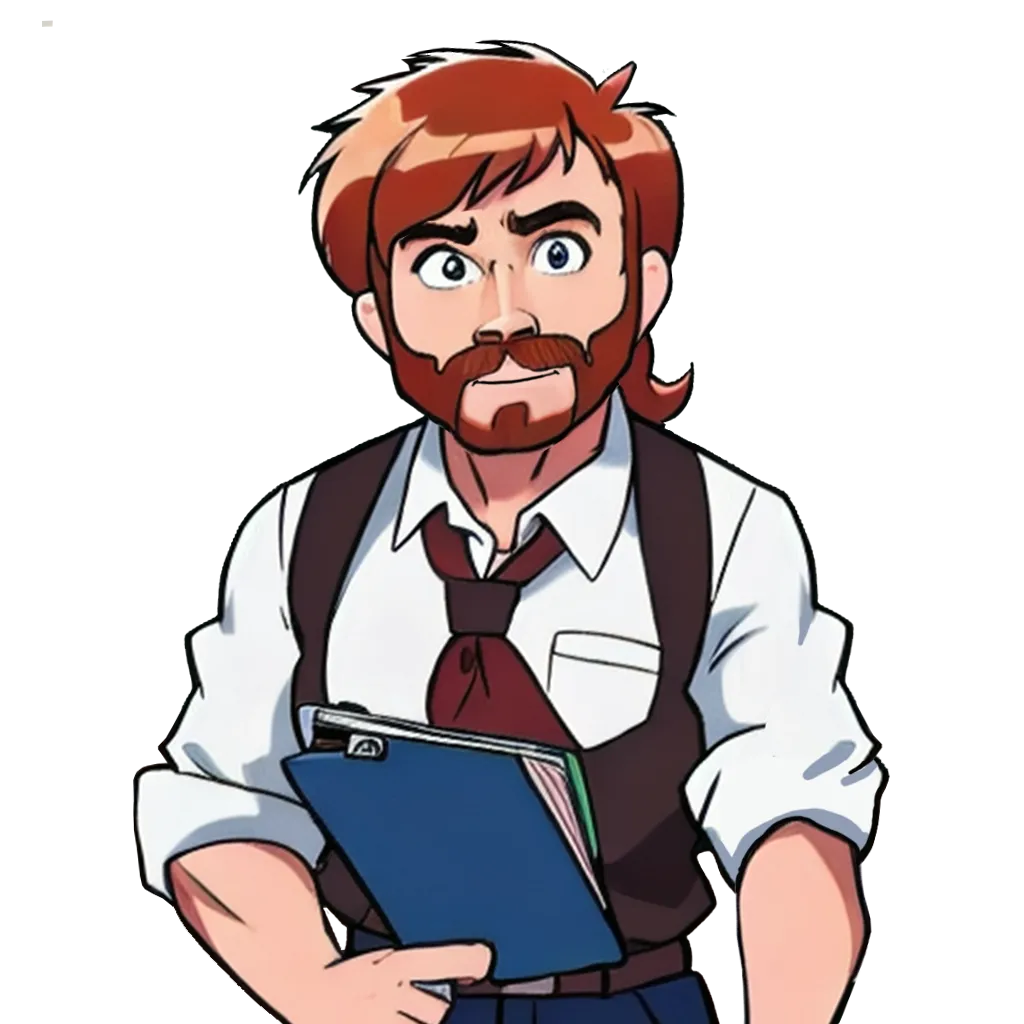
- Introduction to Testing in Node.js
- Installation and Configuration of Jest
- Basic Testing Concepts
- Structure of a Test with Jest
- Asserts and Matchers in Jest
- Test Driven Development (TDD) with Jest
- Mocks and Stubs in Jest
- Testing Asynchronous Functions
- Testing RESTful APIs
- Integration of Tests in the CI/CD Process
- Load and Performance Testing
- Best Practices in Testing
- Herramientas Complementarias para Testing
- Practical Examples and Use Cases
- Conclusions and Next Steps