Testing in Node.js with Jest
Test Driven Development (TDD) with Jest
Test Driven Development (TDD) is a software development methodology that emphasizes writing tests before implementing the code. The TDD cycle consists of three main steps: red (write a failing test), green (write the minimum code needed to pass the test), and refactor (optimize the code while keeping the tests green). In this section, you will learn how to apply TDD using Jest in a Node.js project.
Fundamentals of TDD
- Red: First write a test that fails because the functionality hasn't been implemented yet.
- Green: Implement the minimum functionality needed to make the test pass.
- Refactor: Optimize the code ensuring all tests continue to pass.
Advantages of TDD
- More reliable code: Tests ensure that any changes in the code do not break existing functionality.
- Improved design: Writing tests first forces you to think about the API and the code design from the user's perspective.
- Documentation: Tests serve as documentation that describes how the system is expected to work.
TDD Example with Jest
Imagine you want to write a sum
function that adds two numbers. Let's follow the TDD process to implement it.
Red Stage: Write a Failing Test
Write a test for the sum
function that you expect to fail because the function hasn't been implemented yet.
javascript
Run the test with:
bash
The test should fail because sum
is not defined correctly:
mobile-image:
desktop-image:
[Placeholder for image: Screenshot showing the failing test execution in the terminal.]
Green Stage: Implement the Functionality
Implement the minimum code needed to make the test pass.
javascript
Run the test again. This time, it should pass:
bash
mobile-image:
desktop-image:
[Placeholder for image: Screenshot showing the successful test execution in the terminal.]
Refactor Stage: Optimize the Code
Since the test has passed, you can refactor your code to improve its clarity or efficiency.
In this case, our sum
function is already quite simple, so there isn't much to refactor. But in more complex projects, this step might include reorganizing modules, removing redundant code, etc.
javascript
Complete Example: TDD Cycle for a subtract
Function
Let's follow the same process to write a subtract
function.
-
Red: Write the failing test
javascript -
Green: Implement the minimum code
javascript -
Refactor: Optimize the code
If necessary, refactor the code to improve its clarity or efficiency.
Practical Tips for TDD
- Start with small, simple tests.
- Ensure each new implementation passes all previous tests as well as the new ones.
- Don't move to the next step of the TDD cycle until the current test has passed.
- Regularly refactor to keep the code clean and manageable.
[Placeholder for image: Flowchart of the TDD cycle showing the steps Red, Green, and Refactor.]
Conclusion
TDD is a powerful methodology for improving the quality and maintainability of your code. By using Jest to apply TDD in Node.js, you can ensure that your code works as expected and is easy to maintain and extend. In the following chapters, we will explore how to work with mocks, stubs, and testing asynchronous functions in Jest.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
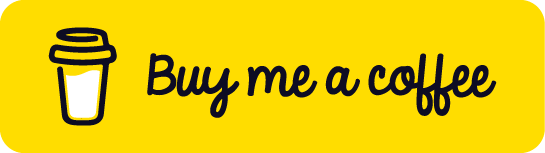
Chat with Chuck
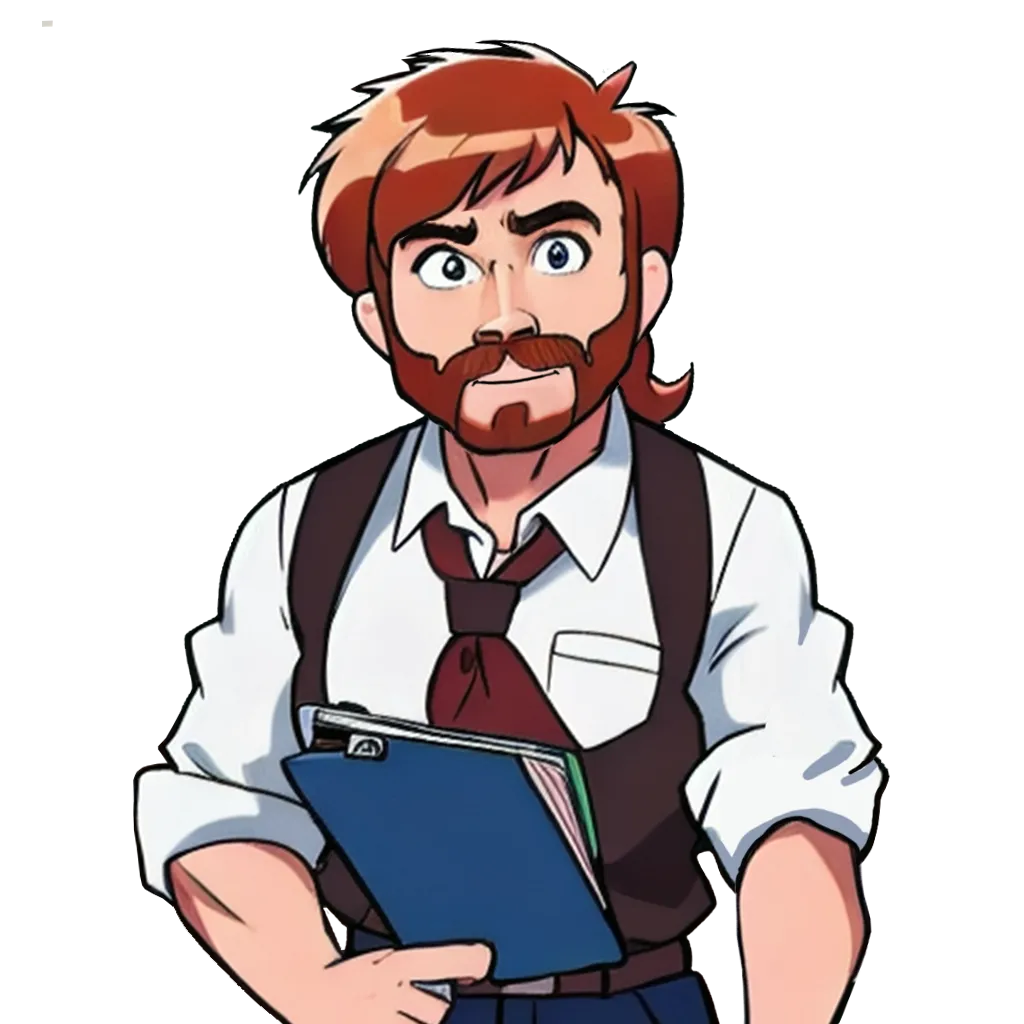
- Introduction to Testing in Node.js
- Installation and Configuration of Jest
- Basic Testing Concepts
- Structure of a Test with Jest
- Asserts and Matchers in Jest
- Test Driven Development (TDD) with Jest
- Mocks and Stubs in Jest
- Testing Asynchronous Functions
- Testing RESTful APIs
- Integration of Tests in the CI/CD Process
- Load and Performance Testing
- Best Practices in Testing
- Herramientas Complementarias para Testing
- Practical Examples and Use Cases
- Conclusions and Next Steps