Testing in Node.js with Jest
Practical Examples and Use Cases
In this section, we will consolidate everything learned through practical examples and typical use cases in Node.js application development. We will address common situations you will encounter and how to effectively apply testing techniques and tools.
Use Case 1: Authentication Module Testing
Suppose you have an authentication module responsible for registering users and authenticating sessions. Here, we will integrate Jest, Supertest, and Mocks to ensure that the critical functionalities of the authentication module work correctly.
Project Structure
Module Implementation
javascript
Authentication Module Tests
javascript
Use Case 2: Product API Testing
Suppose you have an API to manage products and want to ensure that CRUD operations work correctly.
Module Implementation
javascript
Product API Tests
javascript
[Placeholder for image: Diagram showing the interaction between user, server, and database in typical use case testing.]
Conclusion
These practical examples and use cases illustrate how you can apply the learned testing techniques to ensure the quality and functionality of various modules and APIs in your Node.js application. Adapting and extending these examples to your specific needs will strengthen your confidence in the code and improve the overall quality of the project.
In the next chapter, we will discuss the conclusions and next steps to continue improving your testing skills and strategies in Node.js.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
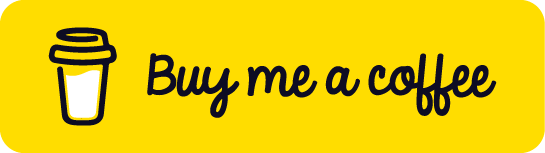
Chat with Chuck
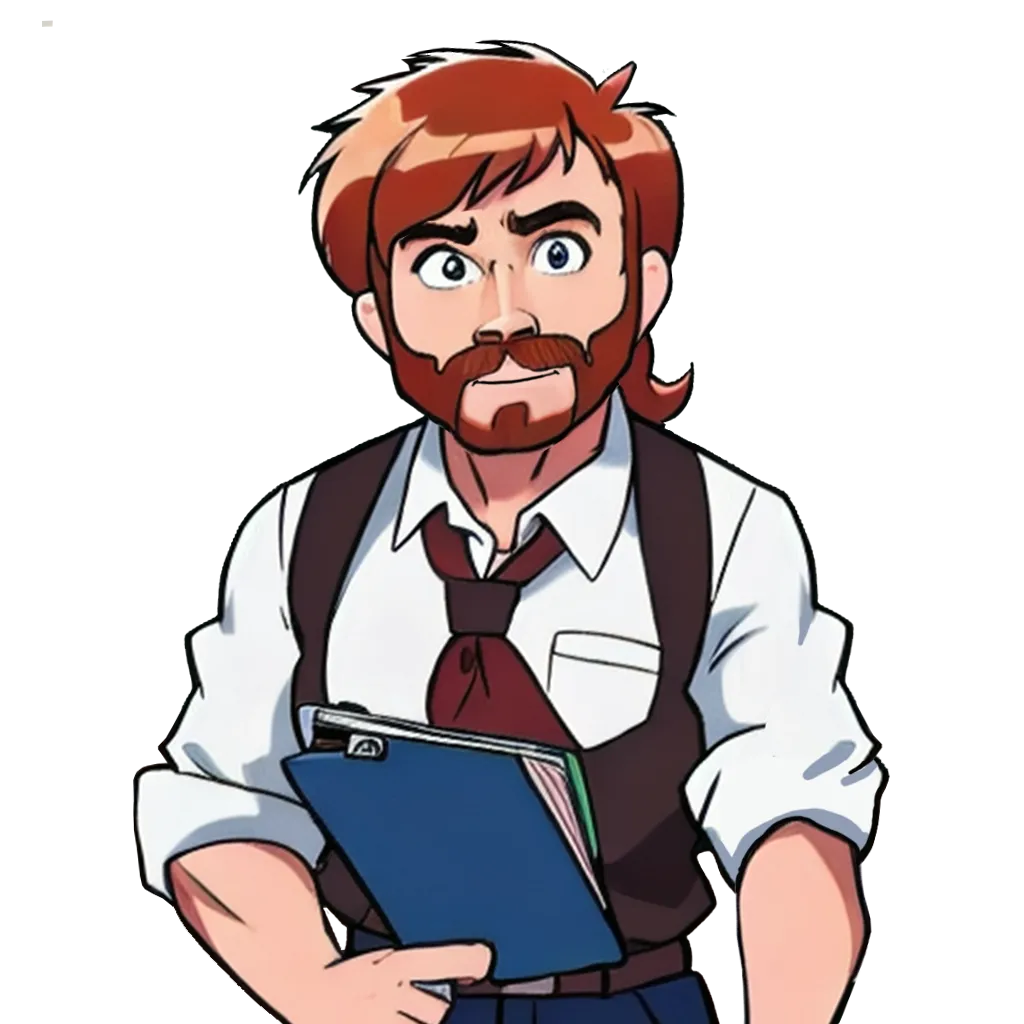
- Introduction to Testing in Node.js
- Installation and Configuration of Jest
- Basic Testing Concepts
- Structure of a Test with Jest
- Asserts and Matchers in Jest
- Test Driven Development (TDD) with Jest
- Mocks and Stubs in Jest
- Testing Asynchronous Functions
- Testing RESTful APIs
- Integration of Tests in the CI/CD Process
- Load and Performance Testing
- Best Practices in Testing
- Herramientas Complementarias para Testing
- Practical Examples and Use Cases
- Conclusions and Next Steps