Testing in Node.js with Jest
Introduction to Testing in Node.js
Testing is a crucial part of software development as it ensures that the code works as expected and helps identify errors before the software is released to production. In the context of Node.js, tests are essential due to the asynchronous nature of the environment and the server functionalities it supports.
What is Testing?
Testing is the process of evaluating and verifying that a program or application meets the specified requirements and functions correctly. Various types of tests can be conducted, such as:
- Unit tests: Evaluate small parts of code called units, usually functions or methods.
- Integration tests: Verify that different modules or services work correctly together.
- Functional tests: Ensure that the system meets the functional requirements.
- Load and performance tests: Evaluate how the system behaves under different workloads.
Importance of Testing in Node.js
Node.js, as a back-end platform, often handles critical operations and sensitive data. Untested code can lead to hard-to-track bugs and, in the worst case, security breaches. The benefits of performing tests in Node.js include:
- Increased code reliability: Detecting errors in the early stages of development.
- Ease of changes and refactors: Allows modifications in the code with confidence, knowing that tests will catch any possible failures.
- Implicit documentation: Tests can serve as documentation that explains how the code is expected to work.
- Improved code design: The process of writing tests encourages clean and maintainable code design.
Testing Tools in Node.js
Node.js has a wide variety of tools for testing. One of the most popular and robust is Jest. Jest is a JavaScript testing framework that offers an easy way to write, organize, and run tests. Some features of Jest include:
- Fast and efficient: Runs tests in parallel and performs test caching.
- Built-in Mocks and Stubs: Facilitates the simulation of dependencies and the capture of detailed behaviors.
- Code coverage: Generates detailed reports on the coverage of code tests.
Example of a Simple Test
Below is a simple test example using Jest. The general structure of a test in Jest includes descriptions (describe) and individual tests (test or it).
javascript
[Placeholder for image: Graphic example showing the flow of a unit test in Node.js with Jest, including the function to be tested, the test file, the expected result, and the test result.]
In the following sections of the course, we will delve into how to install and configure Jest, structure tests, use assertions and matchers, and many other advanced testing techniques for Node.js.
Let's start this adventure of ensuring the quality of your Node.js applications with Jest!
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
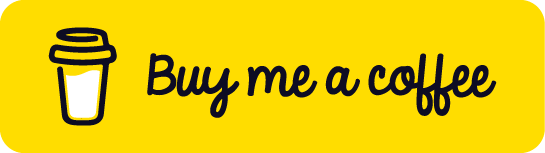
Chat with Chuck
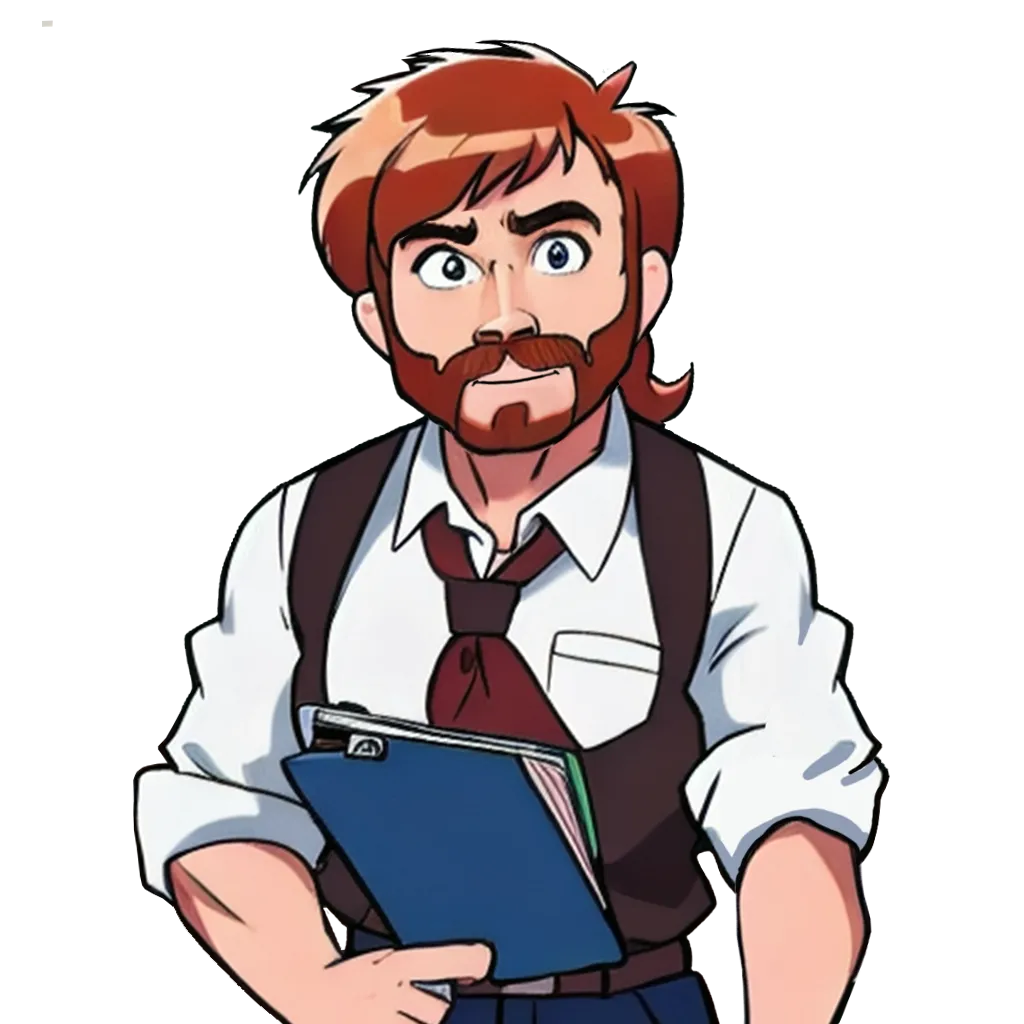
- Introduction to Testing in Node.js
- Installation and Configuration of Jest
- Basic Testing Concepts
- Structure of a Test with Jest
- Asserts and Matchers in Jest
- Test Driven Development (TDD) with Jest
- Mocks and Stubs in Jest
- Testing Asynchronous Functions
- Testing RESTful APIs
- Integration of Tests in the CI/CD Process
- Load and Performance Testing
- Best Practices in Testing
- Herramientas Complementarias para Testing
- Practical Examples and Use Cases
- Conclusions and Next Steps