Testing in Node.js with Jest
Load and Performance Testing
Load and performance testing are essential to ensure that your application can handle expected traffic and workloads without degrading its performance. This chapter covers how to perform load and performance tests on Node.js applications using tools like Artillery
and k6
.
What Are Load and Performance Tests?
-
Load Tests: Assess how the system behaves under a specific number of requests or users. The goal is to identify the point at which the application starts to show performance issues.
-
Performance Tests: Measure response time and system efficiency under different conditions. They focus on verifying whether it meets the expected performance requirements.
Popular Tools for Load and Performance Testing
Several tools can help you perform load and performance tests. Among the most popular are Artillery
and k6
.
Installing Artillery
Artillery is a modern and powerful tool for load and performance testing of applications.
sh
Installing k6
k6 is another excellent option, focused on simplicity and performance.
sh
Configuring and Using Artillery
Artillery Configuration File
Create a load-test.yml
configuration file to define the test scenario.
yaml
Running Tests with Artillery
Run the load test with:
sh
Configuring and Using k6
k6 Configuration File
Create a load-test.js
script file to define the test scenario.
javascript
Running Tests with k6
Run the load test script with:
sh
Result Analysis
Interpreting the results of load and performance tests is vital to understand how your application handles the load. Key metrics include:
- TPS (Transactions per Second): Number of requests handled per second.
- Response Time: Latency from when the request is sent to when the response is received.
- Errors: Rate of failed requests.
- Resource Usage: CPU, memory, and network consumption during the test.
Complete Example: Load Testing with Artillery
Below is a complete example using Artillery to test a RESTful API:
yaml
Run the test with:
sh
[Placeholder for image: Graph showing load test results with response time and error rate curves as a function of the number of users.]
Conclusion
Load and performance testing are a fundamental part of developing scalable and robust applications. Using tools like Artillery and k6, you can identify bottlenecks and ensure that your application can handle expected traffic and workloads. In the next chapter, we will cover best practices in testing, providing techniques and tips to keep your test suite efficient and manageable.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
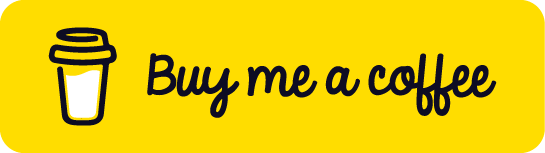
Chat with Chuck
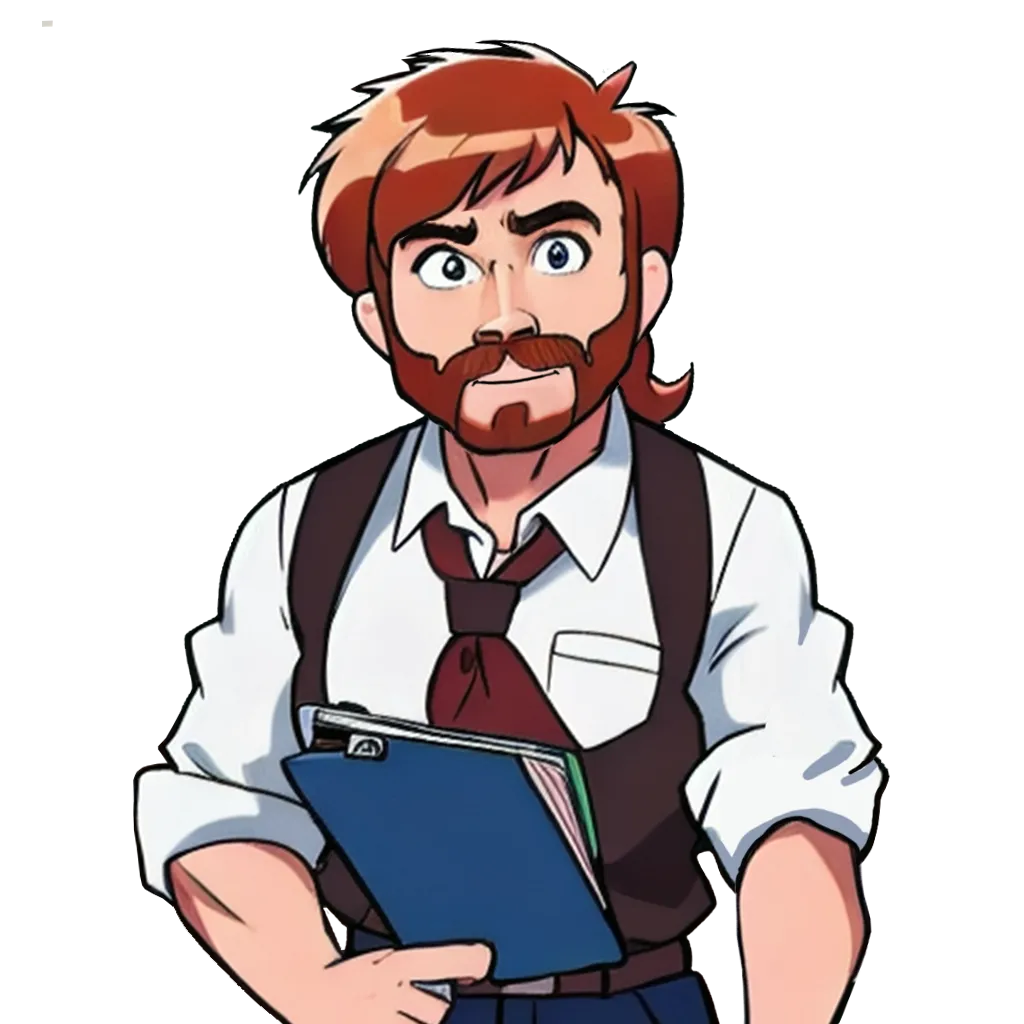
- Introduction to Testing in Node.js
- Installation and Configuration of Jest
- Basic Testing Concepts
- Structure of a Test with Jest
- Asserts and Matchers in Jest
- Test Driven Development (TDD) with Jest
- Mocks and Stubs in Jest
- Testing Asynchronous Functions
- Testing RESTful APIs
- Integration of Tests in the CI/CD Process
- Load and Performance Testing
- Best Practices in Testing
- Herramientas Complementarias para Testing
- Practical Examples and Use Cases
- Conclusions and Next Steps