Testing in Node.js with Jest
Testing RESTful APIs
In this section, we will learn how to test RESTful APIs in Node.js using Jest. Testing APIs is crucial to ensure that our endpoints work correctly and handle requests and responses as expected. We will use supertest
, a popular tool for making HTTP requests in our tests.
Setting Up the Environment
First, make sure you have a basic Express server configured. Here is a simple example:
javascript
Installing Supertest
Install supertest
as a development dependency:
bash
Configuring Test for RESTful API
We will use Jest and Supertest to make requests to our API and verify the responses.
GET Endpoint Test
javascript
POST Endpoint Test
javascript
Handling Errors
It is important to also test how our API handles errors and invalid requests.
javascript
Authentication and Authorization
If your API requires authentication, you can include tokens or credentials in your request.
javascript
Complete Example: Testing RESTful API
Below is a complete example of testing a RESTful API using Jest and Supertest:
javascript
[Placeholder for image: Diagram showing the flow of an HTTP request from the client to the server and vice versa, with a focus on the testing and validation process of responses.]
Conclusion
Testing RESTful APIs is essential to ensure that your endpoints work correctly and handle requests and responses appropriately. Using Jest along with Supertest makes this process easier. In the next chapter, we will address integrating tests into the CI/CD flow, ensuring that your tests run automatically as part of the development process.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
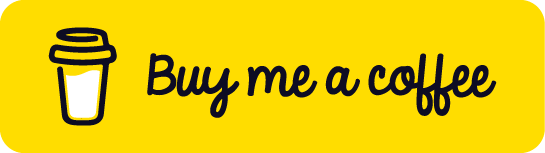
Chat with Chuck
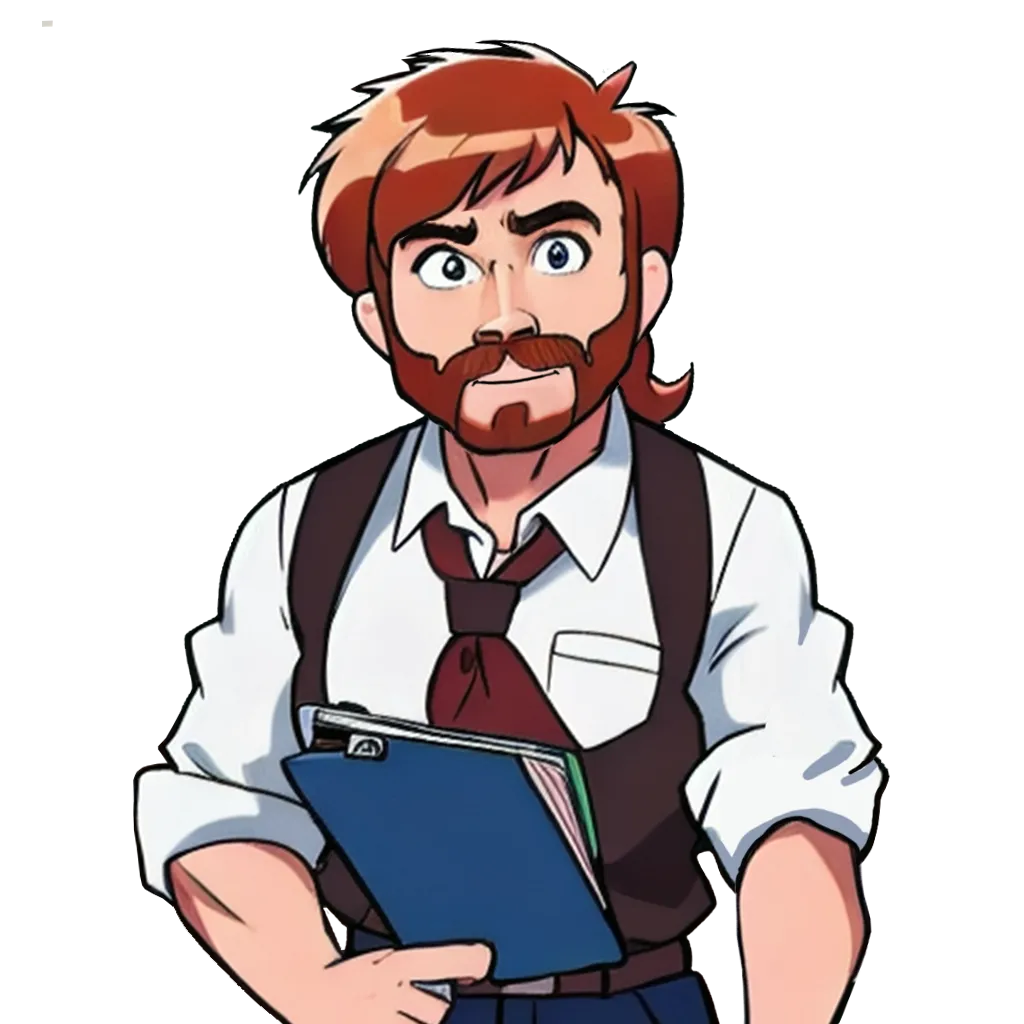
- Introduction to Testing in Node.js
- Installation and Configuration of Jest
- Basic Testing Concepts
- Structure of a Test with Jest
- Asserts and Matchers in Jest
- Test Driven Development (TDD) with Jest
- Mocks and Stubs in Jest
- Testing Asynchronous Functions
- Testing RESTful APIs
- Integration of Tests in the CI/CD Process
- Load and Performance Testing
- Best Practices in Testing
- Herramientas Complementarias para Testing
- Practical Examples and Use Cases
- Conclusions and Next Steps