Testing in Node.js with Jest
Structure of a Test with Jest
In this section, you will learn how to structure your tests using Jest so that they are clear, maintainable, and effective. Jest provides a simple yet powerful structure for organizing your tests, making use of describe
blocks, individual tests (test
or it
), and assertions (expect
).
Organizing Tests with describe
The describe
block allows you to group related tests under a common context. This makes your test suite more organized and easier to read.
javascript
Writing Tests with test
or it
Within a describe
block, we use test
or it
to define individual tests. These methods are interchangeable, and the choice between them is a matter of personal or team style preference.
javascript
Assertions with expect
Assertions are the core of tests in Jest. We use expect
to evaluate the result of an expression or function and verify if it meets a specific condition.
javascript
Test Lifecycle
Jest allows defining hooks to set up and tear down the environment before and after tests. The most common hooks are:
beforeAll
: Runs once before all tests in the block.afterAll
: Runs once after all tests in the block.beforeEach
: Runs before each test in the block.afterEach
: Runs after each test in the block.
javascript
Complete Example
Below is an example that uses all the mentioned concepts:
javascript
[Placeholder for image: Diagram showing the structure of a test in Jest with describe blocks, it/test, beforeAll/afterAll/ beforeEach/afterEach hooks, and expect assertions.]
Conclusion
The key to writing good tests is organization and clarity. Using describe
, test
or it
, and expect
effectively will help you keep your tests readable and easy to understand. In the next section, we will explore more details on how to use assertions and matchers in Jest.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
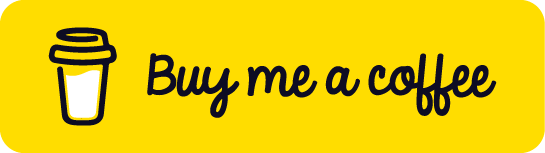
Chat with Chuck
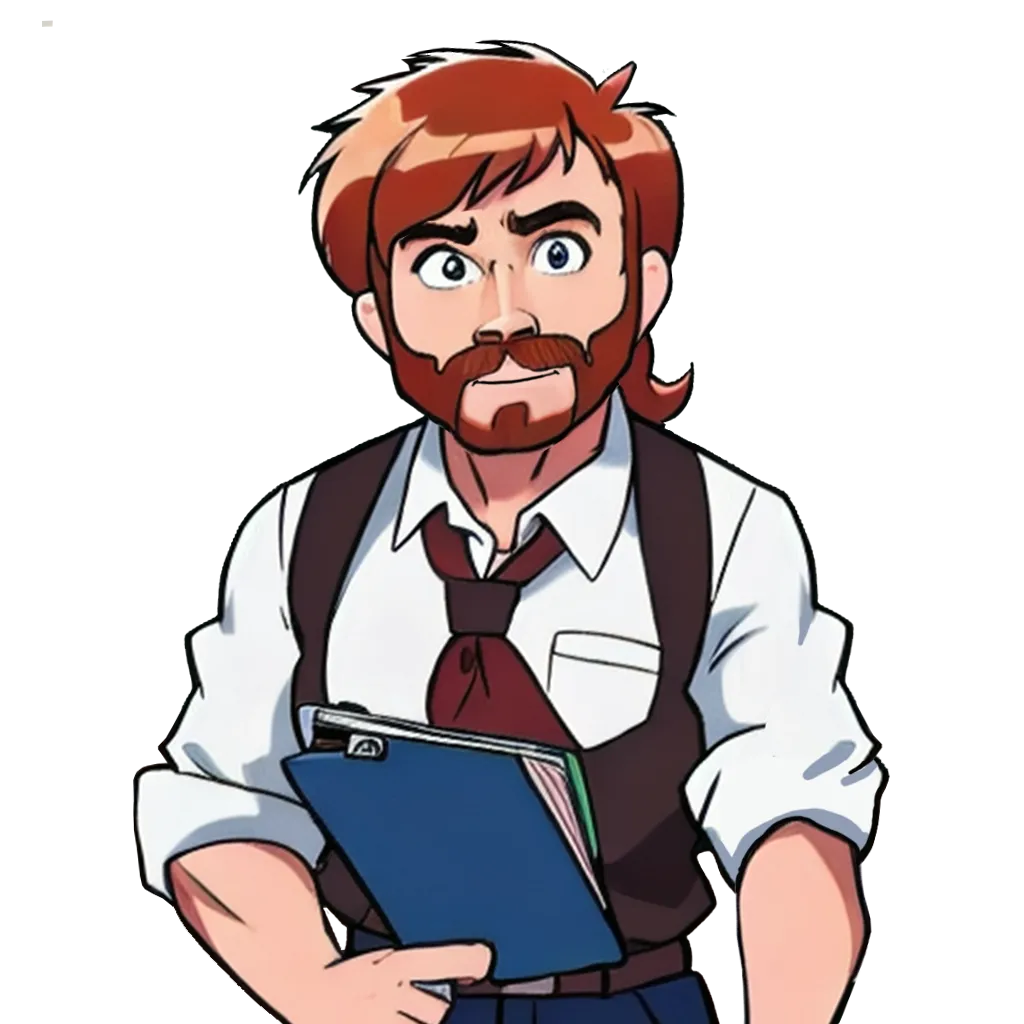
- Introduction to Testing in Node.js
- Installation and Configuration of Jest
- Basic Testing Concepts
- Structure of a Test with Jest
- Asserts and Matchers in Jest
- Test Driven Development (TDD) with Jest
- Mocks and Stubs in Jest
- Testing Asynchronous Functions
- Testing RESTful APIs
- Integration of Tests in the CI/CD Process
- Load and Performance Testing
- Best Practices in Testing
- Herramientas Complementarias para Testing
- Practical Examples and Use Cases
- Conclusions and Next Steps