Testing in Node.js with Jest
Installation and Configuration of Jest
In this section, we will focus on how to install and configure Jest to use it in a Node.js project. Jest is a powerful and comprehensive library for testing in JavaScript that simplifies many common tasks.
Prerequisites
To follow this chapter, make sure you have Node.js and npm (Node Package Manager) installed on your system. You can download Node.js from nodejs.org.
Installing Jest
You can install Jest globally or locally in your project. However, it is a recommended practice to install it locally so that each project can have its own version of Jest.
-
Initialize your project with npm: If you have not yet created a Node.js project, you can do so with the following command:
bash -
Install Jest as a development dependency:
bash
Configuring Jest
After installing Jest, there are various ways to configure it, from the npm command line, via the package.json file, or using a dedicated configuration file.
Configuration via package.json
-
Add a test script in package.json: Inside your
package.json
file, add the following script under the "scripts" section:jsonNow you can run your tests by running:
bash
Configuration via jest.config.js file
-
Create a configuration file: You can create a
jest.config.js
file in the root of your project to customize Jest configuration:javascript -
Common configuration options:
- verbose: If set to
true
, Jest will provide detailed information about each test. - testEnvironment: Specifies the environment for the tests. By default, it is "jsdom" but can be changed to "node" for Node.js applications.
- moduleNameMapper: Allows specifying paths that Jest should consider when importing modules.
- setupFilesAfterEnv: Specifies an array of scripts that will run before each test suite.
- verbose: If set to
Example of Complete Configuration
Below is an example of how your complete jest.config.js
file might look:
javascript
Running Tests with Jest
Once you have configured Jest, you can start writing and running your tests. You can organize your tests inside a tests
folder or by naming your test files with the convention .test.js
or .spec.js
.
To run your tests, simply use:
bash
[Placeholder for image: Command line screen showing the execution of tests in Jest, including an example output with passed and failed tests.]
Now that you have Jest installed and configured, you are ready to start writing and running tests. In the upcoming sections, we will explore in detail how to structure a test in Jest and the various functionalities that Jest provides for testing in Node.js.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
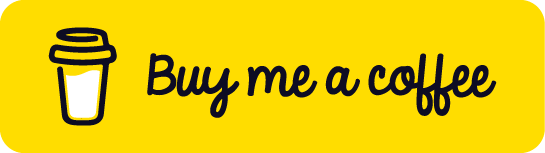
Chat with Chuck
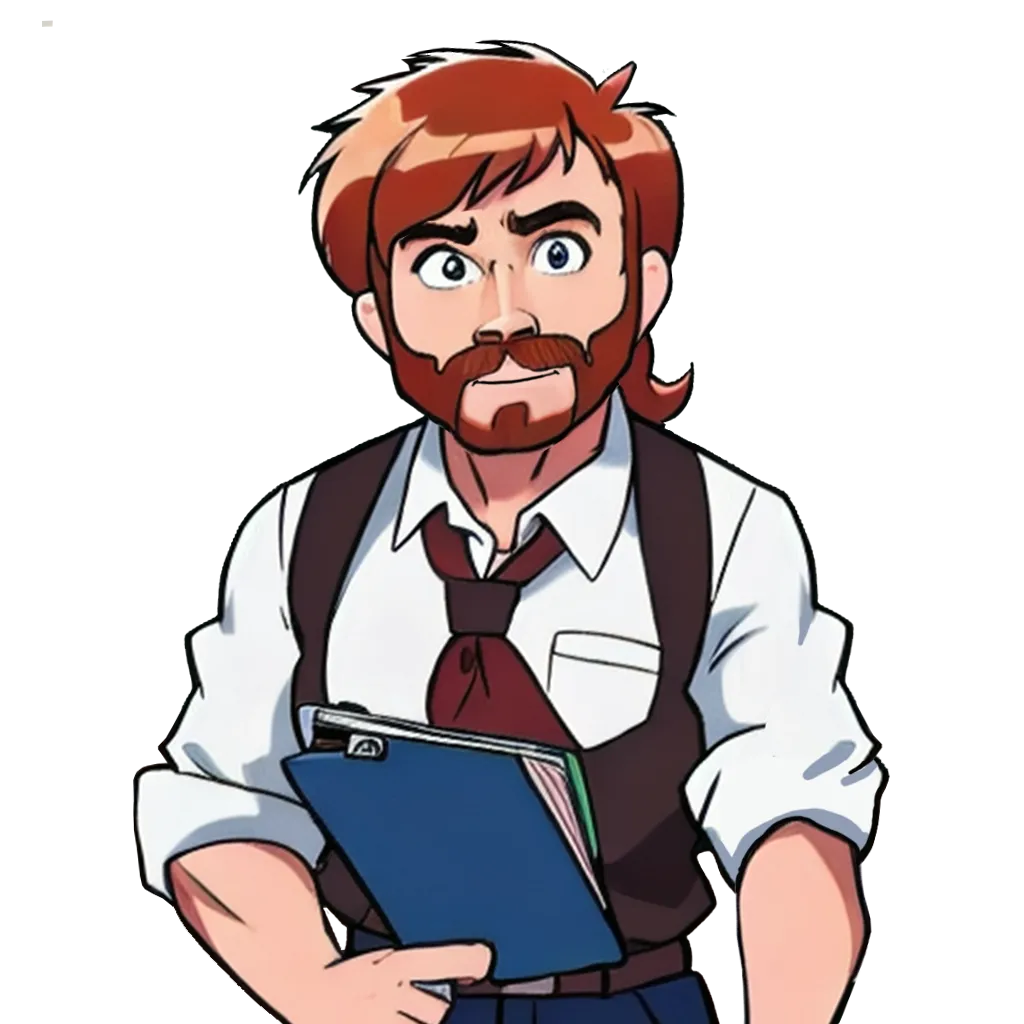
- Introduction to Testing in Node.js
- Installation and Configuration of Jest
- Basic Testing Concepts
- Structure of a Test with Jest
- Asserts and Matchers in Jest
- Test Driven Development (TDD) with Jest
- Mocks and Stubs in Jest
- Testing Asynchronous Functions
- Testing RESTful APIs
- Integration of Tests in the CI/CD Process
- Load and Performance Testing
- Best Practices in Testing
- Herramientas Complementarias para Testing
- Practical Examples and Use Cases
- Conclusions and Next Steps