Testing in Node.js with Jest
Integration of Tests in the CI/CD Process
Continuous Integration/Continuous Deployment (CI/CD) is an essential practice in modern software development. Integrating automated tests into the CI/CD flow ensures that code is continuously tested at different stages of development, guaranteeing quality and stability. In this chapter, you will learn how to integrate Jest tests into your CI/CD pipeline.
What is CI/CD?
- Continuous Integration (CI): The practice of integrating code changes into a shared repository several times a day, followed by the automation of builds and tests.
- Continuous Deployment (CD): The practice of automatically deploying every change that passes CI tests to the production environment.
Popular CI/CD Tools
There are several tools for setting up CI/CD pipelines, including:
- GitHub Actions
- GitLab CI
- Travis CI
- Jenkins
- CircleCI
In this chapter, we will focus on how to set up Jest in GitHub Actions and GitLab CI.
GitHub Actions
GitHub Actions provides an easy and powerful way to automate workflows directly from your GitHub repository.
Setting up GitHub Actions
-
Create a workflow file: In your repository, create a file at
.github/workflows/ci.yml
. -
Define the workflow: Here is an example GitHub Actions configuration to run Jest tests:
yaml
GitLab CI
GitLab CI is an integrated and fully free solution for continuous integration of Git repositories.
Setting up GitLab CI
-
Create a
.gitlab-ci.yml
file: At the root of your repository, create a file named.gitlab-ci.yml
. -
Define the pipeline: Here is an example GitLab CI configuration to run Jest tests:
yaml
Code Coverage Integration
Having visibility into code coverage is crucial in a CI/CD environment. Jest makes it easy to generate coverage reports.
Setting Up Code Coverage
-
Update Jest configuration: Ensure that Jest is configured to generate code coverage reports. Add or update your
jest.config.js
file with the following configuration:javascript -
Add coverage support in your workflow (optional): You can upload coverage reports using specific integrations of each CI/CD tool or tools like Coveralls or Codecov.
yaml
Complete Example: Integration with GitHub Actions
Here is a complete example of GitHub Actions configuration with code coverage integration:
yaml
[Placeholder for image: Flowchart showing a CI/CD pipeline with steps for repository checkout, dependency installation, test execution, and coverage report generation.]
Conclusion
Integrating Jest into your CI/CD flow is a crucial step to ensure the continuous quality of your code. Using tools like GitHub Actions or GitLab CI, you can automate testing, ensure that your code passes all tests before deployment, and monitor code coverage. In the following chapters, we will explore load and performance testing, as well as best practices in testing.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
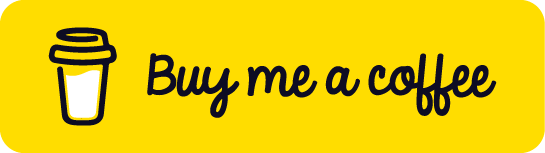
Chat with Chuck
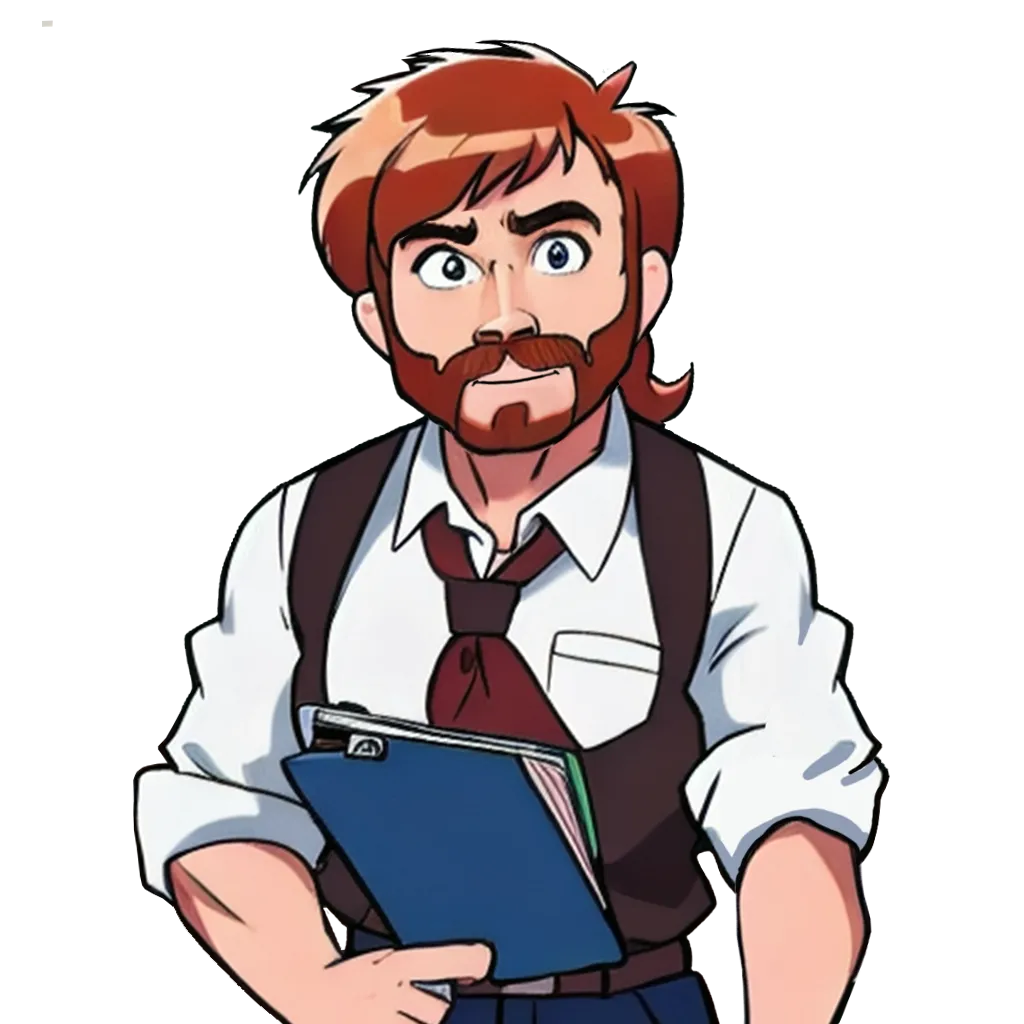
- Introduction to Testing in Node.js
- Installation and Configuration of Jest
- Basic Testing Concepts
- Structure of a Test with Jest
- Asserts and Matchers in Jest
- Test Driven Development (TDD) with Jest
- Mocks and Stubs in Jest
- Testing Asynchronous Functions
- Testing RESTful APIs
- Integration of Tests in the CI/CD Process
- Load and Performance Testing
- Best Practices in Testing
- Herramientas Complementarias para Testing
- Practical Examples and Use Cases
- Conclusions and Next Steps