Testing in Node.js with Jest
Testing Asynchronous Functions
Testing asynchronous functions is crucial in Node.js applications, where asynchronous operations, such as database and API calls, are common. Jest provides various tools to effectively handle asynchronous tests. This chapter will guide you through the essential techniques for testing asynchronous functions.
Asynchronous Testing Methods in Jest
There are several ways to handle asynchronous functions in Jest:
- Callbacks: Use callbacks to test asynchronous functions.
- Promises: Work with promises for cleaner and more manageable tests.
- async/await: A more modern and clearer syntax for handling asynchronous operations.
Using Callbacks
To test functions that use callbacks, Jest accepts a done
parameter. Call done
when the asynchronous operation has completed.
javascript
Using Promises
Jest can handle promises directly. Return a promise in your test, and Jest will wait for it to resolve.
javascript
Using .resolves
and .rejects
Jest provides useful methods like .resolves
and .rejects
to work with promises more readably.
javascript
Using async/await
The async/await
syntax is more modern and makes asynchronous code easier to read and write.
javascript
Complete Example: Asynchronous Testing in Action
Let's implement a function that fetches data from a simulated API and test various aspects of its asynchronous behavior.
javascript
[Placeholder for image: Flowchart showing the process of testing asynchronous functions with callbacks, promises, and async/await.]
Conclusion
Testing asynchronous functions is essential to ensure that your Node.js application handles asynchronous operations correctly. The tools provided by Jest make this process simpler and more efficient. In the following chapters, we will explore how to test RESTful APIs and how to integrate your tests into the CI/CD workflow.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
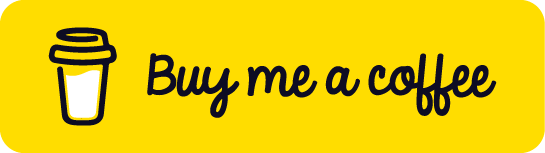
Chat with Chuck
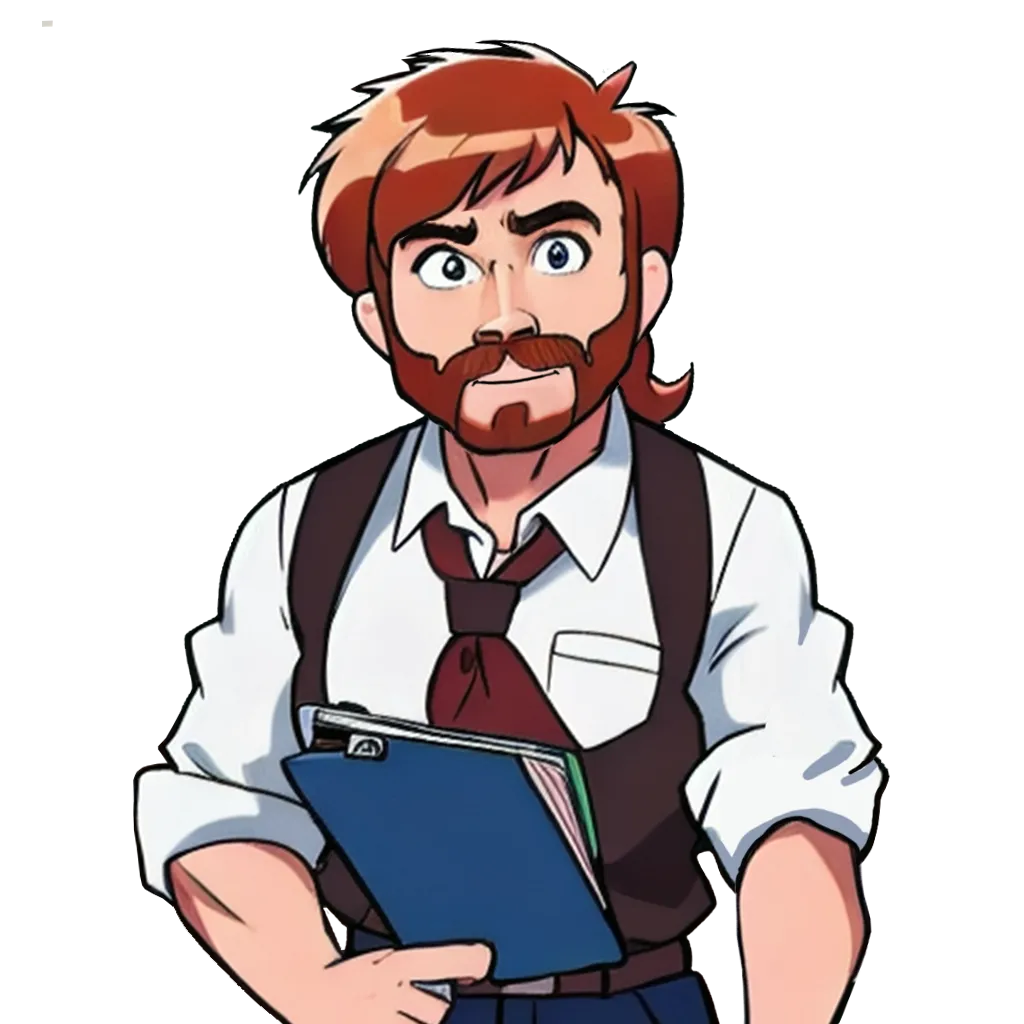
- Introduction to Testing in Node.js
- Installation and Configuration of Jest
- Basic Testing Concepts
- Structure of a Test with Jest
- Asserts and Matchers in Jest
- Test Driven Development (TDD) with Jest
- Mocks and Stubs in Jest
- Testing Asynchronous Functions
- Testing RESTful APIs
- Integration of Tests in the CI/CD Process
- Load and Performance Testing
- Best Practices in Testing
- Herramientas Complementarias para Testing
- Practical Examples and Use Cases
- Conclusions and Next Steps