Testing in Node.js with Jest
Best Practices in Testing
To maintain an efficient, manageable, and high-quality test suite, it is important to follow certain recommended practices. These practices not only help keep your code organized but also ensure that your tests are effective and reliable.
1. Write Simple and Isolated Tests
Tests should be simple and focus on one single responsibility or feature of the code. Ensure that each test runs in isolation and does not depend on other tests.
javascript
2. Use Descriptive Names
Test names should be clear and descriptive. This makes it easier to identify issues when a test fails and improves code readability.
javascript
3. Set Up and Clean Up the Environment
Use Jest's beforeAll
, afterAll
, beforeEach
, and afterEach
hooks to set up and clean up the testing environment. This ensures that each test starts with a clean and predictable state.
javascript
4. Mocking Dependencies
Mocking dependencies helps isolate the unit of code you are testing, eliminating side effects and increasing the reliability of tests.
javascript
5. Perform Coverage Testing
Test coverage is a metric that indicates which parts of the code are executed by the tests. Although high coverage does not guarantee the absence of bugs, it helps identify parts of the code that have not been tested.
sh
Configure Jest to show test coverage by editing jest.config.js
:
javascript
6. Keep Tests Fast
Tests should be fast enough to run frequently. If tests are slow, they are less likely to be run regularly. Optimize tests by minimizing I/O usage and running them in parallel.
javascript
7. Tests in Different Environments
Ensure that tests run in different development and CI/CD environments to identify any inconsistencies.
8. Reuse Common Code
Create utility functions to set up, clean, and check common states, reducing code duplication in your tests.
javascript
9. Review and Refactor Tests Regularly
Just like production code, tests should also be reviewed and refactored regularly to keep them clean and manageable.
10. Document Tests
Document test cases and any special setup required. This is especially useful for new team members who need to understand how and what is being tested.
Combined Example
Below is an example that combines several best practices:
javascript
[Placeholder for image: Diagram visualizing best practices, including test setup and cleanup, mocking dependencies, and coverage analysis.]
Conclusion
Following these best practices in testing not only improves code quality but also makes it easier to maintain and expand your test suite. In the upcoming chapters, we will explore complementary tools that can further enhance your testing workflow.
- Introduction to Testing in Node.js
- Installation and Configuration of Jest
- Basic Testing Concepts
- Structure of a Test with Jest
- Asserts and Matchers in Jest
- Test Driven Development (TDD) with Jest
- Mocks and Stubs in Jest
- Testing Asynchronous Functions
- Testing RESTful APIs
- Integration of Tests in the CI/CD Process
- Load and Performance Testing
- Best Practices in Testing
- Herramientas Complementarias para Testing
- Practical Examples and Use Cases
- Conclusions and Next Steps
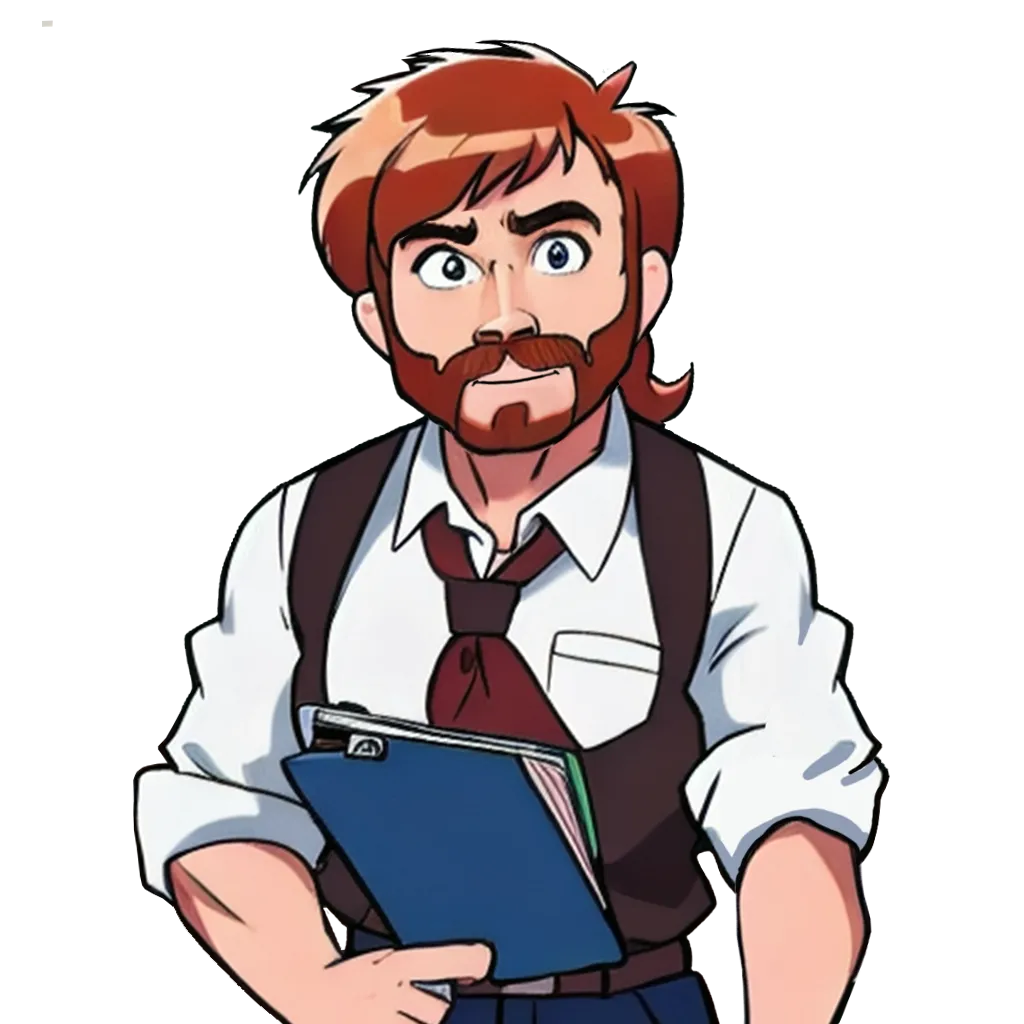