Design Patterns in JavaScript
Command Pattern
The Command pattern is a behavioral design pattern that turns requests or operations into objects. This pattern allows requests to be parameterized, queued, logged, and undone, providing greater flexibility and decoupling in executing operations.
Features of the Command Pattern
- Encapsulation: Requests are encapsulated as independent objects.
- Decoupling: Decouples the object that invokes the operation from the object that performs it.
- Flexibility: Allows enqueuing, storing, and undoing operations in a flexible manner.
Benefits of the Command Pattern
- Reusability: Operations encapsulated as objects can be reused in different contexts.
- Maintainability: Facilitates the addition and modification of commands without affecting existing code.
- Undo and Redo: Facilitates the implementation of undo and redo functionality for operations.
Implementation of the Command Pattern in JavaScript
Below are examples of how to implement the Command pattern using modern ES6 syntax.
Example 1: Basic Implementation of the Command Pattern
javascript
In this example, the LightOnCommand
and LightOffCommand
encapsulate the actions of turning the light on and off. The RemoteControl
acts as an invoker that executes and undoes these commands.
Example 2: Chaining Commands
This example demonstrates how multiple commands can be chained.
javascript
In this example, MacroCommand
groups several commands and allows them to be executed or undone in sequence.
Use Cases of the Command Pattern
The Command pattern is useful in situations where:
- Operation history: A history of operations needs to be maintained to support undo/redo.
- Parameterized operations: Operations need to be parameterized and executed in different contexts.
- Decoupled invocation: The invocation of operations needs to be decoupled from their implementation.
Considerations and Best Practices
- Proper granularity: Define commands with appropriate granularity to avoid complicating the management of operations.
- Exception handling: Implement proper exception handling within commands to avoid cascading failures.
- Document intentions: Clearly document the intentions and effects of each command to facilitate its maintenance and usage.
In the next chapter, we will explore the Strategy Pattern and how it can be used to define a family of algorithms and make them interchangeable.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
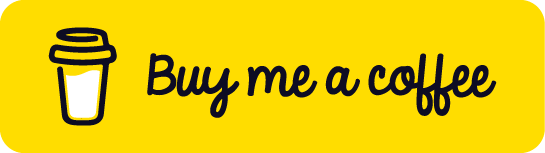
Chat with Chuck
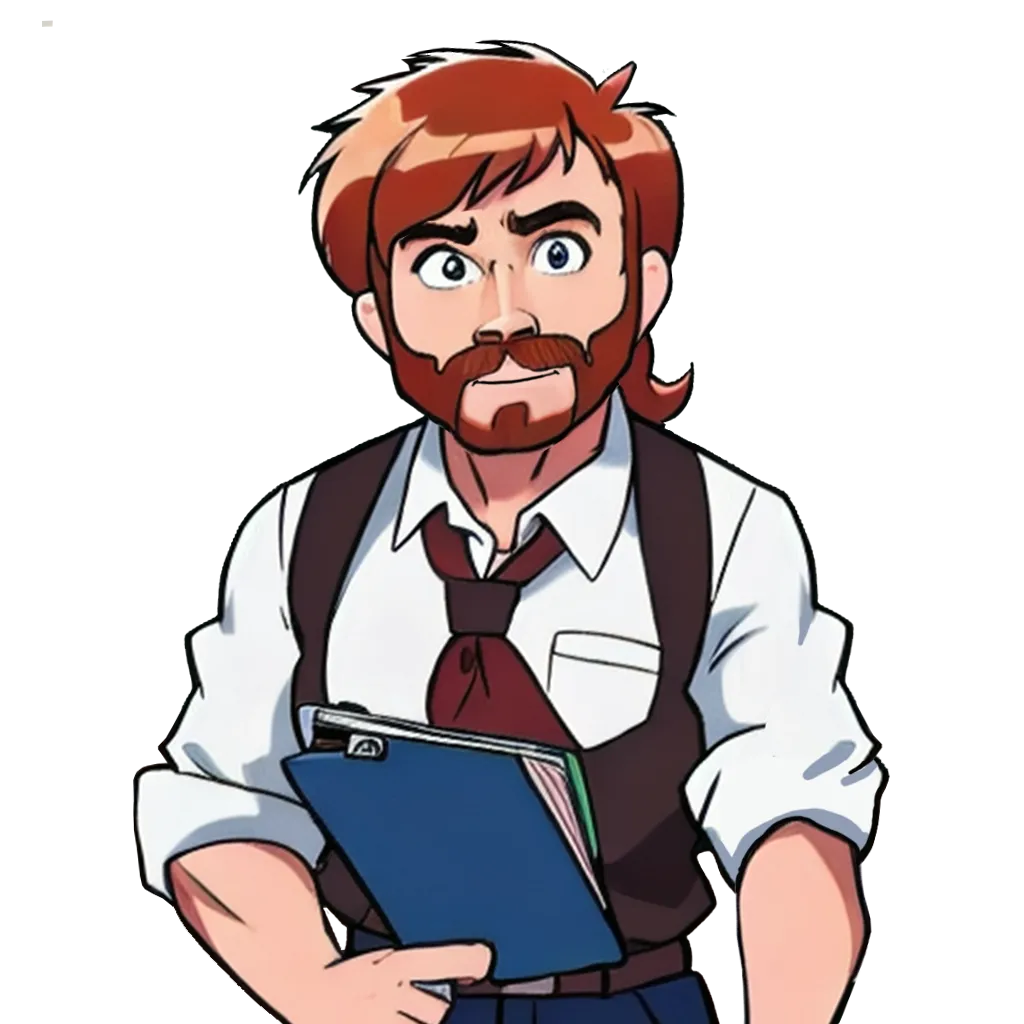
- Introduction to Design Patterns
- JavaScript and ES6 Fundamentals
- Singleton Pattern
- Factory Pattern
- Patrón Prototype
- Observer Pattern
- Module Pattern
- Revealing Module Pattern
- Mediator Pattern
- Decorator Pattern
- Command Pattern
- Strategy Pattern
- Template Pattern
- State Pattern
- Conclusions and Best Practices in Design Patterns