Design Patterns in JavaScript
Introduction to Design Patterns
Design patterns are reusable solutions to common problems encountered in software development. These patterns are not specific pieces of code but rather templates on how to solve problems that can arise in different situations. The main idea behind design patterns is to create a standard for addressing recurring problems, facilitating collaboration and communication among developers.
Why use design patterns?
- Efficiency: They help save time and effort by providing already tested and optimized solutions.
- Ease of maintenance: They facilitate code comprehension by following recognized and documented patterns.
- Flexibility and scalability: They enable the creation of more robust and scalable applications.
- Improves communication: They help development teams speak a common language.
Categories of Design Patterns
Design patterns are generally divided into three categories:
- Creational Patterns: Address problems related to object creation. Examples include Singleton, Factory, and Prototype.
- Structural Patterns: Deal with the composition of classes and objects. Examples include Adapter, Composite, and Decorator.
- Behavioral Patterns: Focus on interaction and responsibility between objects. Examples include Observer, Mediator, and Strategy.
History of Design Patterns
The idea of design patterns was popularized by the book "Design Patterns: Elements of Reusable Object-Oriented Software", written by Erich Gamma, Richard Helm, Ralph Johnson, and John Vlissides, also known as the "Gang of Four" or GoF. This book introduced 23 fundamental design patterns that are still widely used today.
Application of Design Patterns in JavaScript
JavaScript, being a flexible and prototype-based programming language, allows the implementation of design patterns quite efficiently. Using modern ECMAScript (ES6) features, design patterns in JavaScript can be implemented more concisely and clearly. In this course, we will explore how to implement these patterns using the latest features of ES6 and beyond.
Practical Example: Problem and Solution
Suppose we are developing an e-commerce application and need to handle the creation of different types of users (Customers, Administrators, etc.). A naive approach might be to duplicate code in multiple places. This is where a design pattern comes into play to solve the problem.
Problem (Without Design Pattern):
javascript
Solution (Using the Factory Pattern, which we will discuss in detail later):
javascript
In the following chapters, we will delve deeper into each of the mentioned design patterns.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
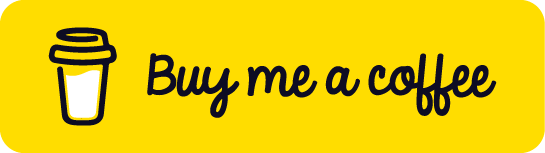
Chat with Chuck
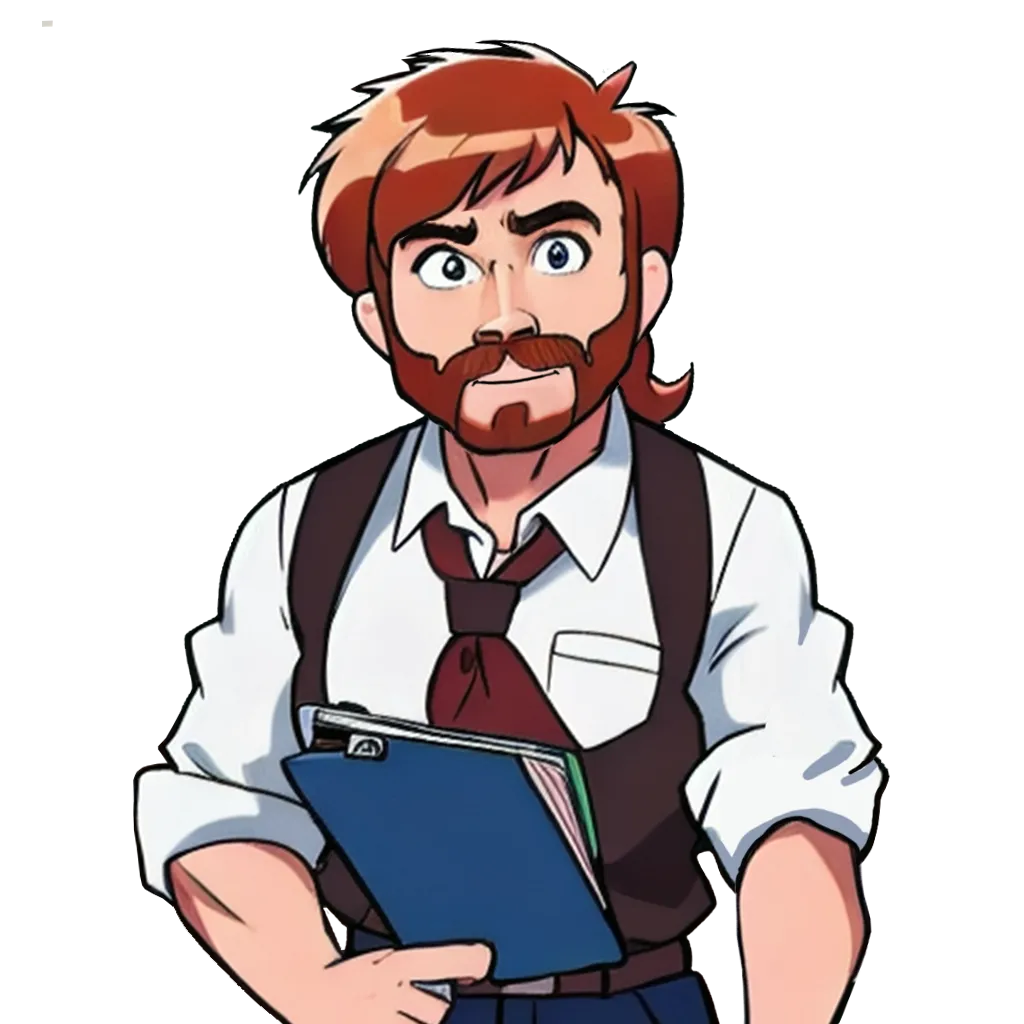
- Introduction to Design Patterns
- JavaScript and ES6 Fundamentals
- Singleton Pattern
- Factory Pattern
- Patrón Prototype
- Observer Pattern
- Module Pattern
- Revealing Module Pattern
- Mediator Pattern
- Decorator Pattern
- Command Pattern
- Strategy Pattern
- Template Pattern
- State Pattern
- Conclusions and Best Practices in Design Patterns