Design Patterns in JavaScript
Strategy Pattern
The Strategy Pattern is a behavioral design pattern that allows defining a family of algorithms, encapsulating each one of them, and making them interchangeable. Thanks to this pattern, the chosen algorithm can vary independently of the context in which it is used.
Characteristics of the Strategy Pattern
- Interchangeability: Algorithms can be easily replaced without modifying the code that uses them.
- Encapsulation: Each algorithm is encapsulated in its own class.
- Decoupling: Separates the behavior and makes it independent of the context in which it is executed.
Benefits of the Strategy Pattern
- Flexibility: Allows dynamically changing the algorithm used at runtime.
- Reusability: Encourages the reusability of algorithms in different contexts and applications.
- Maintenance: Facilitates the maintenance and extension of code by decoupling the algorithms from the context.
Strategy Pattern Implementation in JavaScript
Below are examples of how to implement the Strategy Pattern using modern ES6 syntax.
Example 1: Payment Processing Strategies
javascript
In this example, PaymentProcessor
uses different payment strategies (PayPalPayment
, CreditCardPayment
, BitcoinPayment
) to process payments.
Example 2: Sorting Strategy
javascript
In this example, SortingStrategy
uses different sorting strategies (AscendingSort
, DescendingSort
) to sort a list of data.
Use Cases of the Strategy Pattern
The Strategy Pattern is useful in situations where:
- Interchangeable algorithms: There are multiple ways to perform an operation and one needs to be selected at runtime.
- Decoupling algorithms: You want to keep the algorithms separate and not coupled to the context.
- Modifying behavior: You need to change the behavior of an object without modifying its class.
Considerations and Best Practices
- Consistent interfaces: Ensure all strategies implement a consistent interface to facilitate interchangeability.
- Document: Clearly document each strategy and when it should be used.
- Moderate use: Avoid creating excessive unnecessary strategies that complicate the system.
In the next chapter, we will explore the Template Pattern and how it can be used to define the skeleton of an algorithm.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
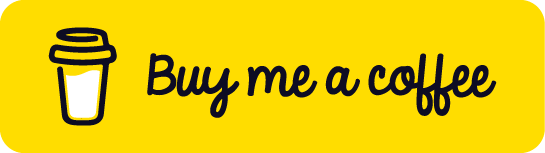
Chat with Chuck
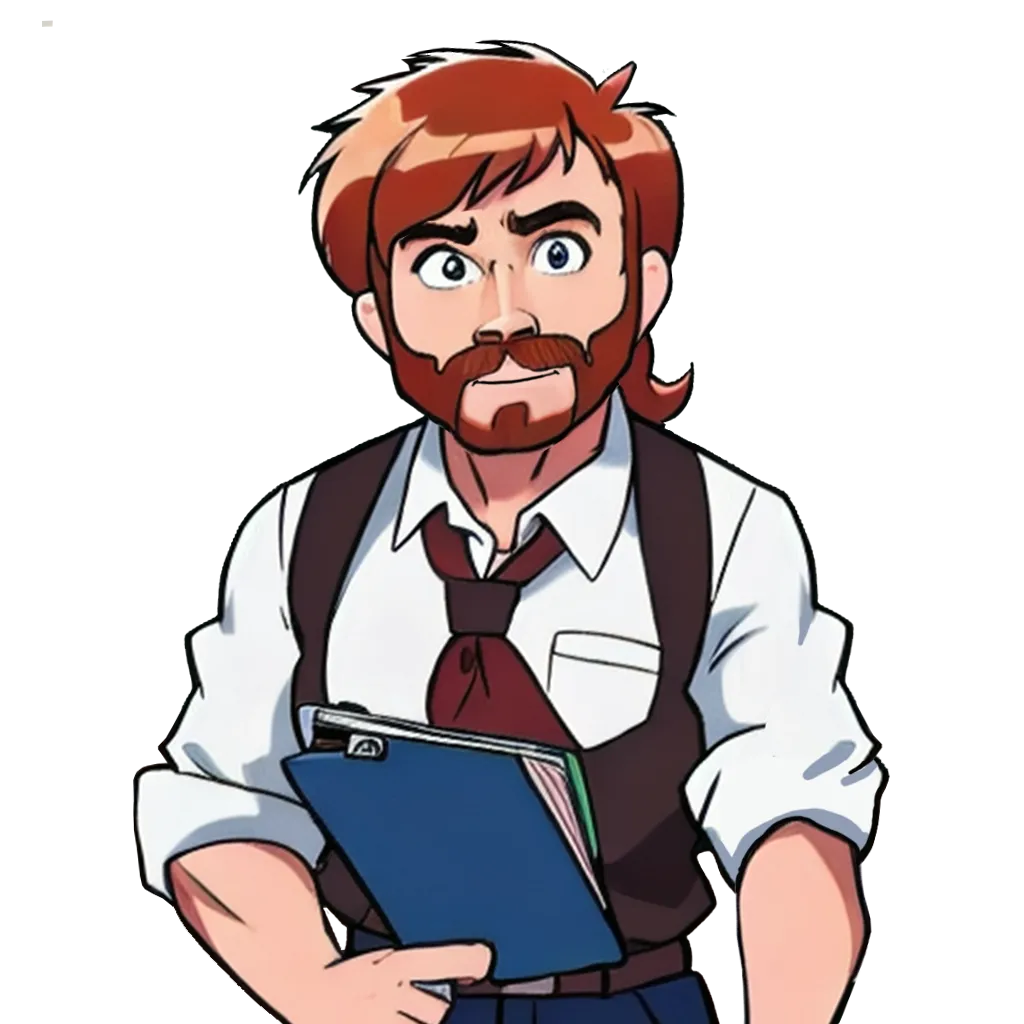
- Introduction to Design Patterns
- JavaScript and ES6 Fundamentals
- Singleton Pattern
- Factory Pattern
- Patrón Prototype
- Observer Pattern
- Module Pattern
- Revealing Module Pattern
- Mediator Pattern
- Decorator Pattern
- Command Pattern
- Strategy Pattern
- Template Pattern
- State Pattern
- Conclusions and Best Practices in Design Patterns