Design Patterns in JavaScript
Mediator Pattern
The Mediator pattern is a behavioral design pattern that defines an object that encapsulates how a set of objects interact. This pattern promotes communication between objects in a controlled and structured manner, reducing the coupling between them by making them communicate through a central mediator.
Characteristics of the Mediator Pattern
- Decoupling: Reduces direct coupling between objects by making them communicate through a mediator.
- Centralized Management: Manages interactions between multiple objects in a centralized manner.
- Simplicity: Simplifies complex interactions by making them pass through a single control point.
Benefits of the Mediator Pattern
- Maintenance: Facilitates system maintenance and modification by centralizing communication logic.
- Extensibility: Allows adding new functionalities and objects without significantly affecting existing code.
- Reusability: Facilitates the reuse of objects regardless of the system they are in, as interaction rules are centralized.
Implementing the Mediator Pattern in JavaScript
Below are examples of how to implement the Mediator pattern using modern ES6 syntax.
Example 1: Simple Mediator
javascript
In this example, Mediador
manages the communication between multiple colleagues (Colega
). When a colleague sends a message, the mediator ensures all other colleagues receive it.
Example 2: Mediator in a Chat System
Let's simplify a chat system using the Mediator pattern.
javascript
In this example, Chatroom
acts as an intermediary between chat participants. Participants can send and receive messages through the chatroom without communicating directly with each other.
Use Cases for the Mediator Pattern
The Mediator pattern is useful in situations where:
- Complex Interactions: There are complex interactions between multiple objects.
- Decoupling: You want to reduce direct coupling between objects.
- Centralized Communication: A central point is needed to manage communication between objects.
Considerations and Best Practices
- Avoid Overload: Although the Mediator pattern centralizes communication, it can become a bottleneck if it handles too many responsibilities.
- Clarity in Interactions: Document interactions managed by the mediator well to facilitate maintenance and understanding of the system.
- Clear Responsibilities: Ensure the mediator handles only communication and not business logic to maintain a separation of responsibilities.
In the next chapter, we will explore the Decorator Pattern and how it can be used to dynamically add responsibilities to objects.
- Introduction to Design Patterns
- JavaScript and ES6 Fundamentals
- Singleton Pattern
- Factory Pattern
- Patrón Prototype
- Observer Pattern
- Module Pattern
- Revealing Module Pattern
- Mediator Pattern
- Decorator Pattern
- Command Pattern
- Strategy Pattern
- Template Pattern
- State Pattern
- Conclusions and Best Practices in Design Patterns
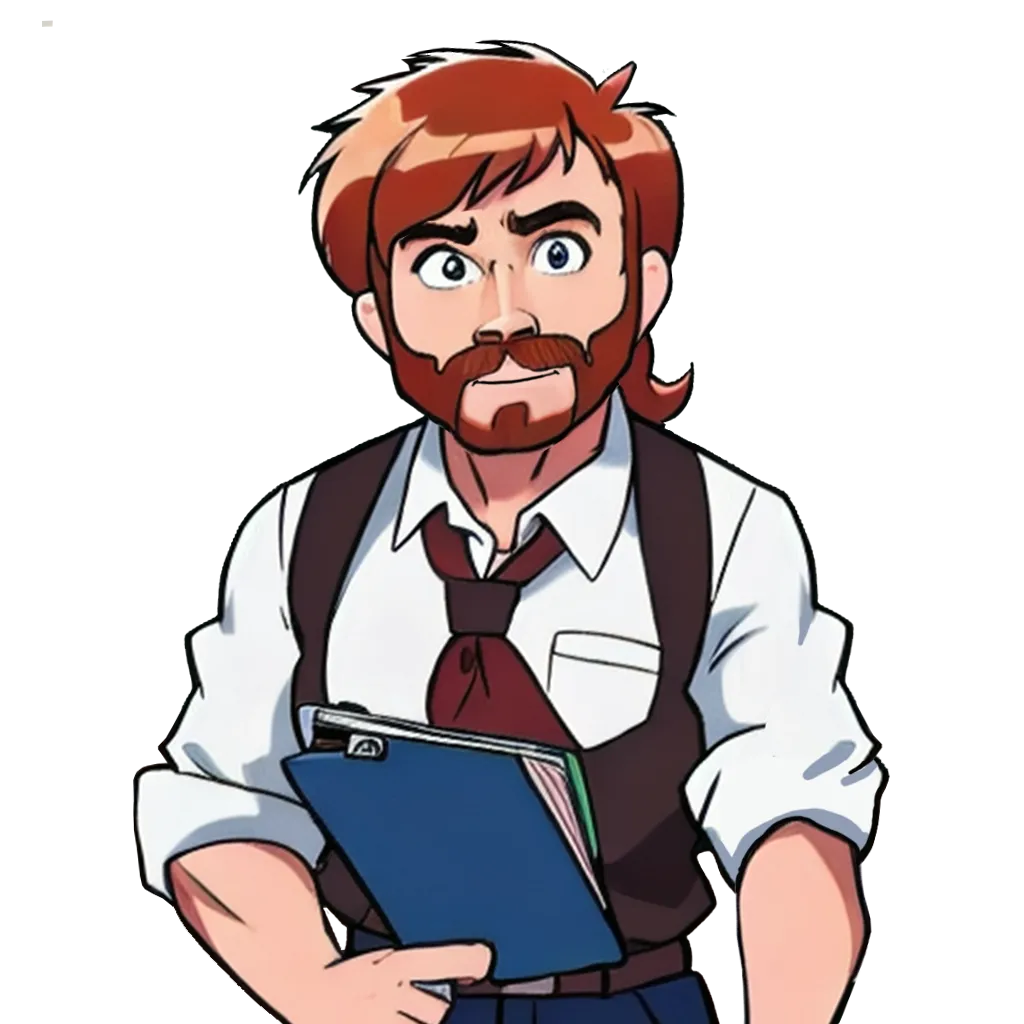