Design Patterns in JavaScript
Decorator Pattern
The Decorator pattern is a structural design pattern that allows responsibilities to be added to an object dynamically. This pattern provides a flexible alternative to inheritance for extending functionalities, allowing additional behaviors to be added by wrapping objects.
Features of the Decorator Pattern
- Extensibility: Allows additional behaviors to be added to objects transparently without modifying existing code.
- Composition: Uses composition instead of inheritance to extend the functionalities of objects.
- Flexibility: Decorators can be applied and combined dynamically, providing great flexibility.
Benefits of the Decorator Pattern
- Reusability: Promotes code reusability by allowing additional functionalities to be applied to different objects.
- Simplicity: Simplifies the extension of functionalities without needing to modify the structure of the original object.
- Separation of responsibilities: Separates additional responsibilities into specific decorators, keeping the code modular and manageable.
Implementation of the Decorator Pattern in JavaScript
Below are examples of how to implement the Decorator pattern using modern ES6 syntax.
Example 1: Class Decorator
javascript
In this example, DiscountedBook
acts as a decorator that adds additional behavior to the Book
class without modifying it.
Example 2: Nested Decorators
Decorators can be nested to add multiple additional responsibilities to an object.
javascript
In this example, the MilkDecorator
and ChocolateDecorator
are used to add additional responsibilities to the Coffee
object.
Use Cases for the Decorator Pattern
The Decorator pattern is useful in situations where:
- Extensibility: You need to add additional responsibilities to objects dynamically.
- Modularity: You prefer to keep the code modular and avoid directly altering the original object.
- Avoid complex hierarchies: You want to avoid creating complex class hierarchies through inheritance.
Considerations and Best Practices
- Avoid excessive nesting: Although decorators provide flexibility, excessive use can lead to a large amount of nesting that complicates code understanding.
- Sensible use: Use decorators in moderation and only when it's necessary to add responsibilities dynamically.
- Document: Ensure that decorators and the functionalities they add are well-documented to facilitate maintenance.
In the next chapter, we will explore the Command Pattern and how it can be used to encapsulate requests as objects.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
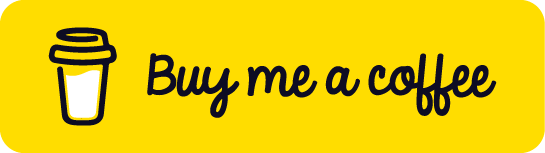
Chat with Chuck
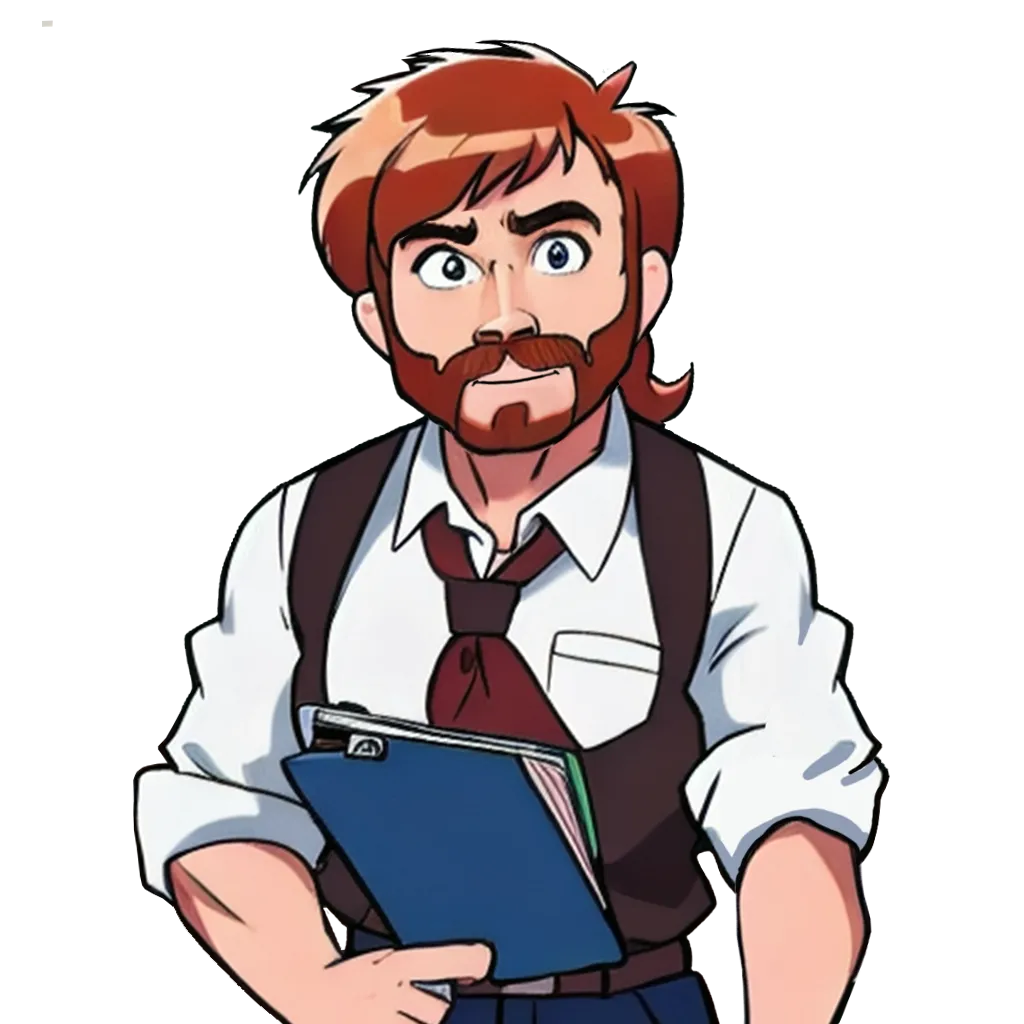
- Introduction to Design Patterns
- JavaScript and ES6 Fundamentals
- Singleton Pattern
- Factory Pattern
- Patrón Prototype
- Observer Pattern
- Module Pattern
- Revealing Module Pattern
- Mediator Pattern
- Decorator Pattern
- Command Pattern
- Strategy Pattern
- Template Pattern
- State Pattern
- Conclusions and Best Practices in Design Patterns