Design Patterns in JavaScript
Conclusions and Best Practices in Design Patterns
Conclusions
Throughout this course, we have explored various design patterns in JavaScript, each with its own characteristics, benefits, and use cases. Design patterns are powerful tools that can become crucial pieces for developing robust, scalable, and maintainable software. Below, we recap the key points and best practices for using these patterns effectively.
Recap of Patterns
- Singleton Pattern: Ensures a class has only one instance and provides a global access point to it.
- Factory Pattern: Provides an interface for creating objects in a superclass, allowing subclasses to alter the type of objects that will be created.
- Prototype Pattern: Allows creating objects by cloning an existing prototype.
- Observer Pattern: Defines a one-to-many dependency relationship, allowing multiple objects to be notified of changes in another object.
- Module Pattern: Encapsulates code, keeping variables and functions private while exposing only the necessary parts through a public interface.
- Revealing Module Pattern: A variant of the Module pattern that enhances the clarity and readability of the code by keeping all declarations within the private scope of the module.
- Mediator Pattern: Defines an object that encapsulates how a set of objects interact, reducing the coupling between them.
- Decorator Pattern: Allows adding responsibilities to an object dynamically without modifying its structure.
- Command Pattern: Turns requests or operations into objects, allowing parameterization, queuing, and logging operations.
- Strategy Pattern: Defines a family of algorithms, encapsulating them and making them interchangeable.
- Template Pattern: Defines the skeleton of an algorithm, allowing subclasses to redefine certain steps without altering its structure.
- State Pattern: Allows an object to change its behavior when its internal state changes.
Best Practices in Using Design Patterns
-
Understand the Problem: Before applying a design pattern, make sure you fully understand the problem you are trying to solve. Use design patterns not just for the sake of using them, but because they genuinely provide a suitable and efficient solution to the problem.
-
Simplicity and Clarity: Keep the implementation of patterns as simple and clear as possible. Avoid overcomplicating the design and follow the KISS principle (Keep It Simple, Stupid).
-
Documentation: Document the use of design patterns in your code. Explain why a specific pattern was used and how it helps solve the problem. This facilitates maintenance and understanding of the code by other developers.
-
Code Reuse: Design patterns encourage code reuse. Identify opportunities to reuse patterns in different parts of your application and in other projects.
-
Testing: Ensure that the components implementing design patterns are well-tested. It is important to verify that patterns work as expected and do not introduce bugs or failures.
-
Flexibility: Design patterns should bring flexibility to your system. Evaluate how patterns can facilitate future changes and extensions in your application.
-
Language Specificity: Consider the features and peculiarities of the programming language you are using. Design patterns can be adapted or slightly modified to take advantage of the language's capabilities (whether it's JavaScript, Python, Java, etc.).
-
Balance: Find a balance between the use of patterns and simplicity. Not all situations require a design pattern, and not all problems are complex enough to need one. Use patterns where they provide a clear benefit.
Final Considerations
Using design patterns does not automatically guarantee a good software design. They are powerful tools but must be used with judgement and understanding. A developer's ability to choose the right pattern or decide not to use one is crucial for creating efficient and maintainable software.
Additional Resources
To further delve into the world of design patterns, consider exploring the following resources:
-
Books:
- "Design Patterns: Elements of Reusable Object-Oriented Software" by Erich Gamma, Richard Helm, Ralph Johnson, and John Vlissides (Gang of Four).
- "JavaScript: The Good Parts" by Douglas Crockford.
-
Online Courses and Tutorials:
- Platforms like Coursera, Udemy, and Pluralsight offer specialized courses in design patterns.
- Websites like MDN Web Docs and freeCodeCamp have detailed tutorials and practical examples.
-
Communities and Forums:
- Participate in developer communities, like Stack Overflow, Reddit, or specific development forums, to discuss and learn more about design patterns.
In this course, we have covered a wide range of design patterns applied in JavaScript, providing you with a solid foundation to tackle common problems in software development in a structured and efficient manner.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
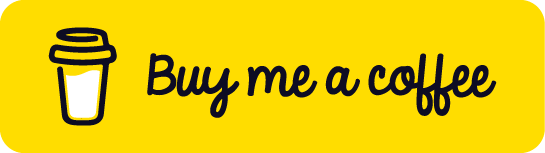
Chat with Chuck
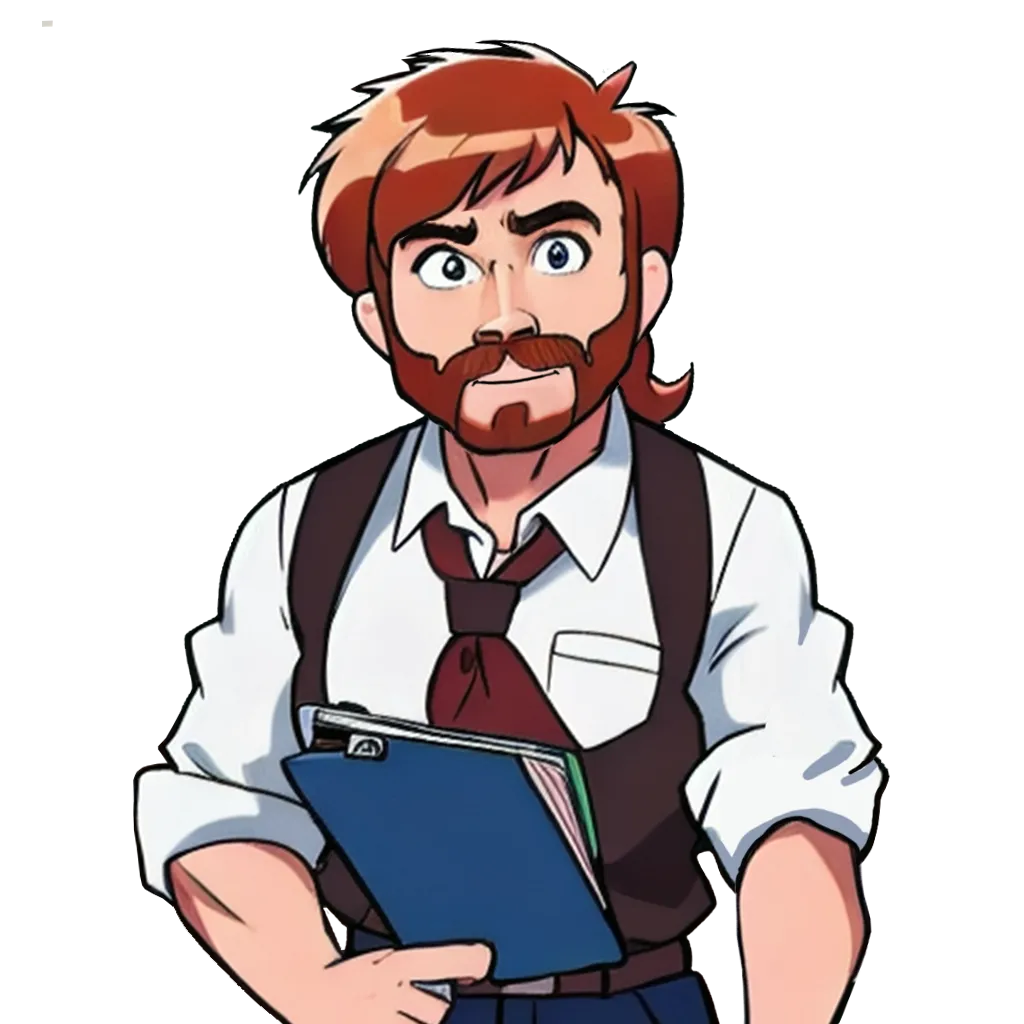
- Introduction to Design Patterns
- JavaScript and ES6 Fundamentals
- Singleton Pattern
- Factory Pattern
- Patrón Prototype
- Observer Pattern
- Module Pattern
- Revealing Module Pattern
- Mediator Pattern
- Decorator Pattern
- Command Pattern
- Strategy Pattern
- Template Pattern
- State Pattern
- Conclusions and Best Practices in Design Patterns