Design Patterns in JavaScript
Singleton Pattern
The Singleton pattern is one of the simplest and most common creational design patterns. Its purpose is to ensure that a class has only one instance and provides a global access point to that instance.
Features of the Singleton Pattern
- Unique Instance: Only one instance of the class is allowed.
- Global Access Point: The unique instance is accessible from anywhere in the system.
- Instance Control: The class itself controls the creation and access to the unique instance.
Benefits of the Singleton Pattern
- Access Control: By having a single instance, we can control resources and coordinate actions through this instance.
- Memory Usage Reduction: It avoids the creation of multiple instances, thus reducing memory consumption.
JavaScript Singleton Implementation
In JavaScript, we can implement a Singleton using functions or classes. Here we show how to do it using ES6 class syntax.
Example 1: Singleton with Class
javascript
In this example, we use a static property instance
to store the sole instance of the Singleton
class. If an instance already exists, the constructor simply returns that instance, ensuring that there are no multiple instances of the class.
Example 2: Singleton with IIFE (Immediately Invoked Function Expression)
Another way to implement a Singleton is through an IIFE and the module pattern.
javascript
In this approach, we use a self-executing function to encapsulate the creation of the instance. The function returns an object with a getInstance
method that creates and returns the unique instance.
Singleton Pattern Use Cases
The Singleton pattern is useful in various scenarios, such as:
- Configuration Management: Store global configurations accessible from any part of the application.
- Resource Controllers: Database controllers or file handlers where coordinated access is needed.
- Logging: A centralized logging service that can be invoked from any module without needing to create multiple instances.
Considerations and Best Practices
- Avoid Overuse: If overused, the Singleton pattern can overly couple certain parts of the application, making code testing and maintenance difficult.
- Use in Tests: In test environments, ensure that Singletons do not maintain state that can interfere with other tests.
- Lazy Initialization: Consider initializing the instance only when needed to improve performance and reduce resource consumption.
In the next chapter, we will look at another very useful creational pattern: the Factory Pattern.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
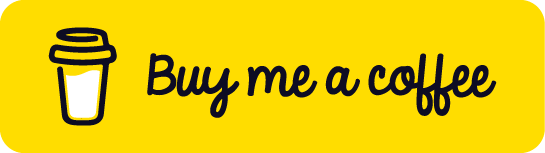
Chat with Chuck
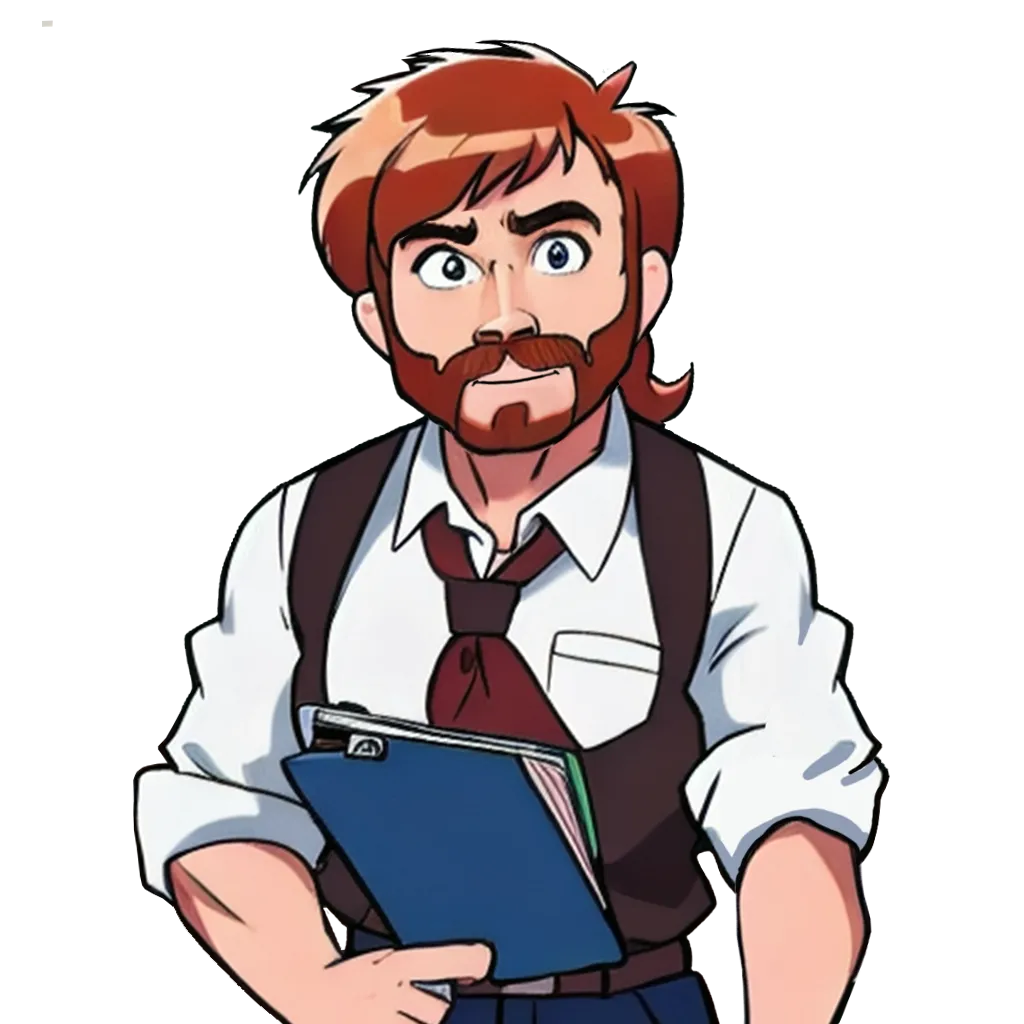
- Introduction to Design Patterns
- JavaScript and ES6 Fundamentals
- Singleton Pattern
- Factory Pattern
- Patrón Prototype
- Observer Pattern
- Module Pattern
- Revealing Module Pattern
- Mediator Pattern
- Decorator Pattern
- Command Pattern
- Strategy Pattern
- Template Pattern
- State Pattern
- Conclusions and Best Practices in Design Patterns