Design Patterns in JavaScript
Revealing Module Pattern
The Revealing Module Pattern is a variation of the Module Pattern aimed at improving code clarity and readability. The main idea behind this pattern is to keep all function and variable declarations within the private scope of the module and only expose the necessary references at the end through an object declaration.
Features of the Revealing Module Pattern
- Clarity: Improves code readability by keeping private functions and variables together.
- Simplicity: Facilitates code maintenance by exposing public references in a coherent and clear manner.
- Encapsulation: Maintains encapsulation and privacy of data and functionalities.
Benefits of the Revealing Module Pattern
- Readability: Makes the code easier to understand and follow.
- Consistency: Provides a consistent way of exposing public interfaces.
- Unification: Groups all declarations and exposures in a single place, reducing code scattering.
Implementing the Revealing Module Pattern in JavaScript
Below are examples of how to implement the Revealing Module Pattern using modern ES6 syntax.
Example: Simple Module with IIFE (Immediately Invoked Function Expression)
javascript
In this example, all variables and functions are declared within the private scope of the module and exposed at the end of the IIFE within the returned object.
Example: Module using ES6 with import
and export
With ES6, native support for modules makes the Revealing Module Pattern even simpler to implement and maintain.
File counter.js
:
javascript
File app.js
:
javascript
In this approach, the counter.js
file defines and exports an object that contains references to the necessary functions, while app.js
imports and uses it.
Use Cases of the Revealing Module Pattern
The Revealing Module Pattern is useful in situations where:
- Consistency: A coherent and well-defined public interface is desired.
- Readability: Improved code readability and maintainability are sought.
- Encapsulation: Clear and structured encapsulation of data and functionalities is needed.
Considerations and Best Practices
- Minimal Exposure: Expose only the functions and data that are necessary, keeping most of the code private.
- Intuitive Use: Ensure that the public interface is intuitive and easy to understand for other developers.
- Avoid Redundancies: Avoid repetition and redundancy in the declaration of functions and variables.
In the next chapter, we will explore the Mediator Pattern and how it can be used to manage communication between objects efficiently.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
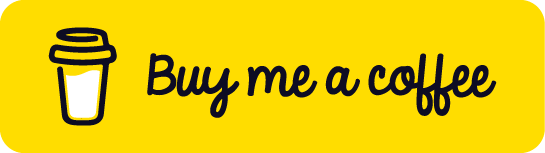
Chat with Chuck
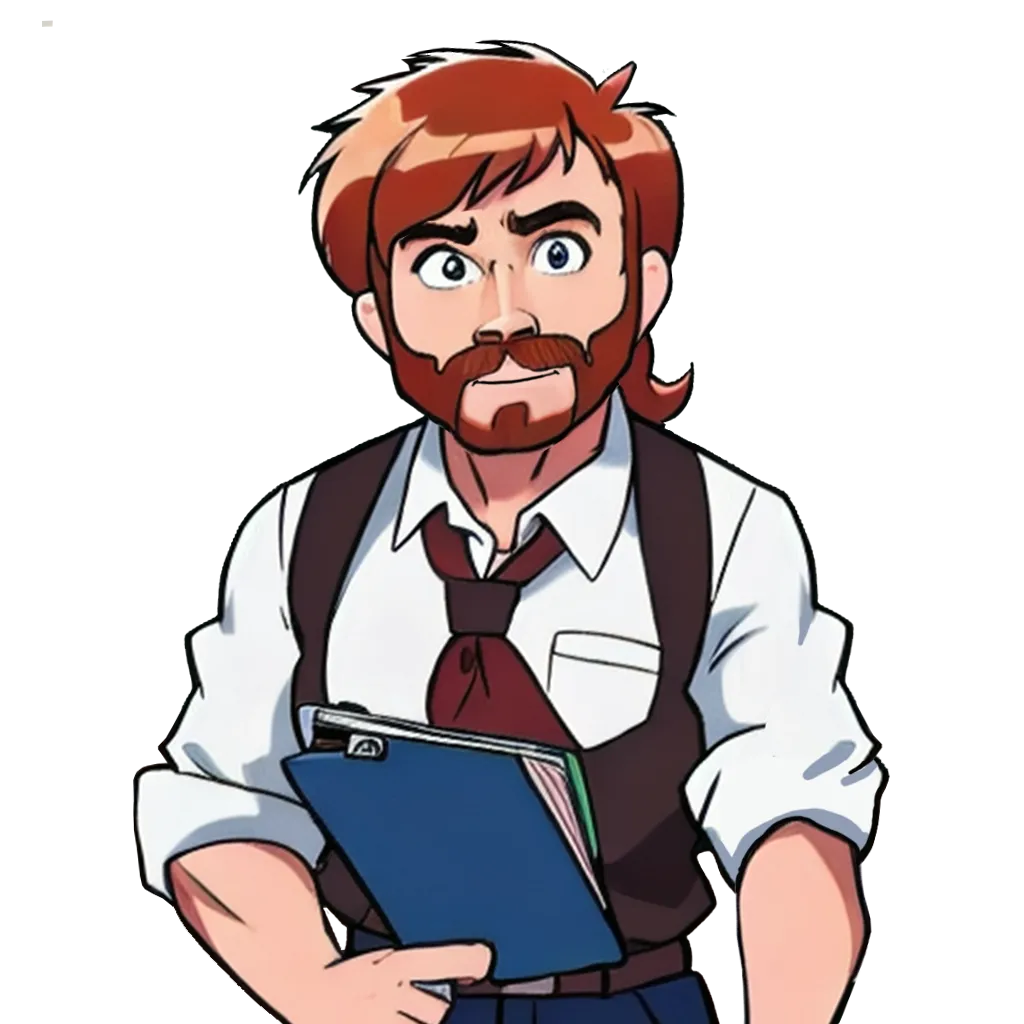
- Introduction to Design Patterns
- JavaScript and ES6 Fundamentals
- Singleton Pattern
- Factory Pattern
- Patrón Prototype
- Observer Pattern
- Module Pattern
- Revealing Module Pattern
- Mediator Pattern
- Decorator Pattern
- Command Pattern
- Strategy Pattern
- Template Pattern
- State Pattern
- Conclusions and Best Practices in Design Patterns