Design Patterns in JavaScript
Observer Pattern
The Observer pattern is a behavioral design pattern that defines a one-to-many dependency relationship between objects, so that when one object changes its state, all its dependents are automatically notified and updated. This pattern is very useful for implementing event and notification systems.
Features of the Observer Pattern
- Decoupling: Decouples the subject (or observable) from its observers, allowing both to evolve independently.
- Flexibility: Allows adding and removing observers at runtime.
- Automatic Notification: Observers are automatically notified of changes in the subject.
Benefits of the Observer Pattern
- Modularity: Facilitates breaking the system into smaller, more manageable modules.
- Maintenance: Simplifies maintenance by encapsulating notification and response to changes functionalities.
- Reactivity: Enables the implementation of reactive applications and event-based systems.
Implementation of the Observer Pattern in JavaScript
JavaScript provides several ways to implement the Observer pattern. Below are examples using modern ES6 syntax.
Example 1: Simple Implementation
javascript
In this example, Observable
manages a list of observers and has methods to subscribe, unsubscribe, and notify the observers.
Example 2: Practical Use with DOM Events
The Observer pattern is widely used in handling events in the DOM.
javascript
In this example, EventEmitter
allows registering, removing, and emitting events, which is very useful for handling custom events in web applications.
Use Cases of the Observer Pattern
The Observer pattern is useful in situations where:
- Event Systems: Implementing event and notification systems, such as reactive user interfaces.
- Decentralized Updates: Coordinating updates between decentralized objects.
- Pub/Sub Implementation: Creating publish/subscribe systems where subscribers react to certain events.
Considerations and Best Practices
- Avoid Notification Cycles: Ensure that the notification of observers does not create infinite cycles.
- Subscription Management: Keep proper track of subscriptions and ensure to unsubscribe observers when they are no longer needed.
- Document Events: Well document events and their possible values to facilitate the use and maintenance of the system.
In the next chapter, we will explore the Module Pattern and how it can be used to effectively structure code in JavaScript.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
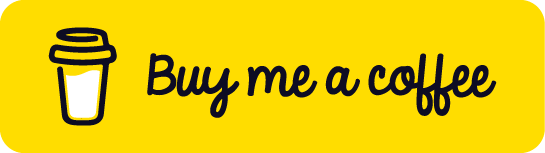
Chat with Chuck
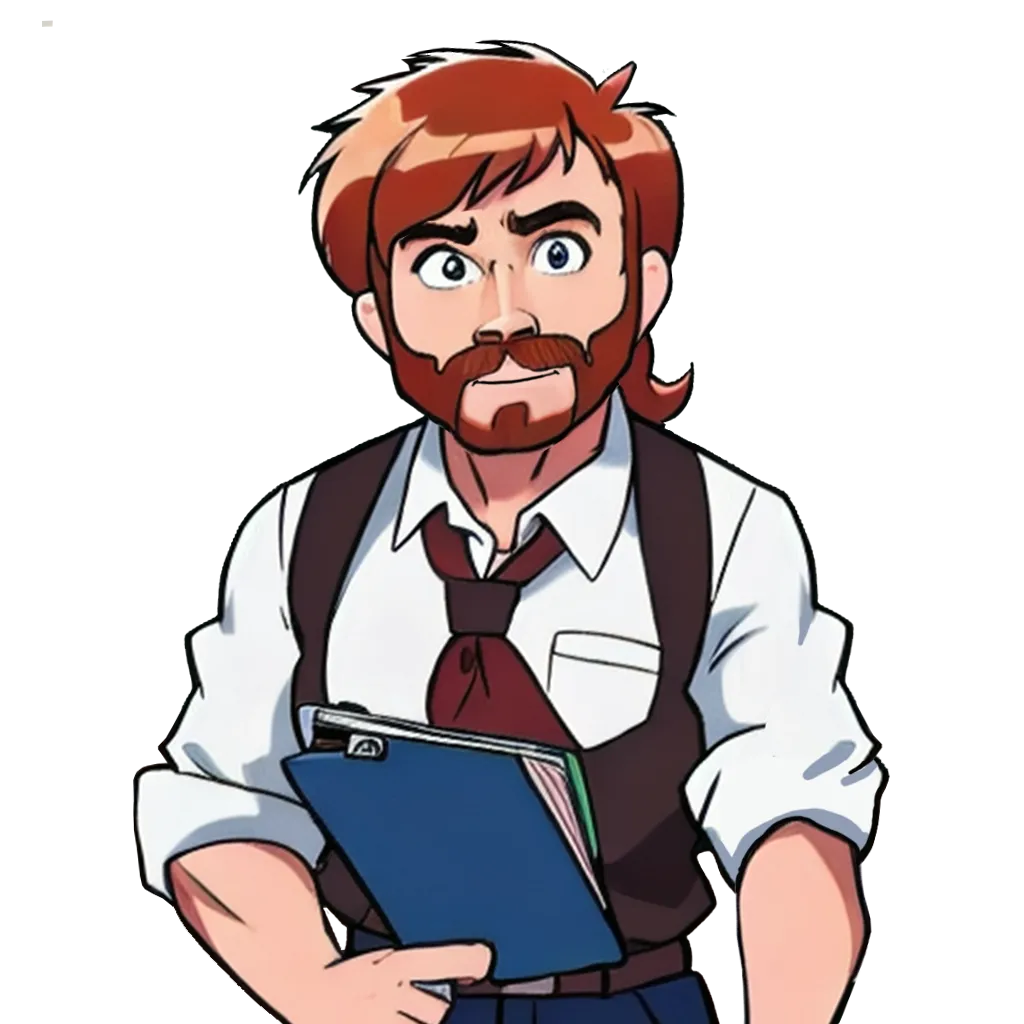
- Introduction to Design Patterns
- JavaScript and ES6 Fundamentals
- Singleton Pattern
- Factory Pattern
- Patrón Prototype
- Observer Pattern
- Module Pattern
- Revealing Module Pattern
- Mediator Pattern
- Decorator Pattern
- Command Pattern
- Strategy Pattern
- Template Pattern
- State Pattern
- Conclusions and Best Practices in Design Patterns