Design Patterns in JavaScript
Module Pattern
The Module pattern is a design pattern that provides a way to encapsulate code, maintaining the scope of variables and private functions, and exposing only the necessary parts of the code through a public interface. This pattern is especially useful for organizing and structuring code in JavaScript applications.
Characteristics of the Module Pattern
- Encapsulation: Offers the ability to encapsulate data and functionalities, avoiding the pollution of the global namespace.
- Privacy: Allows having private variables and methods accessible only within the module.
- Public interface: Exposes only the necessary functions and variables, maintaining a clear and manageable public interface.
Benefits of the Module Pattern
- Organization: Helps keep the code organized and structured.
- Reusability: Facilitates the reuse of modules in different parts of the application.
- Maintenance: Simplifies maintenance by reducing the scope of variables and functions.
Implementing the Module Pattern in JavaScript
The Module pattern can be implemented in various ways in JavaScript. Here are some approaches using ES6 syntax.
Example 1: Simple Module with IIFE (Immediately Invoked Function Expression)
javascript
In this example, the counter
, increment
, and decrement
variables are private and only accessible from within the module. The public interface exposes only the necessary functions.
Example 2: Module using ES6 with import
and export
With ES6, native support for modules makes the Module pattern even simpler and more effective to implement.
counter.js
file:
javascript
app.js
file:
javascript
In this approach, the counter.js
file defines and exports the necessary functions, while app.js
imports and uses them.
Use Cases of the Module Pattern
The Module pattern is useful in situations where:
- Encapsulation: It is necessary to encapsulate data and code to avoid polluting the global namespace.
- Organization: Organize code into manageable and reusable modules.
- Clear interface: Define a clear public interface to interact with the encapsulated code.
Considerations and Best Practices
- Minimize the public interface: Expose only the necessary functionalities to reduce complexity and increase security.
- Use ES6 modules: Take advantage of the native ES6 syntax and features for modules if the environment allows.
- Document: Always document the functions and variables exposed in the public interface to facilitate their use and maintenance.
In the next chapter, we'll explore the Revealing Module Pattern, a variation of the Module Pattern with a focus on making code clearer and more readable.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
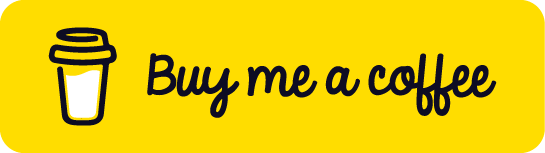
Chat with Chuck
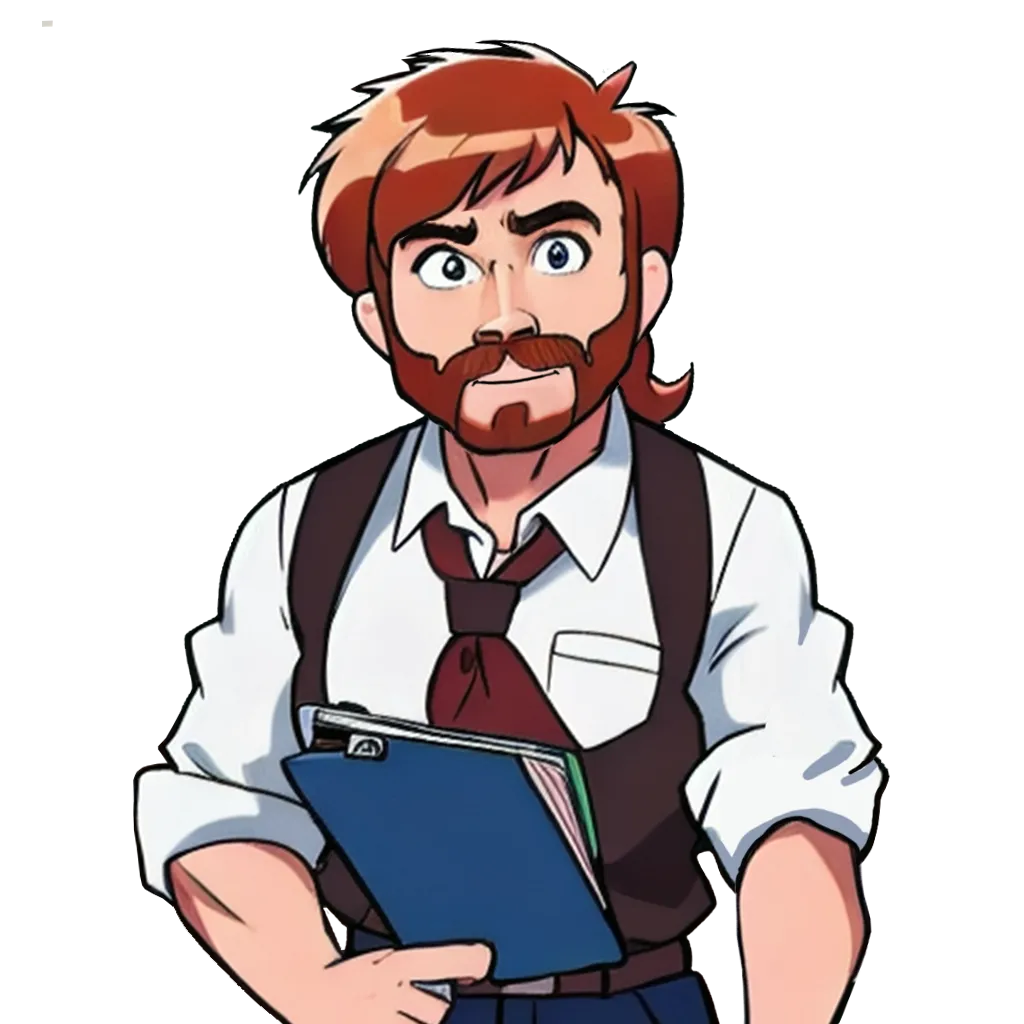
- Introduction to Design Patterns
- JavaScript and ES6 Fundamentals
- Singleton Pattern
- Factory Pattern
- Patrón Prototype
- Observer Pattern
- Module Pattern
- Revealing Module Pattern
- Mediator Pattern
- Decorator Pattern
- Command Pattern
- Strategy Pattern
- Template Pattern
- State Pattern
- Conclusions and Best Practices in Design Patterns