Design Patterns in JavaScript
State Pattern
The State pattern is a behavioral design pattern that allows an object to change its behavior when its internal state changes. This pattern is especially useful when an object needs to change its behavior based on its state, eliminating large conditionals and promoting the use of composition over inheritance.
Characteristics of the State Pattern
- State independence: Each state is represented as a separate class that encapsulates the behavior associated with that state.
- Dynamic change: The main object can change its state at runtime.
- Decoupling: Promotes decoupling by moving the state-specific behavior to different state classes.
Benefits of the State Pattern
- Simplicity and clarity: Eliminates large conditional structures, making the code more readable and maintainable.
- Flexibility: Facilitates the addition of new states without changing the code of the main object.
- Encapsulation: States and associated behaviors are well encapsulated and not mixed.
Implementing the State Pattern in JavaScript
Below are examples of how to implement the State pattern using modern ES6 syntax.
Example 1: Vending Machine
javascript
In this example, VendingMachine
uses different states (NoCoinState
, HasCoinState
, SoldState
) to handle behavior based on its different states.
Example 2: Music Player
javascript
In this example, MusicPlayer
uses different states (PlayingState
, PausedState
, StoppedState
) to handle its actions based on its current state.
Use Cases of the State Pattern
The State pattern is useful in situations where:
- Behavior change: The behavior of an object needs to change dynamically based on its state.
- Simplifying conditionals: There is a desire to eliminate large conditional structures that manage different states.
- Flexibility and extension: A flexible way to add new states and behaviors without modifying existing code is required.
Considerations and Best Practices
- Clear separation: Maintain a clear separation between states and the main object.
- Document: Document states and transitions well to facilitate maintenance.
- Simplify: Use the State pattern to simplify the handling of complex states and avoid nested conditionals.
In the next chapter, we will explore Conclusions and Best Practices in Design Patterns, summarizing what has been learned and providing additional tips for their application.
- Introduction to Design Patterns
- JavaScript and ES6 Fundamentals
- Singleton Pattern
- Factory Pattern
- Patrón Prototype
- Observer Pattern
- Module Pattern
- Revealing Module Pattern
- Mediator Pattern
- Decorator Pattern
- Command Pattern
- Strategy Pattern
- Template Pattern
- State Pattern
- Conclusions and Best Practices in Design Patterns
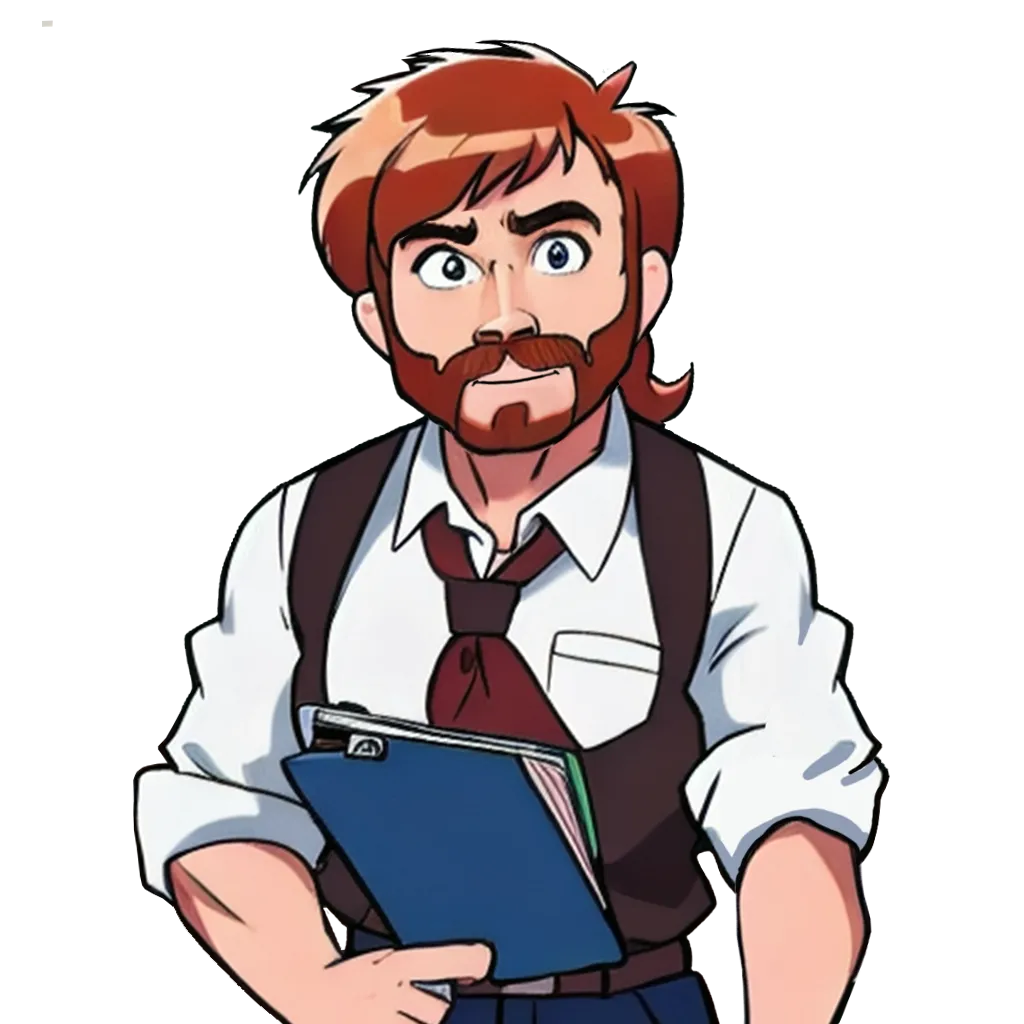