Design Patterns in JavaScript
JavaScript and ES6 Fundamentals
Before diving into design patterns in JavaScript, it's crucial to understand the fundamentals of JavaScript and the features introduced in ECMAScript 6 (ES6). This chapter serves as a refresher to ensure we're all on the same page.
Variables and Scope
JavaScript allows declaring variables using var
, let
, and const
.
var
has function scope and can be redeclared.let
has block scope and cannot be redeclared in the same scope.const
is similar tolet
, but in addition to being block-scoped, it creates a constant that cannot be reassigned.
javascript
Arrow Functions
Arrow functions provide a more compact syntax for writing functions. Additionally, they do not have their own this
context, which can be useful in certain cases.
javascript
Classes and Inheritance
ES6 introduced class syntax, making it easier to create objects and handle inheritance.
javascript
Destructuring
Destructuring allows you to extract values from arrays or objects and assign them to variables.
javascript
Default Parameters and Rest/Spread
We can assign default values to function parameters and use the rest/spread operator to handle multiple arguments.
javascript
Promises and Async/Await
Promises are essential for handling asynchronous operations in JavaScript. ES8 introduced async
and await
, which simplify working with promises.
javascript
To understand and effectively apply design patterns, it's fundamental to be comfortable with these modern JavaScript features.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
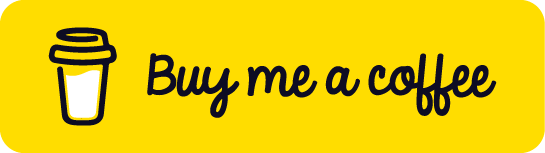
Chat with Chuck
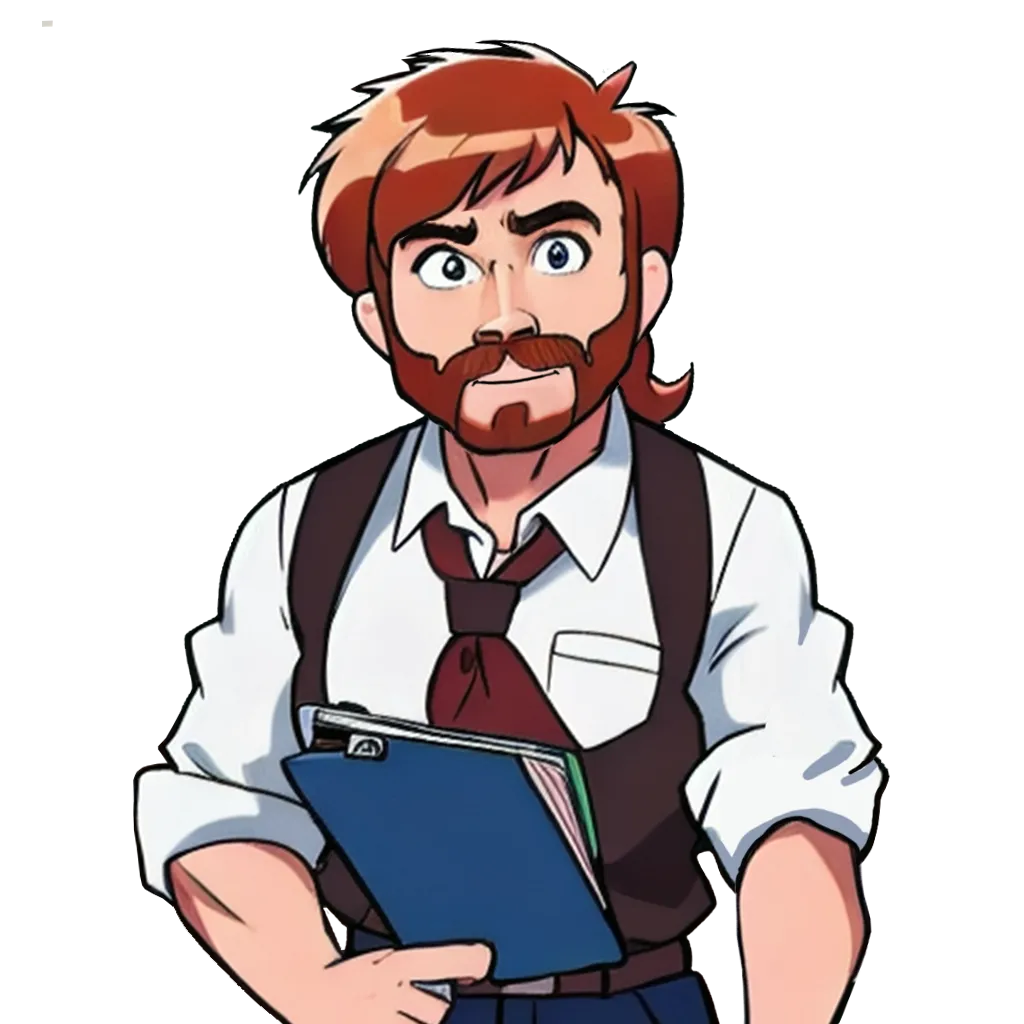
- Introduction to Design Patterns
- JavaScript and ES6 Fundamentals
- Singleton Pattern
- Factory Pattern
- Patrón Prototype
- Observer Pattern
- Module Pattern
- Revealing Module Pattern
- Mediator Pattern
- Decorator Pattern
- Command Pattern
- Strategy Pattern
- Template Pattern
- State Pattern
- Conclusions and Best Practices in Design Patterns