Design Patterns in JavaScript
Factory Pattern
The Factory Pattern is a creational design pattern that provides an interface for creating objects in a superclass but allows subclasses to alter the type of objects that will be created. This pattern helps us handle and manipulate collections of related objects without needing to specify their concrete classes.
Characteristics of the Factory Pattern
- Decoupling: Decouples the creation of objects from their actual implementation.
- Flexibility: Allows us to change the creation logic without modifying client code.
- Reusability: Facilitates code reuse by centralizing the object creation logic.
Benefits of the Factory Pattern
- Encapsulation: The object creation logic is encapsulated.
- Maintenance: Eases maintenance and extension of the code.
- Testability: Makes it easier to perform unit and integration testing.
Implementation of the Factory Pattern in JavaScript
There are different ways to implement the Factory Pattern in JavaScript. Below, we present some techniques using ES6.
Example 1: Factory Method
In the Factory Method approach, a class contains a method that is used to create objects.
javascript
Example 2: Abstract Factory
The Abstract Factory pattern provides an interface for creating families of related objects without specifying their concrete classes.
javascript
Use Cases of the Factory Pattern
The Factory Pattern is useful in situations where:
- Creation of complex objects: When the object creation involves complex logic.
- Change of concrete classes: When we need to change the concrete classes of the objects being created without modifying client code.
- Large number of subclasses: When there are many subclasses and we want to centralize the instantiation process.
Considerations and Best Practices
- Maintain simplicity: Although the Factory Pattern can solve many problems, use it in moderation to avoid unnecessary complexity.
- Document subclasses: Ensure to document subclasses and their behavior well to facilitate maintenance.
- Use in combination: Consider using the Factory Pattern in combination with other patterns like Singleton or Decorator for more robust solutions.
In the next chapter, we will explore the Prototype Pattern and how it can be leveraged in JavaScript.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
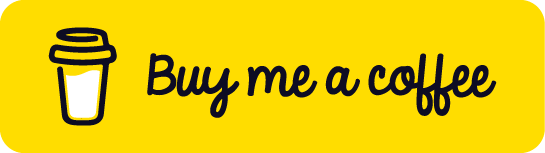
Chat with Chuck
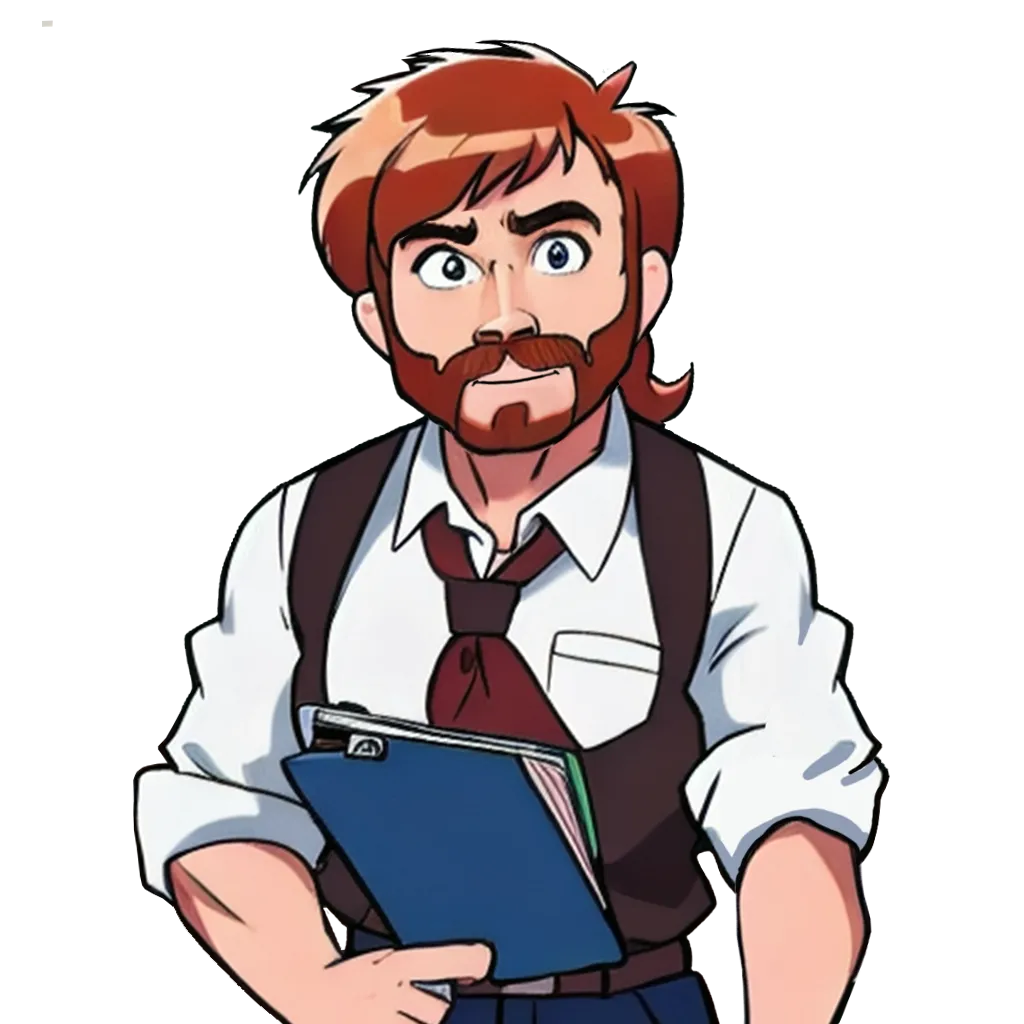
- Introduction to Design Patterns
- JavaScript and ES6 Fundamentals
- Singleton Pattern
- Factory Pattern
- Patrón Prototype
- Observer Pattern
- Module Pattern
- Revealing Module Pattern
- Mediator Pattern
- Decorator Pattern
- Command Pattern
- Strategy Pattern
- Template Pattern
- State Pattern
- Conclusions and Best Practices in Design Patterns