Design Patterns in JavaScript
Template Pattern
The Template Pattern (Template Method) is a behavioral design pattern that defines the skeleton of an algorithm in an operation, separating some of its steps without changing the overall structure. This pattern allows subclasses to redefine certain steps of an algorithm without altering its general structure.
Features of the Template Pattern
- Common Structure: Defines a generic skeleton for common algorithms.
- Customization: Allows subclasses to alter certain steps of the algorithm without changing its overall structure.
- Consistency: Ensures that the structure of the algorithm remains consistent while allowing various approaches in specific steps.
Benefits of the Template Pattern
- Code Reuse: Avoids code duplication by encapsulating the general structure of the algorithm in a base class.
- Flexibility: Allows customizing specific steps of the algorithm in derived classes.
- Maintenance: Facilitates maintenance by centralizing the skeleton of the algorithm in one place.
Implementing the Template Pattern in JavaScript
Below are examples of how to implement the Template Pattern using modern ES6 syntax.
Example 1: Preparing Coffee and Tea
javascript
In this example, Beverage
defines the skeleton of the prepareRecipe
algorithm, while Coffee
and Tea
implement the specific steps prepare
and addCondiments
.
Example 2: Document Construction Process
javascript
In this example, Document
defines the skeleton of the document construction, while Report
and Invoice
implement the specific steps addContent
and render
.
Use Cases for the Template Pattern
The Template Pattern is useful in situations where:
- Common Algorithms: There are common algorithms that share the same structure but differ in some steps.
- Consistency and Flexibility: There is a need to maintain consistency in the algorithm structure while allowing flexibility in implementing specific steps.
- Decoupling Variations: There is a desire to decouple variations in the algorithm steps without duplicating the generic structure.
Considerations and Best Practices
- Simple and Clear: Keep the template method simple and clear to make it easy to understand and maintain.
- Document: Make sure to document the abstract methods that must be implemented by subclasses.
- Avoid Unnecessary Dependencies: Keep internal dependencies of the concrete steps minimal and focused to ensure reuse.
In the next chapter, we will explore the State Pattern and how it can be used to manage internal states and transitions in objects.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
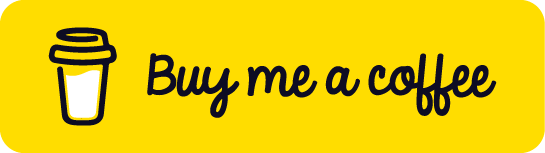
Chat with Chuck
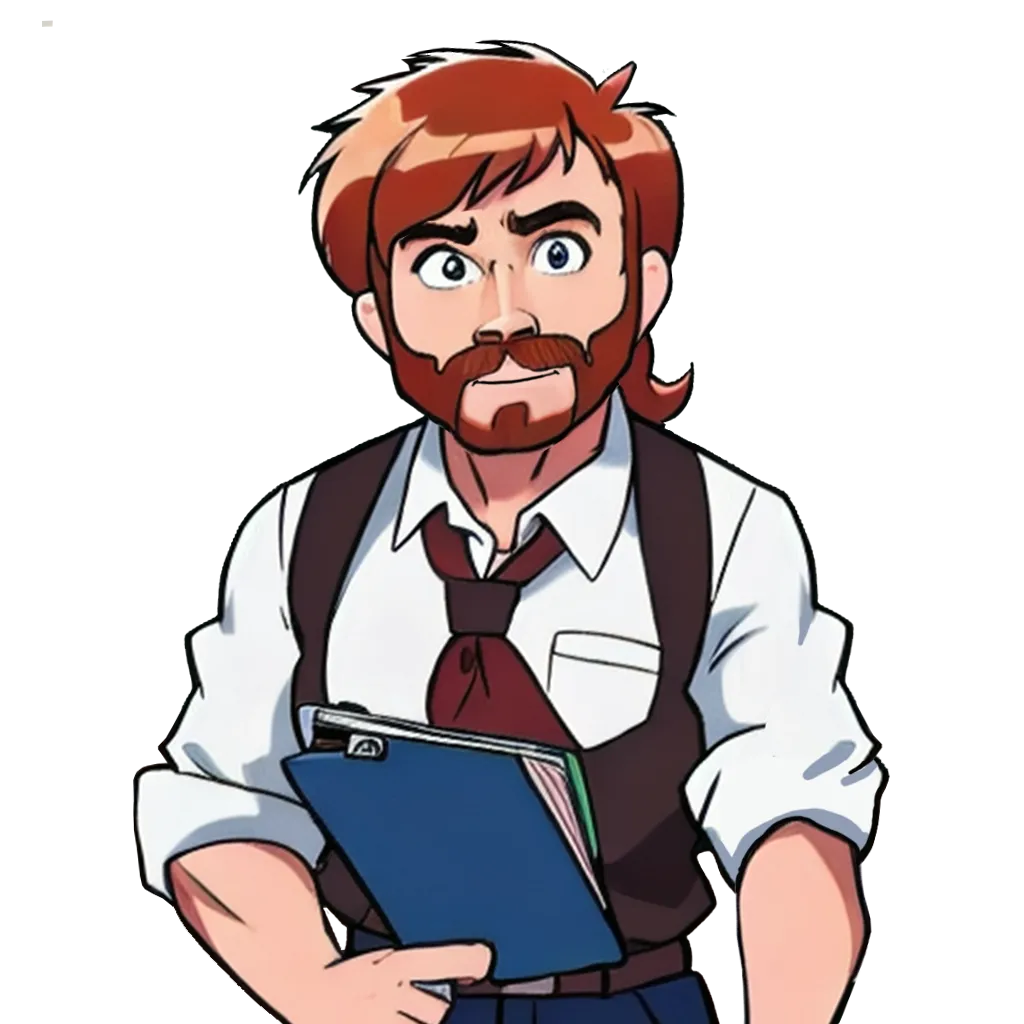
- Introduction to Design Patterns
- JavaScript and ES6 Fundamentals
- Singleton Pattern
- Factory Pattern
- Patrón Prototype
- Observer Pattern
- Module Pattern
- Revealing Module Pattern
- Mediator Pattern
- Decorator Pattern
- Command Pattern
- Strategy Pattern
- Template Pattern
- State Pattern
- Conclusions and Best Practices in Design Patterns