React Context API
Advanced Patterns with Context API
The Context API in React is a powerful tool, and once you master the basics, you can explore advanced patterns to handle more complex states and optimize your application. In this chapter, we'll look at various advanced patterns and examples of how to implement them.
Compound Contexts Pattern
The compound contexts pattern involves combining multiple contexts in a single provider. This pattern is useful when you need to provide multiple context values to multiple components but want to avoid excessive nesting.
Example of Compound Contexts
jsx
In this example, ComposedProvider
is used to combine multiple contexts in a single provider, simplifying the component tree.
Reducers with Context Pattern
A commonly used advanced pattern is combining useReducer
with Context API to handle complex state logic. This pattern is very useful for managing global states that have multiple actions and transitions.
Example with useReducer
jsx
In this example, useReducer
is combined with Context API to handle more complex state with multiple actions.
HOC (Higher-Order Components) Pattern
Using HOCs with Context API can be useful for easily providing contexts to specific components without altering the component tree hierarchy.
Example of HOC with Context
jsx
In this example, the HOC withUser
is used to inject context values into UserProfile
without directly modifying the component.
Render Props Pattern
The render props pattern can be used with Context API to provide context data to child components via a function.
Example of Render Props with Context
jsx
In this example, the render props pattern is used with UserContext.Consumer
to provide context data to UserProfile
.
Conclusion
Exploring advanced patterns with Context API can open up new possibilities for managing states and data more efficiently and in an organized manner in a React application. From combining contexts and using reducers to employing HOCs and render props, these patterns can help you build more robust and maintainable applications. In the next chapter, we will see how to combine Context API with functional components to achieve maximum efficiency and flexibility.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
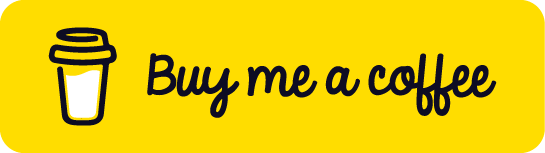
Chat with Chuck
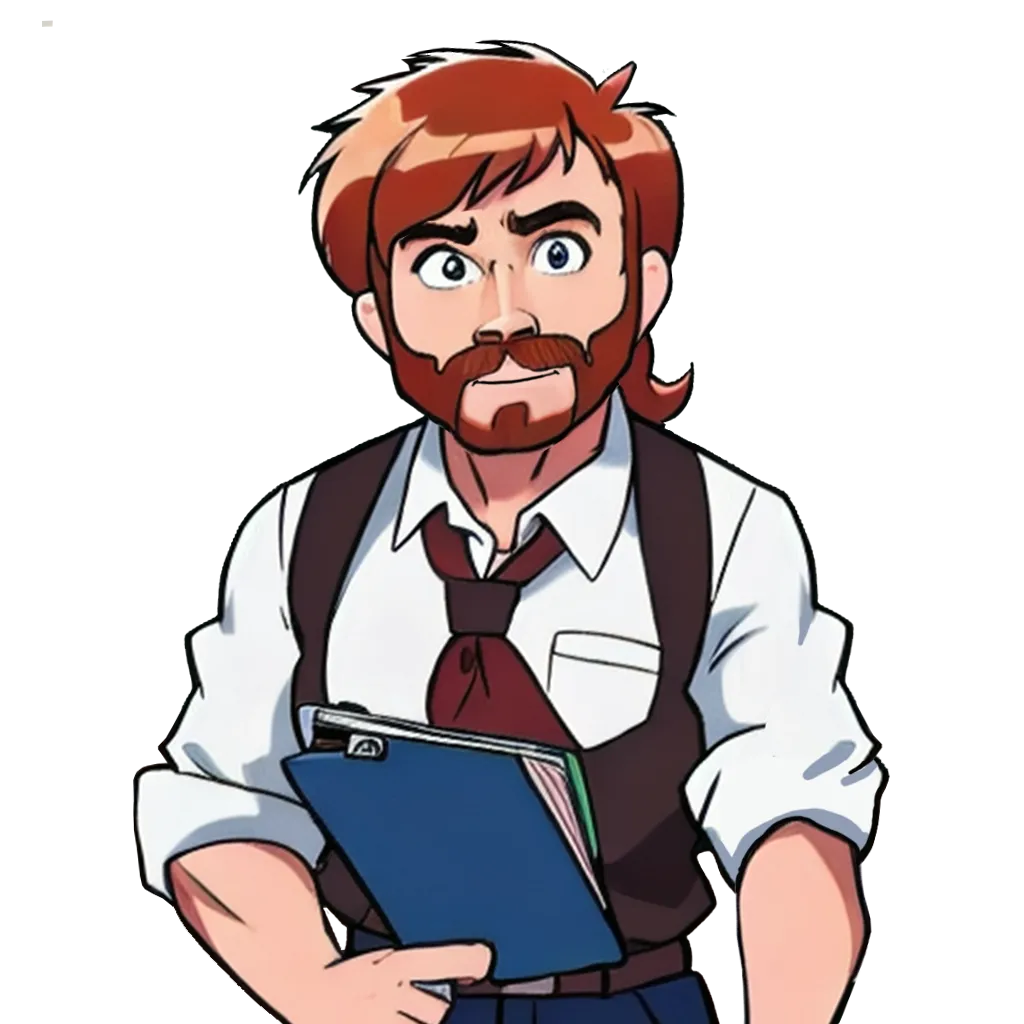
- Introduction to the React Context API
- Fundamentals of State in React
- Creating a Context in React
- Context Provider
- Context Consumer (Context Consumer)
- Using Context with the `useContext` Hook
- Context API vs Prop Drilling
- Context Update
- Nested Contexts
- Context API and Performance
- Advanced Patterns with Context API
- Context API and Functional Components
- Context API and Class Components
- Testing con Context API
- Conclusion and Best Practices