React Context API
Context API and Performance
The use of Context API in React can have a significant impact on your application's performance. It is crucial to understand how contexts and their updates affect component rendering to optimize properly. In this chapter, we will explore various strategies and best practices for handling performance when using Context API.
Impact of Context on Rendering
Every time a context value changes, all consumer components of that context re-render. This behavior can lead to unnecessary renderings, especially in large and complex applications.
Example of Inefficient Renderings
jsx
In this example, when the user's name changes via UserSettings
, both UserProfile
and UserSettings
re-render, even though only one visually depends on the user data.
Optimization Techniques
1. Memoization
Utilizing useMemo
and useCallback
can help prevent unnecessary renderings by memoizing values and functions, ensuring they are recreated only when necessary.
Example with useMemo
jsx
In this example, using useMemo
to memoize providerValue
ensures that the values and functions are recreated only when the user
state changes.
2. Splitting Contexts
Splitting the state into multiple contexts can isolate parts of the state that frequently change, reducing the number of components that re-render unnecessarily. This is especially useful for large or complex states.
Example with Separate Contexts
jsx
In this example, the user and theme states are handled by separate contexts, preventing a theme change from affecting components that depend on the user context, and vice versa.
3. Conditional Rendering
At times, it is possible to conditionally render certain components to avoid unnecessary re-renderings using techniques like React's memoization or external state management libraries.
Example with React.memo
jsx
In this example, UserSettings
is memoized using React.memo
, which prevents it from re-rendering unless its props have changed.
Conclusion
Optimizing your application's performance when using Context API is essential, especially in large and complex applications. Techniques like memoization, splitting contexts, and conditional rendering can help you prevent unnecessary renderings and improve your application's efficiency. In the next chapter, we will explore advanced patterns with Context API to handle states and data more effectively.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
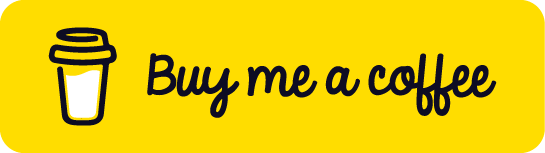
Chat with Chuck
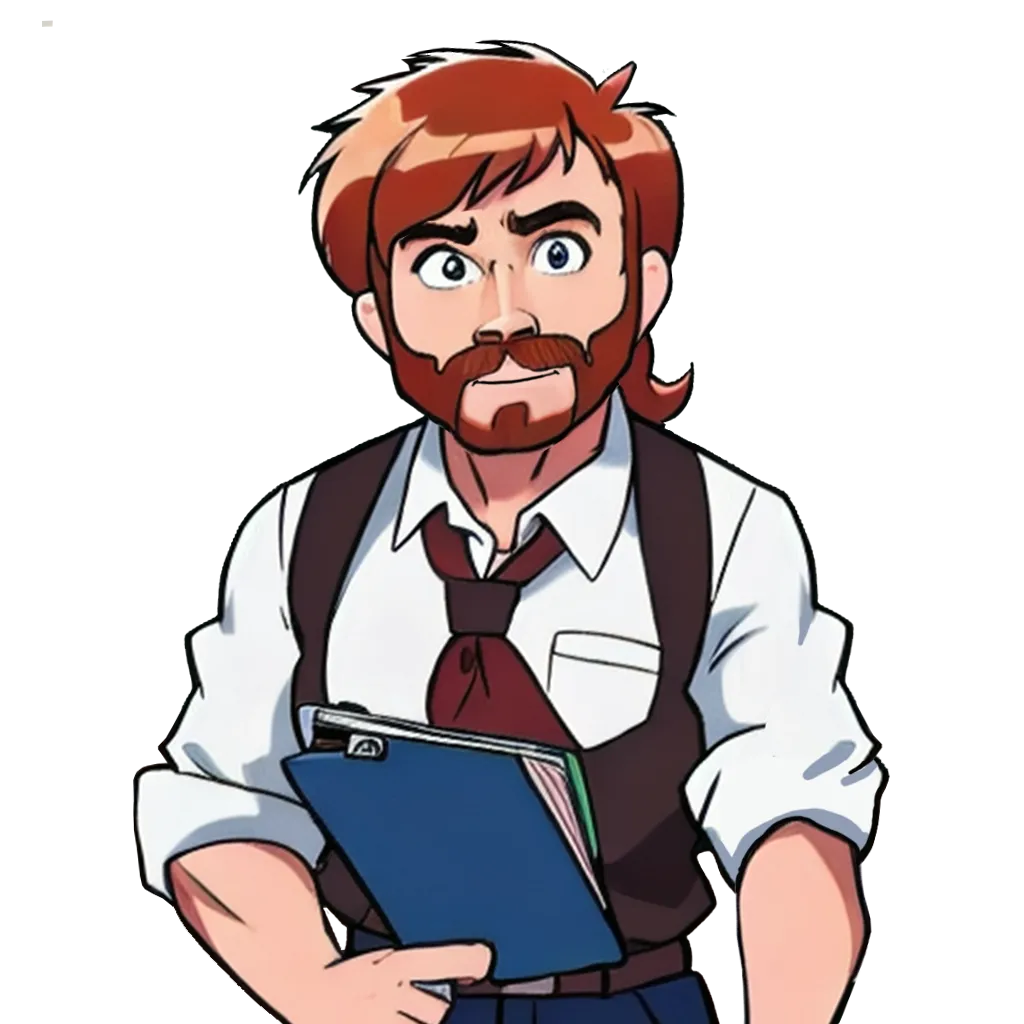
- Introduction to the React Context API
- Fundamentals of State in React
- Creating a Context in React
- Context Provider
- Context Consumer (Context Consumer)
- Using Context with the `useContext` Hook
- Context API vs Prop Drilling
- Context Update
- Nested Contexts
- Context API and Performance
- Advanced Patterns with Context API
- Context API and Functional Components
- Context API and Class Components
- Testing con Context API
- Conclusion and Best Practices