React Context API
Context API vs Prop Drilling
In React application development, sharing data between components can become a challenge, especially when this data needs to be accessible at various levels of the component tree. Two common patterns for handling this data exchange are the Context API and prop drilling. In this chapter, we will compare both approaches and analyze their advantages and disadvantages.
What is Prop Drilling?
Prop drilling is a pattern where properties (props) are passed from a parent component to its child components, and then to the children of those children, until the component that actually needs the data is reached. This approach can be effective in simple applications but can become complicated and hard to manage in larger and more complex applications.
Example of Prop Drilling
jsx
In this example, the user
props are passed from App
to GrandChildComponent
through ParentComponent
and ChildComponent
.
What is Context API?
The React Context API provides a way to pass data through the component tree without having to pass props manually at every level. This makes it easier to manage global data and keeps the code cleaner and easier to maintain.
Example using Context API
jsx
Here, the UserContext.Provider
provides the user
object directly to GrandChildComponent
, eliminating the need to pass props through multiple levels of components.
[Placeholder: Image showing a component tree with Context Provider
and Context Consumer
, linking how the context is distributed through the tree]
Advantages and Disadvantages
Prop Drilling
Advantages:
- Simplicity: It is easy to understand and use in small applications.
- Control: Each component has explicit control over the data it receives and passes.
Disadvantages:
- Verbose: Requires manually passing props through multiple component levels.
- Maintenance Difficulty: As the application grows, this pattern can make the code difficult to maintain and understand.
- Changes Propagation: Any changes in the props require updating all intermediate components.
Context API
Advantages:
- Cleanliness: Simplifies handling and distributing global data without passing props manually.
- Readability: The code is cleaner and easier to follow.
- Maintainability: Facilitates the maintenance and scalability of the application.
Disadvantages:
- Performance: Every time the context value changes, all consumers of the context re-render, which can impact performance.
- Debugging: Can be harder to debug, especially when multiple contexts are used in a large application.
Performance Considerations
When using the Context API, it's important to consider how changes in the context affect the rendering of the consumer components. To mitigate performance issues, techniques such as memoization (useMemo
) or separating contexts for different types of data can be used.
Conclusion
Both Context API and prop drilling have their pros and cons, and the choice between them depends on the specific needs of your application. The Context API is ideal for cases where multiple components need access to the same data without passing props through multiple levels. On the other hand, prop drilling can be sufficient and simpler in small applications or components that do not have many nested levels. In the next chapter, we will explore how to efficiently update context values.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
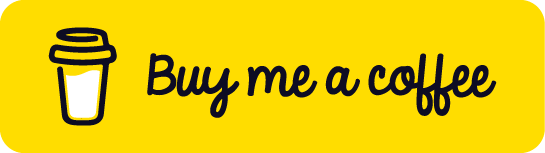
Chat with Chuck
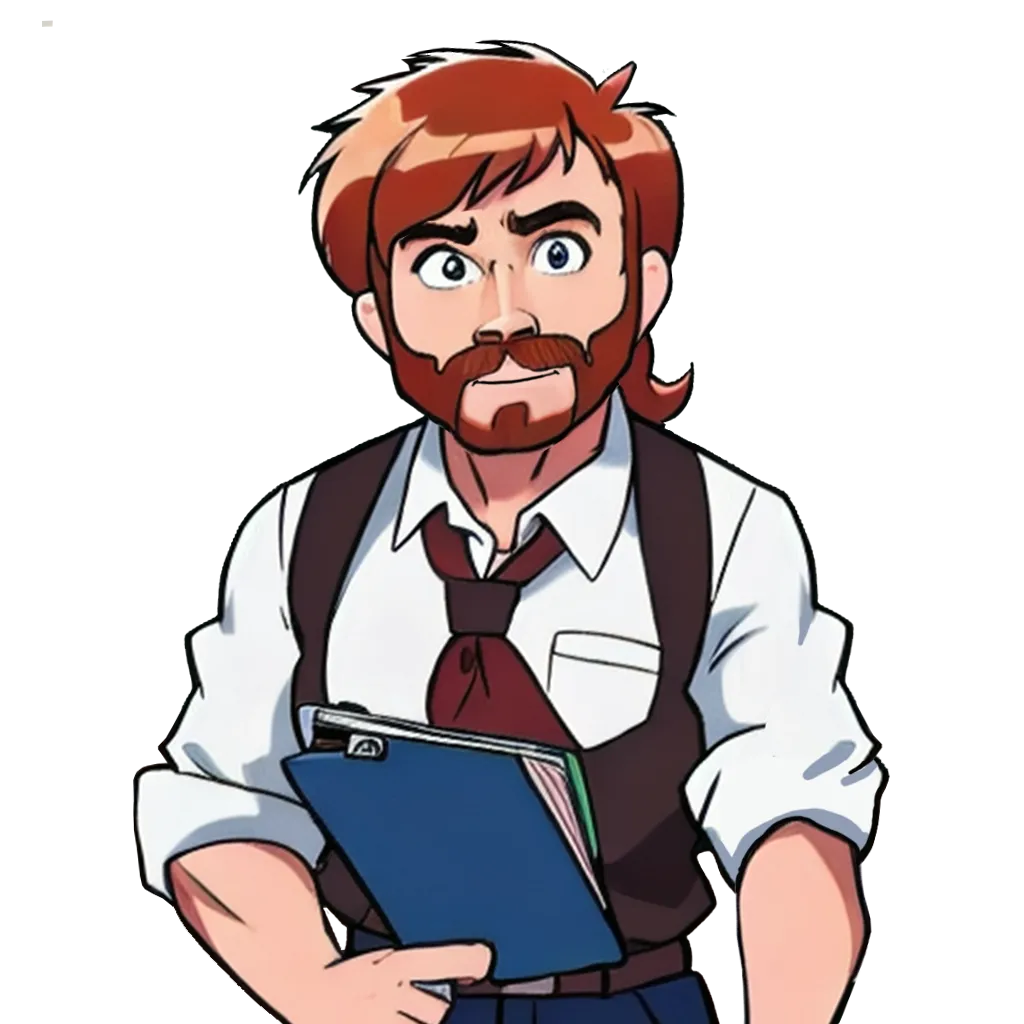
- Introduction to the React Context API
- Fundamentals of State in React
- Creating a Context in React
- Context Provider
- Context Consumer (Context Consumer)
- Using Context with the `useContext` Hook
- Context API vs Prop Drilling
- Context Update
- Nested Contexts
- Context API and Performance
- Advanced Patterns with Context API
- Context API and Functional Components
- Context API and Class Components
- Testing con Context API
- Conclusion and Best Practices