React Context API
Context Provider
The Context Provider
is a fundamental component of the Context API in React. It acts as the data provider and wraps the components that need to access those data. This section will delve into the usage and best practices of the Context Provider
.
What is a Context Provider?
The Context Provider
is created using createContext().Provider
. Its purpose is to wrap the components that need to access the context data, providing those data through the value
property.
Basic Example
To illustrate the usage of a Context Provider
, let's look at a simple example:
jsx
In this example, UserContext.Provider
wraps UserProfile
and passes the user
object as the context value.
value
Property
The Provider
's value
property is used to pass the data we want to share with child components. This property can accept any type of data, including objects, functions, arrays, and primitives.
Example with Primitive Values
jsx
In this example, the value passed by the Provider
is a primitive number.
Nested Providers
In complex applications, it may be necessary to use multiple contexts. A common pattern is nesting multiple Providers
.
jsx
Here, Page
consumes data from both UserContext
and ThemeContext
.
[Placeholder: Image showing a component tree with multiple nested Providers
and how each Provider
encapsulates a subset of the tree]
Best Practices
- Encapsulation of Context: Keep the usage of the
Provider
as encapsulated as possible. Avoid wrapping the entire application if it is not necessary. - Avoid Unnecessary Re-renders: Every time the
Provider
's value changes, all consumers will re-render. Use memos (useMemo
) and update functions to minimize these changes. - Multiple Contexts: Do not hesitate to use multiple contexts to separate concerns and avoid large contexts.
Example with useMemo
jsx
In this example, useMemo
is used to memoize the context value and avoid unnecessary re-renders.
Conclusion
The Context Provider
is an essential tool for sharing data through the component tree in a React application. Understanding how and when to use it effectively can improve the efficiency and maintainability of the code. In the next chapter, we will explore how to consume context data using the Context Consumer
.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
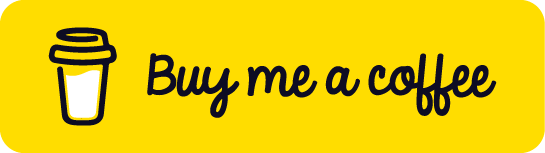
Chat with Chuck
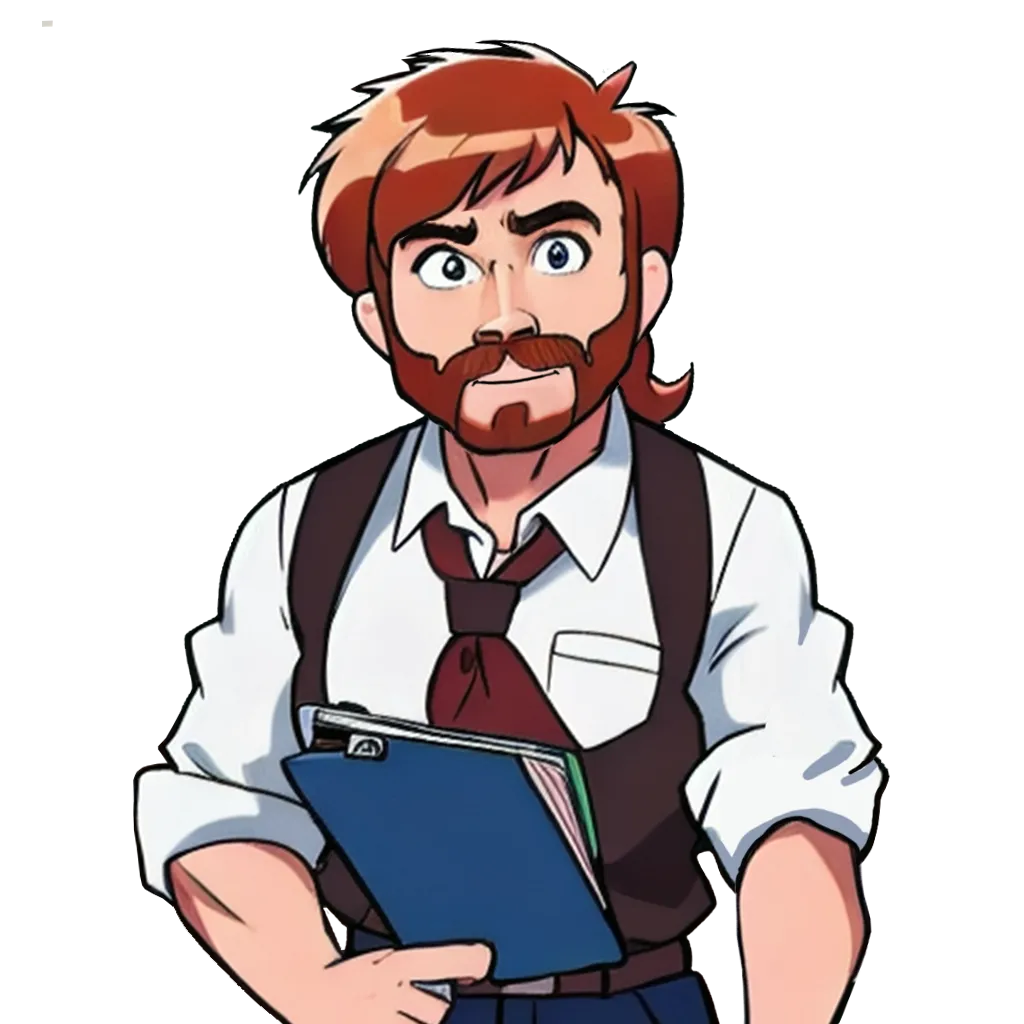
- Introduction to the React Context API
- Fundamentals of State in React
- Creating a Context in React
- Context Provider
- Context Consumer (Context Consumer)
- Using Context with the `useContext` Hook
- Context API vs Prop Drilling
- Context Update
- Nested Contexts
- Context API and Performance
- Advanced Patterns with Context API
- Context API and Functional Components
- Context API and Class Components
- Testing con Context API
- Conclusion and Best Practices