React Context API
Conclusion and Best Practices
Throughout this course, we have explored in-depth the use of React's Context API, covering everything from basic concepts to advanced patterns. As we wrap up, it’s important to synthesize the lessons learned and highlight some best practices for its effective use in React applications.
Summary of the Context API
React's Context API is a powerful tool for managing global states and sharing data between components without the need for prop drilling. It provides a secure and efficient way to pass data through the component tree, allowing for greater flexibility and simplicity in state management.
Main Advantages
- Eliminates Prop Drilling: Facilitates handling data that needs to be accessible at multiple levels in the component tree.
- Improves Code Clarity: Makes the code cleaner and easier to maintain.
- Flexible and Extensible: Allows for the creation of reusable and modulable contexts.
Best Practices for Using the Context API
-
State Encapsulation: Keep the state logic and context encapsulated within specific components. Use providers (
Provider
) and consumers (Consumer
) correctly to avoid data leaks and ensure state isolation. -
Split Context: Do not overload a single context with too much data or state logic. Instead, split the state into several smaller, purpose-specific contexts.
-
Use of Memoization: Use
useMemo
anduseCallback
to memoize values and functions passed as context values. This helps prevent unnecessary re-renders and improves performance.jsx -
Advanced Patterns with
useReducer
: To handle complex states with multiple actions, combine the Context API withuseReducer
. This allows for more predictable and scalable state management.jsx -
Effective Testing: When writing tests for components that use the Context API, make sure to wrap the components in context providers and mock contexts as necessary. This ensures that the components are tested in a controlled and reproducible environment.
jsx -
Context Composition: When multiple contexts are needed, consider using higher-order components (HOCs) or Render Props patterns to abstract and reuse context logic.
jsx
Conclusion
The React Context API offers a powerful and flexible solution for managing states and global data in our applications. From eliminating prop drilling to handling complex states with useReducer
, this tool can transform the way we structure and maintain our applications.
Final Tips
- Evaluate Case by Case: Do not use Context API for all shared data. In some cases, prop drilling might still be the simplest and most efficient solution. Evaluate each situation individually.
- Keep It Simple: While it's easy to get carried away with complexity, always look for the simplest and most straightforward solution to the problem.
- Learn and Practice: The best way to master the Context API is through constant practice. Experiment with different patterns and techniques to find the ones that best suit your needs.
Thank you for following this course on the Context API in React. We hope you found the information useful and can now apply these insights to build more efficient and maintainable applications.
- Introduction to the React Context API
- Fundamentals of State in React
- Creating a Context in React
- Context Provider
- Context Consumer (Context Consumer)
- Using Context with the `useContext` Hook
- Context API vs Prop Drilling
- Context Update
- Nested Contexts
- Context API and Performance
- Advanced Patterns with Context API
- Context API and Functional Components
- Context API and Class Components
- Testing con Context API
- Conclusion and Best Practices
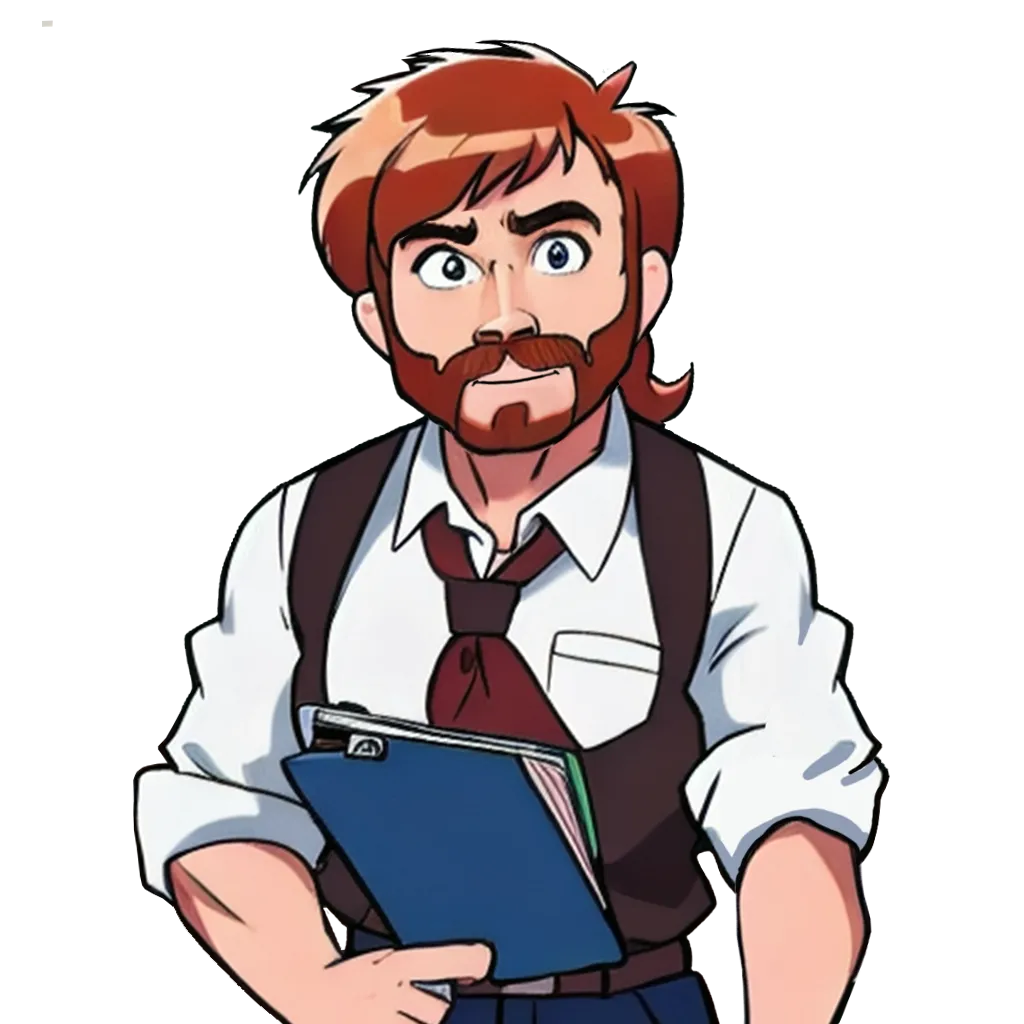