React Context API
Nested Contexts
In large and complex React applications, it can be beneficial to use multiple contexts to manage different parts of the global state. Nested contexts allow for better organization and separation of data, but they can also introduce additional complexities. This chapter will explore how to work with nested contexts efficiently.
What are Nested Contexts?
Nested contexts involve wrapping a component in multiple Providers
, each providing a different context. This allows different parts of the global state to be available at various levels of the component tree.
Advantages of Using Nested Contexts
- Separation of Concerns: Allows managing different aspects of the state separately, improving code clarity and maintainability.
- Performance Optimization: Minimizes the risk of unnecessary re-renders by isolating changes to specific parts of the state.
- Reusability: Facilitates the reuse of components in different parts of the application with different contexts.
Example of Nested Contexts
Let's look at a simple example of how to nest contexts.
jsx
In this example, UserContext.Provider
and ThemeContext.Provider
are nested to provide their values to Page
and its child components.
Consuming Multiple Contexts in a Component
When a component needs to consume multiple contexts, useContext
can be called multiple times.
Example
jsx
In this example, UserAndTheme
consumes both UserContext
and ThemeContext
to render their data.
Performance Considerations
- Memoization: Use
useMemo
anduseCallback
to memoize context values and update functions, minimizing unnecessary renders. - State Fragmentation: Do not combine too much data into a single context. Split the state into multiple contexts as needed.
- Composition of Contexts: Leverage Higher Order Components (HOCs) and other composition patterns to organize and combine multiple contexts more effectively.
Advanced Example with Memoization
jsx
In this example, useMemo
is used to memoize the context values, improving the application's performance.
Conclusion
Nested contexts offer a flexible and organized way to manage multiple parts of the global state in a React application. By understanding how to nest and consume contexts effectively, you can improve both the code clarity and the performance of your application. In the next chapter, we will discuss how the Context API affects and enhances your application's performance.
- Introduction to the React Context API
- Fundamentals of State in React
- Creating a Context in React
- Context Provider
- Context Consumer (Context Consumer)
- Using Context with the `useContext` Hook
- Context API vs Prop Drilling
- Context Update
- Nested Contexts
- Context API and Performance
- Advanced Patterns with Context API
- Context API and Functional Components
- Context API and Class Components
- Testing con Context API
- Conclusion and Best Practices
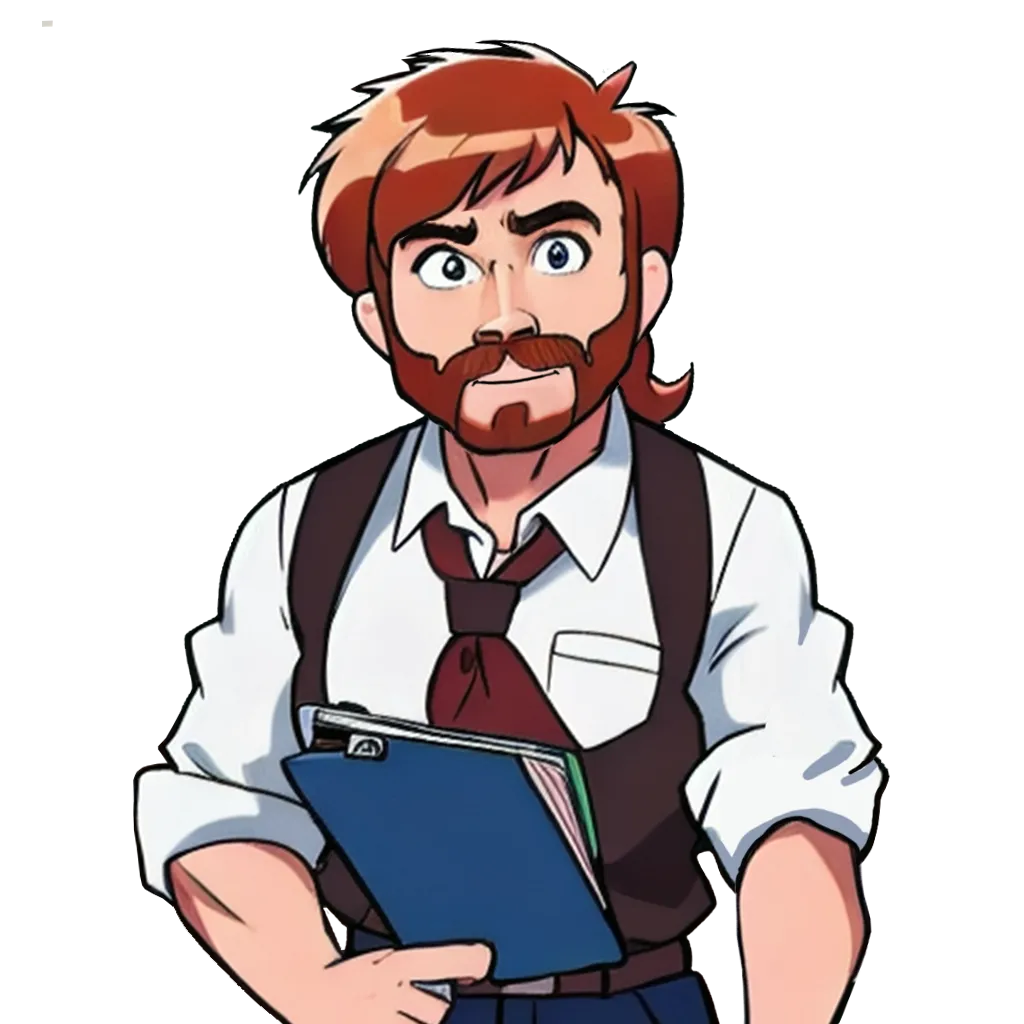